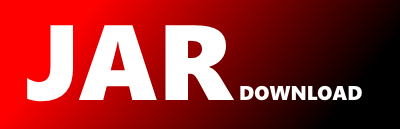
org.fastnate.generator.context.EmbeddedProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastnate-generator Show documentation
Show all versions of fastnate-generator Show documentation
The Fastnate Offline SQL Generator
The newest version!
package org.fastnate.generator.context;
import java.io.IOException;
import java.lang.reflect.Constructor;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Modifier;
import java.util.Collection;
import java.util.HashSet;
import java.util.Map;
import java.util.Set;
import java.util.TreeMap;
import javax.annotation.Nonnull;
import javax.persistence.Access;
import javax.persistence.AssociationOverride;
import javax.persistence.AttributeOverride;
import javax.persistence.Column;
import javax.persistence.Embeddable;
import javax.persistence.EmbeddedId;
import org.fastnate.generator.statements.StatementsWriter;
import org.fastnate.generator.statements.TableStatement;
import lombok.AccessLevel;
import lombok.Getter;
/**
* Handling of properties defined in {@link Embeddable}s.
*
* @author Tobias Liefke
*
* @param
* The type of the container class (the entity)
* @param
* The type of the embeddable object
*/
@Getter
public class EmbeddedProperty extends Property {
/**
* The properties within the embedded object.
*/
private final Map> embeddedProperties = new TreeMap<>();
private final boolean id;
@Nonnull
@Getter(AccessLevel.NONE)
private final Constructor constructor;
/**
* Instantiates a new embedded property.
*
* @param entityClass
* the class of the entity
* @param attribute
* the attribute that contains the embedded object
*/
public EmbeddedProperty(final EntityClass> entityClass, final AttributeAccessor attribute) {
super(attribute);
this.id = attribute.isAnnotationPresent(EmbeddedId.class);
final Class type = (Class) attribute.getType();
if (!type.isAnnotationPresent(Embeddable.class)) {
throw new IllegalArgumentException(attribute + " does reference " + type + " which is not embeddable.");
}
try {
// Try to find the noargs constructor
this.constructor = type.getDeclaredConstructor();
if (!Modifier.isPublic(this.constructor.getModifiers())) {
this.constructor.setAccessible(true);
}
} catch (final NoSuchMethodException e) {
throw new ModelException("Could not find constructor without arguments for " + type);
}
// Determine the access style
final AccessStyle accessStyle;
final Access accessType = type.getAnnotation(Access.class);
if (accessType != null) {
accessStyle = AccessStyle.getStyle(accessType.value());
} else {
accessStyle = attribute.getAccessStyle();
}
final Map attributeOverrides = EntityClass
.getAttributeOverrides(attribute.getElement());
final Map accociationOverrides = EntityClass
.getAccociationOverrides(attribute.getElement());
for (final AttributeAccessor field : accessStyle.getDeclaredAttributes((Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy