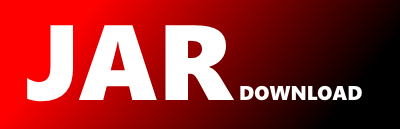
org.fastnate.generator.context.PrimitiveProperty Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastnate-generator Show documentation
Show all versions of fastnate-generator Show documentation
The Fastnate Offline SQL Generator
The newest version!
package org.fastnate.generator.context;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.util.Calendar;
import java.util.Date;
import javax.persistence.Basic;
import javax.persistence.Column;
import javax.validation.constraints.NotNull;
import org.fastnate.generator.DefaultValue;
import org.fastnate.generator.converter.ValueConverter;
import org.fastnate.generator.dialect.GeneratorDialect;
import org.fastnate.generator.statements.ColumnExpression;
import org.fastnate.generator.statements.PrimitiveColumnExpression;
import org.fastnate.generator.statements.TableStatement;
import lombok.Getter;
/**
* Describes a singular primitive property of an {@link EntityClass}.
*
* A primitive property is not only a Java primitive, but it includes all properties that are of a type that is easily
* mapped to an SQL expression using a {@link ValueConverter}.
*
* This includes:
*
* - Java primitive types (boolean, char, byte, short, int, long, float, double)
* - Their wrapper types ({@link Boolean}, {@link Character}, {@link Byte}, {@link Short}, {@link Integer},
* {@link Long}, {@link Float}, {@link Double})
* - {@link String}
* - {@link BigInteger} and {@link BigDecimal}
* - {@link Date} and its subclasses ({@link java.sql.Date}, {@link java.sql.Time} and {@link java.sql.Timestamp})
*
* - {@link Calendar}
* - byte arrays ({@code byte[]} and {@code Byte[]})
* - character array ({@code char[]} and {@code Character[]})
* - Enumerated types ({@link Enum})
* - User-defined serializable types
*
*
* @param
* The type of the container class
* @param
* The type of the primitive
* @author Tobias Liefke
* @author Andreas Penski
*/
@Getter
public class PrimitiveProperty extends SingularProperty {
private static boolean isRequired(final AttributeAccessor attribute) {
final Basic basic = attribute.getAnnotation(Basic.class);
return basic != null && !basic.optional() || attribute.isAnnotationPresent(NotNull.class)
|| attribute.getType().isPrimitive();
}
/** The current context. */
private final GeneratorContext context;
/** The table of the column. */
private final GeneratorTable table;
/** The column name in the table. */
private final GeneratorColumn column;
/** Indicates if the property required. */
private final boolean required;
/** The converter for any value. */
private final ValueConverter converter;
/** The default value. */
private final String defaultValue;
/**
* Instantiates a new primitive property.
*
* @param context
* the current context
* @param table
* the table that the column belongs to
* @param attribute
* the attribute of the property
* @param columnMetadata
* the column metadata
*/
public PrimitiveProperty(final GeneratorContext context, final GeneratorTable table,
final AttributeAccessor attribute, final Column columnMetadata) {
this(context, table, attribute, columnMetadata, false);
}
/**
* Instantiates a new primitive property.
*
* @param context
* the current context
* @param table
* the table that the column belongs to
* @param attribute
* the attribute of the property
* @param columnMetadata
* the column metadata
* @param autogenerated
* {@code true} if the values of this property are not part of any insert statement because they are
* generated by the database
*/
public PrimitiveProperty(final GeneratorContext context, final GeneratorTable table,
final AttributeAccessor attribute, final Column columnMetadata, final boolean autogenerated) {
super(attribute);
this.context = context;
this.table = table;
this.column = table
.resolveColumn(columnMetadata == null || columnMetadata.name().length() == 0 ? attribute.getName()
: columnMetadata.name(), autogenerated);
this.required = columnMetadata != null && !columnMetadata.nullable() || isRequired(attribute);
this.converter = context.getProvider().createConverter(attribute, (Class) attribute.getType(), false);
this.defaultValue = getDefaultValue(attribute);
}
@Override
public void addInsertExpression(final TableStatement statement, final E entity) {
final T value = getValue(entity);
if (value != null) {
statement.setColumnValue(getColumn(), this.converter.getExpression(value, this.context));
} else if (this.defaultValue != null) {
statement.setColumnValue(getColumn(), this.converter.getExpression(this.defaultValue, this.context));
} else {
failIfRequired(entity);
if (this.context.isWriteNullValues()) {
statement.setColumnValue(this.column, PrimitiveColumnExpression.NULL);
}
}
}
/**
* Finds the default value from the given attribute.
*
* @param attribute
* the current attribute of the property
* @return the default value or {@code null} if none is set
*
* @see DefaultValue#value()
*/
protected String getDefaultValue(final AttributeAccessor attribute) {
final DefaultValue defaultValueAnnotation = attribute.getAnnotation(DefaultValue.class);
if (defaultValueAnnotation != null) {
return defaultValueAnnotation.value();
}
return null;
}
/**
* Resolves the current dialect from the context.
*
* @return the dialect of the current generation context
*/
protected GeneratorDialect getDialect() {
return getContext().getDialect();
}
@Override
public ColumnExpression getExpression(final E entity, final boolean whereExpression) {
final T value = getValue(entity);
if (value == null) {
if (this.defaultValue != null) {
return this.converter.getExpression(this.defaultValue, this.context);
}
return PrimitiveColumnExpression.NULL;
}
return this.converter.getExpression(value, this.context);
}
@Override
public String getPredicate(final E entity) {
final T value = getValue(entity);
if (value == null) {
return this.column + " IS NULL";
}
return this.column + " = " + this.converter.getExpression(value, this.context);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy