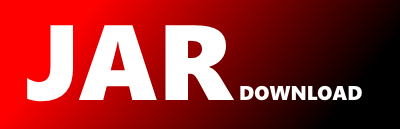
org.fastnate.generator.provider.JpaProvider Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of fastnate-generator Show documentation
Show all versions of fastnate-generator Show documentation
The Fastnate Offline SQL Generator
The newest version!
package org.fastnate.generator.provider;
import java.io.Serializable;
import java.util.Calendar;
import java.util.Date;
import java.util.Properties;
import javax.persistence.AttributeConverter;
import javax.persistence.Convert;
import javax.persistence.Lob;
import javax.persistence.SequenceGenerator;
import javax.persistence.TableGenerator;
import javax.persistence.TemporalType;
import org.apache.commons.lang.ClassUtils;
import org.fastnate.generator.context.AttributeAccessor;
import org.fastnate.generator.context.PrimitiveProperty;
import org.fastnate.generator.converter.BooleanConverter;
import org.fastnate.generator.converter.CalendarConverter;
import org.fastnate.generator.converter.CharConverter;
import org.fastnate.generator.converter.CustomValueConverter;
import org.fastnate.generator.converter.DateConverter;
import org.fastnate.generator.converter.EnumConverter;
import org.fastnate.generator.converter.LobConverter;
import org.fastnate.generator.converter.NumberConverter;
import org.fastnate.generator.converter.SerializableConverter;
import org.fastnate.generator.converter.StringConverter;
import org.fastnate.generator.converter.UnsupportedTypeConverter;
import org.fastnate.generator.converter.ValueConverter;
import org.fastnate.util.ClassUtil;
/**
* Encapsulates details specific to the current JPA implementation.
*
* @author Tobias Liefke
*/
public interface JpaProvider {
/**
* Creates a converter for a primitive database type.
*
* @param
* the generic type
* @param attributeName
* the name of the attribute that contains the value - only to print that name in case of any error
* @param targetType
* the primitive database type
* @return the converter or an instance of {@link UnsupportedTypeConverter} if no converter is available
*/
default ValueConverter createBasicConverter(final String attributeName, final Class targetType) {
if (String.class == targetType) {
return (ValueConverter) new StringConverter();
}
if (byte[].class == targetType || char[].class == targetType) {
return (ValueConverter) new LobConverter();
}
if (Date.class.isAssignableFrom(targetType)) {
return (ValueConverter) new DateConverter(TemporalType.TIMESTAMP);
}
if (Calendar.class.isAssignableFrom(targetType)) {
return (ValueConverter) new CalendarConverter(TemporalType.TIMESTAMP);
}
final Class type = ClassUtils.primitiveToWrapper(targetType);
if (Character.class == type) {
return (ValueConverter) new CharConverter();
}
if (Boolean.class == type) {
return (ValueConverter) new BooleanConverter();
}
if (Number.class.isAssignableFrom(type)) {
return (ValueConverter) new NumberConverter((Class) type);
}
return createFallbackConverter(attributeName, type);
}
/**
* Creates a converter for an attribute that has a {@link PrimitiveProperty}.
*
* @param
* the generic binding of the type of the value
* @param attribute
* the accessor for the attribute that contains the value, not nessecarily of the target type
* @param targetType
* the type of the value
* @param mapKey
* indicates that the converter is for the key of a map
* @return the converter or an instance of {@link UnsupportedTypeConverter} if no converter is available
*/
default ValueConverter createConverter(final AttributeAccessor attribute, final Class targetType,
final boolean mapKey) {
final Convert convert = attribute.getAnnotation(Convert.class);
if (convert != null && !convert.disableConversion()) {
final Class> converterClass = convert.converter();
final Class
© 2015 - 2025 Weber Informatics LLC | Privacy Policy