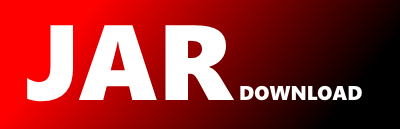
org.fbk.cit.hlt.thewikimachine.index.PageNavigationTemplateSearcher Maven / Gradle / Ivy
/*
* Copyright (2013) Fondazione Bruno Kessler (http://www.fbk.eu/)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.fbk.cit.hlt.thewikimachine.index;
import org.apache.commons.cli.*;
import org.apache.log4j.Logger;
import org.apache.log4j.PropertyConfigurator;
import org.fbk.cit.hlt.thewikimachine.index.util.SetSearcher;
import org.fbk.cit.hlt.thewikimachine.xmldump.AbstractWikipediaExtractor;
import java.io.BufferedReader;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStreamReader;
import java.util.Properties;
/**
* Created with IntelliJ IDEA.
* User: aprosio
* Date: 1/24/13
* Time: 11:37 PM
* To change this template use File | Settings | File Templates.
*/
public class PageNavigationTemplateSearcher extends MappedSetSearcher {
/**
* Define a static logger variable so that it references the
* Logger instance named PageNavigationTemplateSearcher
.
*/
static Logger logger = Logger.getLogger(PageNavigationTemplateSearcher.class.getName());
public PageNavigationTemplateSearcher(String indexName) throws IOException {
super(indexName, PageNavigationTemplateIndexer.PAGE_FIELD_NAME, PageNavigationTemplateIndexer.NAVIGATION_TEMPLATE_FIELD_NAME);
logger.trace(toString(10));
}
@Override
public void interactive() throws Exception {
InputStreamReader reader = null;
BufferedReader myInput = null;
while (true) {
System.out.println("\nPlease write a key and type to continue (CTRL C to exit):");
reader = new InputStreamReader(System.in);
myInput = new BufferedReader(reader);
String query = myInput.readLine().toString();
String[] s = tabPattern.split(query);
if (s.length == 1) {
long begin = System.nanoTime();
String[] result = search(s[0]);
long end = System.nanoTime();
if (result != null && result.length > 0) {
for (int i = 0; i < result.length; i++) {
logger.info(i + "\t" + result[i]);
if (mappings != null) {
logger.info("Topic: " + mappings.getProperty(result[i]));
}
}
logger.info(s[0] + " found in " + tf.format(end - begin) + " ns");
}
else {
logger.info(s[0] + " not found in " + tf.format(end - begin) + " ns");
}
}
}
}
public static void main(String args[]) throws Exception {
String logConfig = System.getProperty("log-config");
if (logConfig == null) {
logConfig = "configuration/log-config.txt";
}
PropertyConfigurator.configure(logConfig);
Options options = new Options();
CommandLine line = null;
try {
Option indexNameOpt = OptionBuilder.withArgName("index").hasArg().withDescription("open an index with the specified name").isRequired().withLongOpt("index").create("i");
Option interactiveModeOpt = OptionBuilder.withArgName("interactive-mode").withDescription("enter in the interactive mode").withLongOpt("interactive-mode").create("t");
Option searchOpt = OptionBuilder.withArgName("search").hasArg().withDescription("search for the specified key").withLongOpt("search").create("s");
Option notificationPointOpt = OptionBuilder.withArgName("int").hasArg().withDescription("receive notification every n pages (default is " + AbstractWikipediaExtractor.DEFAULT_NOTIFICATION_POINT + ")").withLongOpt("notification-point").create("b");
options.addOption(OptionBuilder.withArgName("file").hasArg().withDescription("portal-topic mapping file").withLongOpt("mapping-file").create("m"));
options.addOption("h", "help", false, "print this message");
options.addOption("v", "version", false, "output version information and exit");
options.addOption(indexNameOpt);
options.addOption(interactiveModeOpt);
options.addOption(searchOpt);
options.addOption(notificationPointOpt);
CommandLineParser parser = new PosixParser();
line = parser.parse(options, args);
if (line.hasOption("help") || line.hasOption("version")) {
throw new ParseException("");
}
} catch (ParseException e) {
// oops, something went wrong
if (e.getMessage().length() > 0) {
System.out.println("Parsing failed: " + e.getMessage() + "\n");
}
HelpFormatter formatter = new HelpFormatter();
formatter.printHelp(400, "java -cp dist/thewikimachine.jar org.fbk.cit.hlt.thewikimachine.index.PageNavigationTemplateSearcher", "\n", options, "\n", true);
System.exit(1);
}
int notificationPoint = AbstractWikipediaExtractor.DEFAULT_NOTIFICATION_POINT;
if (line.hasOption("notification-point")) {
notificationPoint = Integer.parseInt(line.getOptionValue("notification-point"));
}
PageNavigationTemplateSearcher pageNavigationTemplateSearcher = new PageNavigationTemplateSearcher(line.getOptionValue("index"));
if (line.hasOption("m")) {
Properties p = new Properties();
p.load(new InputStreamReader(new FileInputStream(line.getOptionValue("m")), "UTF-8"));
pageNavigationTemplateSearcher.setMappings(p);
}
pageNavigationTemplateSearcher.setNotificationPoint(notificationPoint);
if (line.hasOption("search")) {
logger.debug("searching " + line.getOptionValue("search") + "...");
String[] result = pageNavigationTemplateSearcher.search(line.getOptionValue("search"));
logger.info(result);
}
if (line.hasOption("interactive-mode")) {
pageNavigationTemplateSearcher.interactive();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy