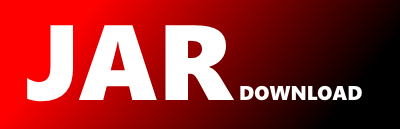
org.fcrepo.client.test.ScalabilityTests Maven / Gradle / Ivy
/* The contents of this file are subject to the license and copyright terms
* detailed in the license directory at the root of the source tree (also
* available online at http://fedora-commons.org/license/).
*/
package org.fcrepo.client.test;
import java.io.File;
import java.io.PrintStream;
import java.util.ArrayList;
import java.util.concurrent.Callable;
import java.util.concurrent.ExecutorService;
import java.util.concurrent.Executors;
import org.fcrepo.client.FedoraClient;
import org.fcrepo.common.Constants;
import org.fcrepo.server.management.FedoraAPIMMTOM;
import org.fcrepo.server.utilities.TypeUtility;
/**
* Performs test to determine the maximum number of objects
* which can be stored in a Fedora repository.
*
* @author Bill Branan
*/
public class ScalabilityTests
implements Constants {
private FedoraAPIMMTOM apim;
private PrintStream out;
private static String DEMO_FOXML_TEXT;
private static byte[] DEMO_FOXML_BYTES;
private static final Boolean TRUE = new Boolean(true);
private long totalIngested = 0;
private static final int defaultBatchSize = 10;
private static final int defaultNumBatches = 1;
private static final int defaultNumThreads = 1;
private int batchSize = 1;
private int numBatches = 1;
private int numThreads = 1;
private ExecutorService threadPool = null;
private ArrayList> ingestRunnerList = null;
static {
// Test FOXML object
StringBuilder sb = new StringBuilder();
sb.append("");
sb.append("");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" Datastream Content ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" PHhtbD5EYXRhc3RyZWFtIENvbnRlbnQ8L3htbD4=");
sb.append(" ");
sb.append(" ");
sb.append(" ");
sb.append(" ");
DEMO_FOXML_TEXT = sb.toString();
DEMO_FOXML_BYTES = DEMO_FOXML_TEXT.getBytes();
}
public void init(String host, String port, String username, String password, String batch, String batches, String threads, String outputFileLocation, String context) throws Exception {
String baseURL = "http://" + host + ":" + port + "/" + context;
FedoraClient fedoraClient = new FedoraClient(baseURL, username, password);
apim = fedoraClient.getAPIMMTOM();
try {
batchSize = Integer.valueOf(batch);
} catch (NumberFormatException nfe) {
System.err.println("Batch Size value could not be " +
"converted to an integer, using the default value (" +
defaultBatchSize + ") instead");
batchSize = defaultBatchSize;
}
try {
numBatches = Integer.valueOf(batches);
} catch (NumberFormatException nfe) {
System.err.println("Number of Batches value could not be " +
"converted to an integer, using the default value (" +
defaultNumBatches + ") instead");
numBatches = defaultNumBatches;
}
try {
numThreads = Integer.valueOf(threads);
} catch (NumberFormatException nfe) {
System.err.println("Number of Threads value could not be " +
"converted to an integer, using the default value (" +
defaultNumThreads + ") instead");
numThreads = defaultNumThreads;
}
File outputFile = new File(outputFileLocation);
out = new PrintStream(outputFile);
out.println("--- Scalability Test Results ---");
out.println("Total Objects Ingested, " +
"Time (ms) To Ingest Batch of " + batchSize + " Objects, " +
"Average Ingest Time (ms) Per Object");
threadPool = Executors.newFixedThreadPool(numThreads);
ingestRunnerList = new ArrayList>();
for(int i=0; i 0) {
for(int i=0; i {
@Override
public Boolean call() throws Exception {
apim.ingest(TypeUtility.convertBytesToDataHandler(DEMO_FOXML_BYTES), FOXML1_1.uri, "Ingest Test");
return TRUE;
}
}
private static void usage() {
System.out.println("Runs a scalability test over a running Fedora repository.");
System.out.println("USAGE: ant scalability-tests " +
"-Dhost=HOST " +
"-Dport=PORT " +
"-Dusername=USERNAME " +
"-Dpassword=PASSWORD " +
"-Dbatchsize=BATCH-SIZE " +
"-Dbatches=NUM-BATCHES " +
"-Dthreads=NUM-THREADS " +
"-Dfile=OUTPUT-FILE " +
"[-Dcontext=CONTEXT]");
System.out.println("Where:");
System.out.println(" HOST = Host on which Fedora server is running.");
System.out.println(" PORT = Port on which the Fedora server APIs can be accessed.");
System.out.println(" USERNAME = A fedora user with administrative privileges.");
System.out.println(" PASSWORD = The fedora user's password.");
System.out.println(" BATCH-SIZE = The size of the ingest batch. Statements are only printed");
System.out.println(" to the output file at the end of each batch.");
System.out.println(" NUM-BATCHES = The number of batches to run. Set to 0 to run indefinitely.");
System.out.println(" NUM-THREADS = The number of threads to use in the thread pool.");
System.out.println(" OUTPUT-FILE = The file to which the test results will be written.");
System.out.println(" If the file does not exist, it will be created, if the");
System.out.println(" file does exist the new results will be appended.");
System.out.println(" CONTEXT = The application server context Fedora is deployed in. This parameter is optional");
System.out.println("Example:");
System.out.println("ant scalability-tests " +
"-Dhost=localhost " +
"-Dport=8080 " +
"-Dusername=fedoraAdmin " +
"-Dpassword=fedoraAdmin " +
"-Dbatchsize=100 " +
"-Dbatches=10 " +
"-Dthreads=5 " +
"-Dfile=C:\\temp\\scalability_testing_output.txt " +
"-Dcontext=my-fedora");
System.exit(1);
}
public static void main(String[] args) throws Exception {
if(args.length < 8 || args.length > 9) {
usage();
}
String host = args[0];
String port = args[1];
String username = args[2];
String password = args[3];
String batchSize = args[4];
String numBatches = args[5];
String threads = args[6];
String output = args[7];
String context = Constants.FEDORA_DEFAULT_APP_CONTEXT;
if (args.length == 9 && !args[8].equals("")){
context = args[8];
}
if(host == null || host.startsWith("$") ||
port == null || port.startsWith("$") ||
username == null || username.startsWith("$") ||
password == null || password.startsWith("$") ||
batchSize == null || batchSize.startsWith("$") ||
numBatches == null || numBatches.startsWith("$") ||
threads == null || threads.startsWith("$") ||
output == null || output.startsWith("$")) {
usage();
}
ScalabilityTests tests = new ScalabilityTests();
tests.init(host, port, username, password, batchSize, numBatches, threads, output, context);
System.out.println("Running Scalability Test...");
tests.runIngestTest();
tests.close();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy