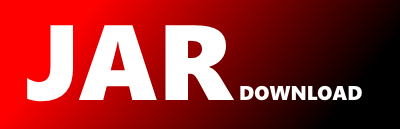
org.modeshape.jcr.api.Property Maven / Gradle / Ivy
Show all versions of modeshape-jcr-api Show documentation
/*
* ModeShape (http://www.modeshape.org)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.modeshape.jcr.api;
import javax.jcr.Node;
import javax.jcr.NodeIterator;
import javax.jcr.RepositoryException;
import javax.jcr.ValueFormatException;
/**
* Extension of {@link javax.jcr.Property} with some custom methods for retrieving values.
*
* @author Horia Chiorean ([email protected])
*/
public interface Property extends javax.jcr.Property {
/**
* Converts the value(s) of the property (if possible) to the specified type.
* The list of supported types for single-valued properties is:
*
* - {@link String}
* - {@link Long}
* - {@link Double}
* - {@link Boolean}
* - {@link java.math.BigDecimal}
* - {@link java.util.Calendar}
* - {@link java.util.Date}
* - {@link org.modeshape.jcr.api.value.DateTime}
* - {@link org.modeshape.jcr.api.Binary}
* - {@link javax.jcr.Binary}
* - {@link java.io.InputStream}
* - {@link javax.jcr.Node}
* - {@link javax.jcr.NodeIterator}
*
* The list of supported types for multi-valued properties is:
*
* - {@link String}{@code []}
* - {@link Long}{@code []}
* - {@link Double}{@code []}
* - {@link Boolean}{@code []}
* - {@link java.math.BigDecimal}{@code []}
* - {@link java.util.Calendar}{@code []}
* - {@link java.util.Date}{@code []}
* - {@link org.modeshape.jcr.api.value.DateTime}{@code []}
* - {@link org.modeshape.jcr.api.Binary}{@code []}
* - {@link javax.jcr.Binary}{@code []}
* - {@link java.io.InputStream}{@code []}
* - {@link javax.jcr.Node}{@code []}
* - {@link javax.jcr.NodeIterator}
*
*
* For single-valued properties, this method attempts to convert the actual value into the specified type.
* For multi-valued properties, this method attempts to convert the actual values into an array of the specified
* type; the only exception to this rule is that in the
* case of multi-valued reference properties a simple {@link javax.jcr.NodeIterator} type is expected:
* {@code getAs(NodeIterator.class)}.
*
*
* For example, the following shows how to obtain the value of a single-valued property as a {@code String} value:
*
* Property prop = ...
* String value = prop.getAs(String.class);
*
* If the property is multi-valued, then the values can be obtained by passing in the desired array type:
*
* String[] values = prop.getAs(String[].class);
*
* This example will always work, since all property values can be converted to a {@code String}.
*
*
* The following attempts to convert all values to {@link java.util.Date} instances:
*
* Date[] values = prop.getAs(Date[].class);
*
*
*
* Finally, a single- or multi-valued property whose value(s) are references can easily be obtained as a
* {@link NodeIterator}, which can represent one or multiple {@link Node} instances:
*
* NodeIterator iter = prop.getAs(NodeIterator.class);
*
*
*
* @param type a {@link Class} representing the type to which to convert the values of the property; may not be {@code null}
* and is expected to be one of the above types.
* @param the type-argument for {@code type}
* @return the value of this property converted to the given type.
* @throws ValueFormatException if the conversion cannot be performed. This can occur for a number of reasons: type
* incompatibility or passing in an array type for a single value property or passing in a non-array type for a multi-valued
* property.
* @throws RepositoryException if anything else unexpected fails when attempting to perform the conversion.
*/
public T getAs(Class type) throws ValueFormatException, RepositoryException;
/**
* Converts the value of the property located at the given index to the specified type. This can be used for single-valued
* properties if the index
property is '0', or multi-valued properties. Array types should not be supplied
* as the first parameter.
*
* For example, this is a property usage of this method to obtain the value of a single-valued property or
* the first value of a multi-valued property as a {@code long}:
*
* Long value = prop.getAs(Long.class, 0)
*
* Likewise, the following usage will only work for multi-valued properties, since only a multi-valued property
* can have 2 or more values:
*
* Long value = prop.getAs(Long.class, 1)
*
*
*
* @param type a {@link Class} representing the type to which to convert the value of the property; may not be {@code null}
* and is expected to be one of the types from {@link Property#getAs(Class)}.
* @param index the position of the property in the array of values.
* @param the type-argument for {@code type}
* @return the value at the position {@code index} converted to the given type.
* @throws ValueFormatException if the conversion cannot be performed or if this method is called for single-valued properties.
* @throws IndexOutOfBoundsException if {@code index} is outside the array of values for the property.
* @throws RepositoryException if anything unexpected fails.
*/
public T getAs(Class type, int index) throws ValueFormatException, IndexOutOfBoundsException, RepositoryException;
}