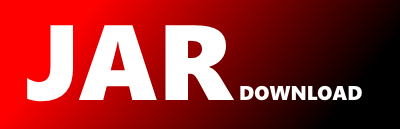
org.fernice.flare.style.context.StyleRootStack.kt Maven / Gradle / Ivy
/*
* This Source Code Form is subject to the terms of the Mozilla Public
* License, v. 2.0. If a copy of the MPL was not distributed with this
* file, You can obtain one at http://mozilla.org/MPL/2.0/.
*/
package org.fernice.flare.style.context
import org.fernice.flare.dom.Element
import org.fernice.flare.style.StyleRoot
import java.util.LinkedList
class StyleRootStack(styleRoot: StyleRoot) : Iterable {
private val layers = LinkedList()
private val styleRoots = LinkedList()
init {
styleRoots.push(styleRoot)
}
fun insert(element: Element) {
if (peek() === element) return
val parent = element.traversalParent
while (!isEmpty() && peek() !== parent) {
pop()
}
if (peek() === parent) {
push(element)
} else {
val reversed = LinkedList()
var current: Element? = element
while (current != null) {
reversed.addFirst(current)
current = current.traversalParent
}
for (entry in reversed) {
push(entry)
}
}
}
private fun push(element: Element) {
val styleRoot = element.styleRoot
layers.push(Layer(element, styleRoot != null))
if (styleRoot != null) {
styleRoots.push(styleRoot)
}
}
private fun pop() {
val layer = layers.pop()
if (layer.hasStyleRoot) {
styleRoots.pop()
}
}
private fun peek(): Element? {
return layers.peek()?.element
}
private fun isEmpty(): Boolean {
return layers.isEmpty()
}
private fun clear() {
repeat(layers.size) {
pop()
}
}
override fun iterator(): Iterator = styleRoots.iterator()
fun reversedIterator(): Iterator {
return object : Iterator {
private val iterator = styleRoots.listIterator(styleRoots.size)
override fun hasNext(): Boolean = iterator.hasPrevious()
override fun next(): StyleRoot = iterator.previous()
}
}
fun asSequence(): Sequence = styleRoots.asSequence()
fun asReversedSequence(): Sequence = Sequence { reversedIterator() }
private class Layer(val element: Element, val hasStyleRoot: Boolean)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy