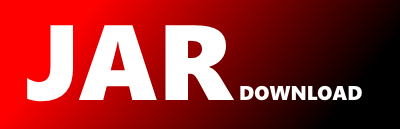
org.ferris.journal.gui.model.Model Maven / Gradle / Ivy
package org.ferris.journal.gui.model;
import java.util.List;
import org.ferris.journal.jws.account.Account;
import org.ferris.journal.jws.journal.Journal;
import org.ferris.journal.jws.journalentry.JournalEntry;
public interface Model
{
/**
* Model will no longer be used, typically this is done
* when the program is exited.
*
* @return
* Reference to this instance.
*/
public Model release();
/**
* Application is performing these operations which the model
* can log, store, etc., and notify it's observers.
*/
public Model performingOperation(String description);
/**
* Store the account
*/
public Model setAccount(Account iAmLoggedIn);
/**
* Get the account logged in, null if not logged
* in or if login failed.
*/
public Account getAccount();
/**
* Get all the journals for the account
*/
public List getAllJournals();
/**
* Get all the active journals for the account
*/
public List getActiveJournals();
/**
* Store list of all journals
*/
public Model setJournals(List allJournals);
/**
* Add Journal to the list
*/
public Model insertJournal(Journal j);
/**
* Update Journal in the list
*/
public Model updateJournal(Journal j);
/**
* User wants to enter data for a new journal
*/
public Model newJournal();
/**
* User wants to edit data of an existing journal
*/
public Model editJournal(Journal editMe);
/**
* User wants to remove data of an existing journal
*/
public Model deleteJournal(Journal deleteMe);
/**
* Created JournalEntry
*/
public Model insertJournalEntry(JournalEntry je);
/**
* Updated JournalEntry
*/
public Model updateJournalEntry(JournalEntry je);
/**
* New JournalEntry
*/
public Model newJournalEntry();
/**
* Delete JournalEntry
*/
public Model deleteJournalEntry(JournalEntry j);
/**
* Journal entries matching search criteria are found.
*/
public Model setJournalEntrySearchResults(List searchResults);
/**
* Edit this journal entry
*/
public Model editJournalEntry(JournalEntry entry);
/////////////////////////////////////////////////////////////////////
//
// observers
//
/////////////////////////////////////////////////////////////////////
/**
* Register ReleaseObserver
*
* @return
* Reference to this instance.
*/
public Model registerObserver(ReleaseObserver observer);
/**
* Remove ReleaseObserver
*
* @return
* Reference to observer removed or null
* if observer not registered
*/
public ReleaseObserver removeObserver(ReleaseObserver observer);
/**
* Register OperationObserver
*
* @return
* Reference to this instance.
*/
public Model registerObserver(OperationObserver observer);
/**
* Remove OperationObserver
*
* @return
* Reference to observer removed or null
* if observer not registered
*/
public OperationObserver removeObserver(OperationObserver observer);
/**
* Register JournalObserver
*
* @return
* Reference to this instance.
*/
public Model registerObserver(JournalObserver observer);
/**
* Remove JournalObserver
*
* @return
* Reference to observer removed or null
* if observer not registered
*/
public JournalObserver removeObserver(JournalObserver observer);
/**
* Register JournalEntryObserver
*
* @return
* Reference to this instance.
*/
public Model registerObserver(JournalEntryObserver observer);
/**
* Remove JournalEntryObserver
*
* @return
* Reference to observer removed or null
* if observer not registered
*/
public JournalEntryObserver removeObserver(JournalEntryObserver observer);
/**
* Register JournalEntrySearchObserver
*
* @return
* Reference to this instance.
*/
public Model registerObserver(JournalEntrySearchObserver observer);
/**
* Remove JournalEntrySearchObserver
*
* @return
* Reference to observer removed or null
* if observer not registered
*/
public JournalEntrySearchObserver removeObserver(JournalEntrySearchObserver observer);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy