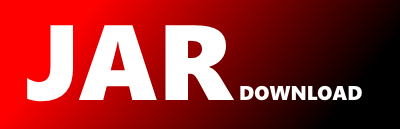
org.ferris.journal.gui.view.frame.KJournalsFrame Maven / Gradle / Ivy
package org.ferris.journal.gui.view.frame;
import static org.ferris.journal.gui.i18n.i18n.getString;
import java.awt.Dimension;
import java.awt.FlowLayout;
import java.awt.GridBagConstraints;
import java.awt.GridBagLayout;
import java.awt.Insets;
import java.awt.MouseInfo;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.ItemEvent;
import java.awt.event.ItemListener;
import java.awt.event.WindowEvent;
import java.awt.event.WindowFocusListener;
import java.util.ArrayList;
import java.util.List;
import javax.swing.BorderFactory;
import javax.swing.JButton;
import javax.swing.JCheckBox;
import javax.swing.JFrame;
import javax.swing.JLabel;
import javax.swing.JOptionPane;
import javax.swing.JPanel;
import javax.swing.JScrollPane;
import javax.swing.JSplitPane;
import javax.swing.border.BevelBorder;
import javax.swing.border.EtchedBorder;
import javax.swing.event.DocumentEvent;
import javax.swing.event.DocumentListener;
import javax.swing.event.ListSelectionEvent;
import javax.swing.event.ListSelectionListener;
import org.apache.log4j.Logger;
import org.ferris.journal.gui.controller.JournalsController;
import org.ferris.journal.gui.model.JournalObserver;
import org.ferris.journal.gui.model.Model;
import org.ferris.journal.gui.model.ModelForVisualEditor;
import org.ferris.journal.gui.model.OperationObserver;
import org.ferris.journal.gui.model.ReleaseObserver;
import org.ferris.journal.gui.view.button.KCloseButton;
import org.ferris.journal.gui.view.button.KDeleteButton;
import org.ferris.journal.gui.view.button.KNewButton;
import org.ferris.journal.gui.view.button.KSaveButton;
import org.ferris.journal.gui.view.dialog.KErrorDialog;
import org.ferris.journal.gui.view.list.KJournalList;
import org.ferris.journal.gui.view.list.KJournalListModelForAllJournals;
import org.ferris.journal.gui.view.textfield.KJournalNameTextField;
import org.ferris.journal.jws.journal.Journal;
import org.ferris.swing.InvokeAndWait;
import org.ferris.swing.ui.ThrowableDialog;
public
class
KJournalsFrame
extends
KDefaultFrame
implements
ActionListener
, ReleaseObserver
, OperationObserver
, JournalObserver
, WindowFocusListener
, ListSelectionListener
, DocumentListener
, ItemListener
{
private static final long serialVersionUID = 8986611052518547576L;
private final Logger log = Logger.getLogger(getClass()); // @jve:decl-index=0:
private Model model; // @jve:decl-index=0:
private JournalsController controller; // @jve:decl-index=0:
private Journal editing; // @jve:decl-index=0:
private JPanel jContentPane = null;
private JPanel mainPanel = null;
private JButton newButton = null;
private JPanel buttonPanel = null;
private JButton saveButton = null;
private JButton closeButton = null;
private JPanel statusPanel = null;
private JLabel statusLabel = null;
private JScrollPane journalsScrollPane = null;
private KJournalList journalsList = null;
private JPanel inputPanel = null;
private JLabel nameLabel = null;
private KJournalNameTextField nameText = null;
private JLabel activeLabel = null;
private JCheckBox activeCheckBox = null;
private JLabel spacer = null;
private JSplitPane mainSplitPane = null;
private JButton deleteButton = null;
/**
* This method initializes and is only here
* because the eclipse visual editor needs it.
*/
public KJournalsFrame() {
super();
initialize();
}
/**
* This method initializes
*/
public KJournalsFrame(JournalsController controller, Model model) {
super();
initialize(controller, model);
}
/**
* This method initializes this
*/
public void initialize(JournalsController controller, Model model) {
this.controller = controller;
this.model = model;
initialize();
registerObservers();
}
/**
* Cleanup resources the frame is using.
*/
public void dispose() {
try {
removeObservers();
} catch (Throwable ignore) {}
super.dispose();
}
/**
* This method initializes this
*/
private void initialize()
{
this.setSize(new Dimension(432, 286));
this.setContentPane(getJContentPane());
this.setDefaultCloseOperation(JFrame.DISPOSE_ON_CLOSE);
setLocationRelativeTo(null);
setTitle(getString("journal.frame.title")); // @jve
addWindowFocusListener(this);
}
/**
* This method initializes jContentPane
*
* @return javax.swing.JPanel
*/
public JPanel getJContentPane() {
if (jContentPane == null) {
GridBagConstraints gridBagConstraints21 = new GridBagConstraints();
gridBagConstraints21.gridx = 1;
gridBagConstraints21.fill = GridBagConstraints.HORIZONTAL;
gridBagConstraints21.gridwidth = 1;
gridBagConstraints21.weighty = 0.0;
gridBagConstraints21.anchor = GridBagConstraints.SOUTH;
gridBagConstraints21.gridy = 4;
GridBagConstraints gridBagConstraints11 = new GridBagConstraints();
gridBagConstraints11.gridx = 0;
gridBagConstraints11.fill = GridBagConstraints.HORIZONTAL;
gridBagConstraints11.insets = new Insets(0, 10, 0, 10);
gridBagConstraints11.gridy = 2;
GridBagConstraints gridBagConstraints2 = new GridBagConstraints();
gridBagConstraints2.gridx = 1;
gridBagConstraints2.fill = GridBagConstraints.HORIZONTAL;
gridBagConstraints2.insets = new Insets(10, 10, 10, 10);
gridBagConstraints2.gridy = 3;
GridBagConstraints gridBagConstraints = new GridBagConstraints();
gridBagConstraints.gridx = 1;
gridBagConstraints.fill = GridBagConstraints.BOTH;
gridBagConstraints.anchor = GridBagConstraints.NORTH;
gridBagConstraints.weighty = 1.0;
gridBagConstraints.weightx = 1.0;
gridBagConstraints.insets = new Insets(10, 10, 0, 10);
gridBagConstraints.gridy = 1;
jContentPane = new JPanel();
jContentPane.setLayout(new GridBagLayout());
jContentPane.add(getMainPanel(), gridBagConstraints);
jContentPane.add(getButtonPanel(), gridBagConstraints2);
jContentPane.add(getStatusPanel(), gridBagConstraints21);
}
return jContentPane;
}
/**
* This method initializes statusLabel
*
* @return javax.swing.JLabel
*/
private JLabel getStatusLabel() {
if (statusLabel == null) {
statusLabel = new JLabel(" ");
}
return statusLabel;
}
/**
* This method initializes mainPanel
*
* @return javax.swing.JPanel
*/
private JPanel getMainPanel() {
if (mainPanel == null) {
GridBagConstraints gridBagConstraints4 = new GridBagConstraints();
gridBagConstraints4.fill = GridBagConstraints.BOTH;
gridBagConstraints4.gridy = 0;
gridBagConstraints4.weightx = 1.0;
gridBagConstraints4.weighty = 1.0;
gridBagConstraints4.gridx = 0;
GridBagConstraints gridBagConstraints1 = new GridBagConstraints();
gridBagConstraints1.fill = GridBagConstraints.NONE;
gridBagConstraints1.weighty = 0.0;
gridBagConstraints1.gridx = 0;
gridBagConstraints1.gridy = 0;
gridBagConstraints1.anchor = GridBagConstraints.WEST;
gridBagConstraints1.weightx = 0.0;
mainPanel = new JPanel();
mainPanel.setLayout(new GridBagLayout());
mainPanel.setBorder(BorderFactory.createEtchedBorder(EtchedBorder.LOWERED));
mainPanel.add(getMainSplitPane(), gridBagConstraints4);
}
return mainPanel;
}
/**
* This method initializes loginButton
*
* @return javax.swing.JButton
*/
private JButton getNewButton() {
if (newButton == null) {
newButton = new KNewButton();
newButton.addActionListener(this);
}
return newButton;
}
/**
* This method initializes buttonPanel
*
* @return javax.swing.JPanel
*/
private JPanel getButtonPanel() {
if (buttonPanel == null) {
GridBagConstraints gridBagConstraints3 = new GridBagConstraints();
gridBagConstraints3.anchor = GridBagConstraints.EAST;
gridBagConstraints3.gridx = -1;
gridBagConstraints3.gridy = -1;
gridBagConstraints3.insets = new Insets(8, 5, 8, 5);
buttonPanel = new JPanel();
buttonPanel.setLayout(new FlowLayout(FlowLayout.TRAILING));
buttonPanel.add(getSaveButton(), null);
buttonPanel.add(getDeleteButton(), null);
buttonPanel.add(getNewButton(), null);
buttonPanel.add(getCloseButton(), null);
}
return buttonPanel;
}
/**
* This method initializes registerButton
*
* @return javax.swing.JButton
*/
private JButton getSaveButton() {
if (saveButton == null) {
saveButton = new KSaveButton();
saveButton.setEnabled(false);
saveButton.addActionListener(this);
}
return saveButton;
}
/**
* This method initializes exitButton
*
* @return org.ferris.journal.exit.ExitButton
*/
private JButton getCloseButton() {
if (closeButton == null) {
closeButton = new KCloseButton();
closeButton.addActionListener(this);
}
return closeButton;
}
/**
* This method initializes statusPanel
*
* @return javax.swing.JPanel
*/
private JPanel getStatusPanel() {
if (statusPanel == null) {
FlowLayout flowLayout = new FlowLayout();
flowLayout.setHgap(0);
flowLayout.setAlignment(java.awt.FlowLayout.LEFT);
flowLayout.setVgap(0);
statusLabel = getStatusLabel();
statusPanel = new JPanel();
statusPanel.setLayout(flowLayout);
statusPanel.setBorder(BorderFactory.createBevelBorder(BevelBorder.LOWERED));
statusPanel.add(statusLabel, null);
}
return statusPanel;
}
/**
* This method initializes journalsScrollPane
*
* @return javax.swing.JScrollPane
*/
private JScrollPane getJournalsScrollPane() {
if (journalsScrollPane == null) {
journalsScrollPane = new JScrollPane();
journalsScrollPane.setViewportView(getJournalsList());
}
return journalsScrollPane;
}
/**
* This method initializes journalsList
*
* @return javax.swing.JList
*/
private KJournalList getJournalsList() {
if (journalsList == null) {
if (this.model == null) {
this.model = new ModelForVisualEditor();
}
journalsList = new KJournalList(
new KJournalListModelForAllJournals(model)
);
journalsList.addListSelectionListener(this);
}
return journalsList;
}
/**
* This method initializes inputPanel
*
* @return javax.swing.JPanel
*/
private JPanel getInputPanel() {
if (inputPanel == null) {
GridBagConstraints gridBagConstraints10 = new GridBagConstraints();
gridBagConstraints10.gridx = 0;
gridBagConstraints10.weighty = 1.0;
gridBagConstraints10.gridy = 2;
spacer = new JLabel();
spacer.setText(" ");
GridBagConstraints gridBagConstraints9 = new GridBagConstraints();
gridBagConstraints9.gridx = 1;
gridBagConstraints9.anchor = GridBagConstraints.WEST;
gridBagConstraints9.insets = new Insets(0, 5, 0, 5);
gridBagConstraints9.gridy = 1;
GridBagConstraints gridBagConstraints8 = new GridBagConstraints();
gridBagConstraints8.gridx = 0;
gridBagConstraints8.anchor = GridBagConstraints.EAST;
gridBagConstraints8.insets = new Insets(8, 5, 8, 5);
gridBagConstraints8.weighty = 0.0;
gridBagConstraints8.gridheight = 1;
gridBagConstraints8.gridy = 1;
activeLabel = new JLabel();
activeLabel.setText("Active :");
GridBagConstraints gridBagConstraints7 = new GridBagConstraints();
gridBagConstraints7.fill = GridBagConstraints.HORIZONTAL;
gridBagConstraints7.gridy = 0;
gridBagConstraints7.weightx = 1.0;
gridBagConstraints7.insets = new Insets(0, 5, 0, 5);
gridBagConstraints7.gridx = 1;
GridBagConstraints gridBagConstraints6 = new GridBagConstraints();
gridBagConstraints6.gridx = 0;
gridBagConstraints6.anchor = GridBagConstraints.EAST;
gridBagConstraints6.insets = new Insets(8, 5, 8, 5);
gridBagConstraints6.gridy = 0;
nameLabel = new JLabel();
nameLabel.setText("Name :");
inputPanel = new JPanel();
inputPanel.setLayout(new GridBagLayout());
inputPanel.add(nameLabel, gridBagConstraints6);
inputPanel.add(getNameText(), gridBagConstraints7);
inputPanel.add(activeLabel, gridBagConstraints8);
inputPanel.add(getActiveCheckBox(), gridBagConstraints9);
inputPanel.add(spacer, gridBagConstraints10);
}
return inputPanel;
}
/**
* This method initializes nameText
*
* @return javax.swing.JTextField
*/
private KJournalNameTextField getNameText() {
if (nameText == null) {
nameText = new KJournalNameTextField();
nameText.getDocument().addDocumentListener(this);
}
return nameText;
}
/**
* This method initializes activeCheckBox
*
* @return javax.swing.JCheckBox
*/
private JCheckBox getActiveCheckBox() {
if (activeCheckBox == null) {
activeCheckBox = new JCheckBox();
activeCheckBox.setSelected(true);
activeCheckBox.addItemListener(this);
}
return activeCheckBox;
}
/**
* This method initializes mainSplitPane
*
* @return javax.swing.JSplitPane
*/
private JSplitPane getMainSplitPane() {
if (mainSplitPane == null) {
mainSplitPane = new JSplitPane();
mainSplitPane.setDividerSize(8);
mainSplitPane.setResizeWeight(0.0D);
mainSplitPane.setOneTouchExpandable(true);
mainSplitPane.setDividerLocation(150);
mainSplitPane.setRightComponent(getInputPanel());
mainSplitPane.setLeftComponent(getJournalsScrollPane());
}
return mainSplitPane;
}
/**
* This method initializes deleteButton
*
* @return javax.swing.JButton
*/
private JButton getDeleteButton() {
if (deleteButton == null) {
deleteButton = new KDeleteButton();
deleteButton.addActionListener(this);
deleteButton.setVisible(false);
}
return deleteButton;
}
/////////////////////////////////////////////////////////////////
//
// WindowFocusListener
//
/////////////////////////////////////////////////////////////////
public void windowGainedFocus(WindowEvent e) {
this.model.registerObserver((OperationObserver)this);
}
public void windowLostFocus(WindowEvent e) {
this.model.removeObserver((OperationObserver)this);
}
/////////////////////////////////////////////////////////////////
//
// ListSelectionListener
//
/////////////////////////////////////////////////////////////////
public void valueChanged(ListSelectionEvent e)
{
log.info("ENTER: valueChanged()");
try {
int selectedIndex
= getJournalsList().getSelectedIndex();
log.info("Now selected: " + selectedIndex);
if (selectedIndex >= 0)
{
final Journal selectedJournal
= getJournalsList().getJournalListModel().getJournalAt(selectedIndex);
Thread t = new Thread() {
public void run() {
setEditing(selectedJournal);
}
};
t.start();
}
}
finally {
}
log.info("EXIT: valueChanged()");
}
/////////////////////////////////////////////////////////////////
//
// ReleaseObserver
//
/////////////////////////////////////////////////////////////////
public void released() {
log.info("ENTER: relase()");
System.out.println("Are you sure you want to exit?");
}
/////////////////////////////////////////////////////////////////
//
// ActionListener
//
/////////////////////////////////////////////////////////////////
public void actionPerformed(ActionEvent evnt)
{
if ("close".equals(evnt.getActionCommand()))
{
controller.closeFrame();
}
else
if ("new".equals(evnt.getActionCommand()))
{
controller.newJournal();
}
else
if ("delete".equals(evnt.getActionCommand()))
{
String msg
= getString("journal.delete.msg");
String title
= getString("journal.delete.title");
Object[] options = { getString("yes"), getString("no") };
int result =
JOptionPane.showOptionDialog(
this //parent component
, msg //Object message
, title //String title
, JOptionPane.DEFAULT_OPTION//int optionType
, JOptionPane.WARNING_MESSAGE//int messageType
, null//Icon icon
, options//Object[] options
, options[1]//Object initialValue
);
log.info("Result: " + result);
if (result == 0)
{
Journal j
= (Journal)getJournalsList().getSelectedValue();
controller.deleteJournal(j);
}
}
else
if ("save".equals(evnt.getActionCommand()))
{
// Error check
List
explainations = new ArrayList();
getNameText().validate(explainations);
if (!explainations.isEmpty()) {
new KErrorDialog(this, explainations);
return;
}
// Insert
if (editing == null)
{
controller.insertJournal(
getNameText().getText()
, getActiveCheckBox().isSelected()
);
}
// Update
else
{
controller.updateJournal(
editing.getId()
, getNameText().getText()
, getActiveCheckBox().isSelected()
);
}
}
else
{
new ThrowableDialog(
new RuntimeException(
getString("exception.actioncommand.not.recognized", evnt.getActionCommand()) // @jve
)
, false
);
}
}
/////////////////////////////////////////////////////////////////
//
// JournalObserver
//
/////////////////////////////////////////////////////////////////
public void deletedJournal(Journal deletedJournl)
{
log.info("ENTER: deletedJournal()");
getJournalsList().getJournalListModel().deletedJournal(deletedJournl);
//getJournalsList().setSelectedValue(null, true);
setEditing(null);
log.info("EXIT: deletedJournal()");
}
public void insertedJournal(Journal newJournal)
{
log.info("ENTER: insertedJournal()");
Long setSelected = newJournal.getId();
log.info("ID of new journal: " + setSelected);
getJournalsList().getJournalListModel().insertedJournal(newJournal);
getJournalsList().setSelectedValue(newJournal, true);
log.info("EXIT: insertedJournal()");
}
public void updatedJournal(Journal updatedJournal)
{
log.info("ENTER: updatedJournal()");
Long setSelected = updatedJournal.getId();
log.info("ID of updated journal: " + setSelected);
getJournalsList().getJournalListModel().updatedJournal(updatedJournal);
getJournalsList().setSelectedValue(updatedJournal, true);
log.info("EXIT: updatedJournal()");
}
public void editJournal(Journal editMe)
{
log.info("ENTER: editJournal()");
setEditing(editMe);
log.info("EXIT: editJournal()");
}
public void newJournal()
{
log.info("ENTER: newJournal()");
setEditing(null);
log.info("EXIT: newJournal()");
}
/////////////////////////////////////////////////////////////////
//
// DocumentListener
//
/////////////////////////////////////////////////////////////////
public void changedUpdate(DocumentEvent e) {
if (!getSaveButton().isEnabled()) {
getSaveButton().setEnabled(true);
}
}
public void removeUpdate(DocumentEvent e) {
if (!getSaveButton().isEnabled()) {
getSaveButton().setEnabled(true);
}
}
public void insertUpdate(DocumentEvent e) {
if (!getSaveButton().isEnabled()) {
getSaveButton().setEnabled(true);
}
}
/////////////////////////////////////////////////////////////////
//
// ItemListener
//
/////////////////////////////////////////////////////////////////
public void itemStateChanged(ItemEvent e)
{
switch (e.getStateChange()) {
case ItemEvent.DESELECTED:
case ItemEvent.SELECTED:
log.info("*** Enable save: itemStateChanged");
if (!getSaveButton().isEnabled()) {
getSaveButton().setEnabled(true);
}
break;
default:
break;
}
}
/////////////////////////////////////////////////////////////////
//
// OperationObserver
//
/////////////////////////////////////////////////////////////////
public void performingOperation(final String description) {
InvokeAndWait.run(
new Runnable() {
public void run() {
getStatusLabel().setText(description);
}
}
);
}
/////////////////////////////////////////////////////////////////
//
// ----------------------- Helpers ------------------------------
//
/////////////////////////////////////////////////////////////////
private void registerObservers() {
this.model.registerObserver((ReleaseObserver)this);
this.model.registerObserver((OperationObserver)this);
this.model.registerObserver((JournalObserver)this);
}
private void removeObservers() {
this.model.removeObserver((ReleaseObserver)this);
this.model.removeObserver((OperationObserver)this);
this.model.removeObserver((JournalObserver)this);
}
private void setEditing(final Journal selectedJournal)
{
log.info("ENTER: setEditing()");
// Editing
this.editing = selectedJournal;
if (selectedJournal != null)
{
// Name
getNameText().getDocument().removeDocumentListener(KJournalsFrame.this);
{
getNameText().setText(selectedJournal.getName());
getNameText().setSelectionStart(0);
getNameText().setSelectionEnd(getNameText().getText().length());
getNameText().grabFocus();
}
getNameText().getDocument().addDocumentListener(KJournalsFrame.this);
// Active
getActiveCheckBox().removeItemListener(KJournalsFrame.this);
{
getActiveCheckBox().setSelected(selectedJournal.getIsActive());
}
getActiveCheckBox().addItemListener(KJournalsFrame.this);
// Buttons
getDeleteButton().setVisible(true);
log.info("Disable save button");
getSaveButton().setEnabled(false);
}
else
{
// Name
getNameText().getDocument().removeDocumentListener(KJournalsFrame.this);
{
getNameText().setText("");
getNameText().grabFocus();
}
getNameText().getDocument().addDocumentListener(KJournalsFrame.this);
// Active
getActiveCheckBox().removeItemListener(KJournalsFrame.this);
{
getActiveCheckBox().setSelected(true);
}
getActiveCheckBox().addItemListener(KJournalsFrame.this);
// Buttons
getDeleteButton().setVisible(false);
log.info("Disable save and set selected index");
getSaveButton().setEnabled(false);
getJournalsList().clearSelection();
}
log.info("EXIT: setEditing()");
}
} // @jve:decl-index=0:visual-constraint="56,4"
© 2015 - 2025 Weber Informatics LLC | Privacy Policy