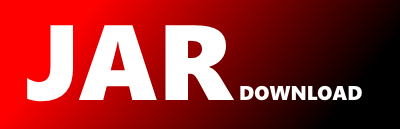
org.ferris.journal.jws.journalentry.JournalEntry Maven / Gradle / Ivy
/**
* JournalEntry.java
*
* This file was auto-generated from WSDL
* by the Apache Axis 1.3 Oct 05, 2005 (05:23:37 EDT) WSDL2Java emitter.
*/
package org.ferris.journal.jws.journalentry;
public class JournalEntry implements java.io.Serializable {
private org.ferris.journal.jws.account.Account account;
private java.util.Calendar createdOn;
private java.util.Calendar day;
private java.lang.String entry;
private java.lang.Long id;
private org.ferris.journal.jws.journal.Journal journal;
private java.lang.String subject;
public JournalEntry() {
}
public JournalEntry(
org.ferris.journal.jws.account.Account account,
java.util.Calendar createdOn,
java.util.Calendar day,
java.lang.String entry,
java.lang.Long id,
org.ferris.journal.jws.journal.Journal journal,
java.lang.String subject) {
this.account = account;
this.createdOn = createdOn;
this.day = day;
this.entry = entry;
this.id = id;
this.journal = journal;
this.subject = subject;
}
/**
* Gets the account value for this JournalEntry.
*
* @return account
*/
public org.ferris.journal.jws.account.Account getAccount() {
return account;
}
/**
* Sets the account value for this JournalEntry.
*
* @param account
*/
public void setAccount(org.ferris.journal.jws.account.Account account) {
this.account = account;
}
/**
* Gets the createdOn value for this JournalEntry.
*
* @return createdOn
*/
public java.util.Calendar getCreatedOn() {
return createdOn;
}
/**
* Sets the createdOn value for this JournalEntry.
*
* @param createdOn
*/
public void setCreatedOn(java.util.Calendar createdOn) {
this.createdOn = createdOn;
}
/**
* Gets the day value for this JournalEntry.
*
* @return day
*/
public java.util.Calendar getDay() {
return day;
}
/**
* Sets the day value for this JournalEntry.
*
* @param day
*/
public void setDay(java.util.Calendar day) {
this.day = day;
}
/**
* Gets the entry value for this JournalEntry.
*
* @return entry
*/
public java.lang.String getEntry() {
return entry;
}
/**
* Sets the entry value for this JournalEntry.
*
* @param entry
*/
public void setEntry(java.lang.String entry) {
this.entry = entry;
}
/**
* Gets the id value for this JournalEntry.
*
* @return id
*/
public java.lang.Long getId() {
return id;
}
/**
* Sets the id value for this JournalEntry.
*
* @param id
*/
public void setId(java.lang.Long id) {
this.id = id;
}
/**
* Gets the journal value for this JournalEntry.
*
* @return journal
*/
public org.ferris.journal.jws.journal.Journal getJournal() {
return journal;
}
/**
* Sets the journal value for this JournalEntry.
*
* @param journal
*/
public void setJournal(org.ferris.journal.jws.journal.Journal journal) {
this.journal = journal;
}
/**
* Gets the subject value for this JournalEntry.
*
* @return subject
*/
public java.lang.String getSubject() {
return subject;
}
/**
* Sets the subject value for this JournalEntry.
*
* @param subject
*/
public void setSubject(java.lang.String subject) {
this.subject = subject;
}
private java.lang.Object __equalsCalc = null;
public synchronized boolean equals(java.lang.Object obj) {
if (!(obj instanceof JournalEntry)) return false;
JournalEntry other = (JournalEntry) obj;
if (obj == null) return false;
if (this == obj) return true;
if (__equalsCalc != null) {
return (__equalsCalc == obj);
}
__equalsCalc = obj;
boolean _equals;
_equals = true &&
((this.account==null && other.getAccount()==null) ||
(this.account!=null &&
this.account.equals(other.getAccount()))) &&
((this.createdOn==null && other.getCreatedOn()==null) ||
(this.createdOn!=null &&
this.createdOn.equals(other.getCreatedOn()))) &&
((this.day==null && other.getDay()==null) ||
(this.day!=null &&
this.day.equals(other.getDay()))) &&
((this.entry==null && other.getEntry()==null) ||
(this.entry!=null &&
this.entry.equals(other.getEntry()))) &&
((this.id==null && other.getId()==null) ||
(this.id!=null &&
this.id.equals(other.getId()))) &&
((this.journal==null && other.getJournal()==null) ||
(this.journal!=null &&
this.journal.equals(other.getJournal()))) &&
((this.subject==null && other.getSubject()==null) ||
(this.subject!=null &&
this.subject.equals(other.getSubject())));
__equalsCalc = null;
return _equals;
}
private boolean __hashCodeCalc = false;
public synchronized int hashCode() {
if (__hashCodeCalc) {
return 0;
}
__hashCodeCalc = true;
int _hashCode = 1;
if (getAccount() != null) {
_hashCode += getAccount().hashCode();
}
if (getCreatedOn() != null) {
_hashCode += getCreatedOn().hashCode();
}
if (getDay() != null) {
_hashCode += getDay().hashCode();
}
if (getEntry() != null) {
_hashCode += getEntry().hashCode();
}
if (getId() != null) {
_hashCode += getId().hashCode();
}
if (getJournal() != null) {
_hashCode += getJournal().hashCode();
}
if (getSubject() != null) {
_hashCode += getSubject().hashCode();
}
__hashCodeCalc = false;
return _hashCode;
}
// Type metadata
private static org.apache.axis.description.TypeDesc typeDesc =
new org.apache.axis.description.TypeDesc(JournalEntry.class, true);
static {
typeDesc.setXmlType(new javax.xml.namespace.QName("http://journalentry.jws.journal.ferris.org/", "journalEntry"));
org.apache.axis.description.ElementDesc elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("account");
elemField.setXmlName(new javax.xml.namespace.QName("", "account"));
elemField.setXmlType(new javax.xml.namespace.QName("http://journalentry.jws.journal.ferris.org/", "account"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("createdOn");
elemField.setXmlName(new javax.xml.namespace.QName("", "createdOn"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("day");
elemField.setXmlName(new javax.xml.namespace.QName("", "day"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "dateTime"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("entry");
elemField.setXmlName(new javax.xml.namespace.QName("", "entry"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("id");
elemField.setXmlName(new javax.xml.namespace.QName("", "id"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "long"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("journal");
elemField.setXmlName(new javax.xml.namespace.QName("", "journal"));
elemField.setXmlType(new javax.xml.namespace.QName("http://journalentry.jws.journal.ferris.org/", "journal"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
elemField = new org.apache.axis.description.ElementDesc();
elemField.setFieldName("subject");
elemField.setXmlName(new javax.xml.namespace.QName("", "subject"));
elemField.setXmlType(new javax.xml.namespace.QName("http://www.w3.org/2001/XMLSchema", "string"));
elemField.setMinOccurs(0);
elemField.setNillable(false);
typeDesc.addFieldDesc(elemField);
}
/**
* Return type metadata object
*/
public static org.apache.axis.description.TypeDesc getTypeDesc() {
return typeDesc;
}
/**
* Get Custom Serializer
*/
public static org.apache.axis.encoding.Serializer getSerializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanSerializer(
_javaType, _xmlType, typeDesc);
}
/**
* Get Custom Deserializer
*/
public static org.apache.axis.encoding.Deserializer getDeserializer(
java.lang.String mechType,
java.lang.Class _javaType,
javax.xml.namespace.QName _xmlType) {
return
new org.apache.axis.encoding.ser.BeanDeserializer(
_javaType, _xmlType, typeDesc);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy