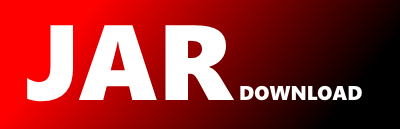
cdm.event.common.functions.Create_Cashflow Maven / Gradle / Ivy
package cdm.event.common.functions;
import cdm.base.math.NonNegativeQuantitySchedule;
import cdm.base.math.UnitType;
import cdm.base.staticdata.party.PayerReceiver;
import cdm.product.common.settlement.Cashflow;
import cdm.product.common.settlement.Cashflow.CashflowBuilder;
import cdm.product.common.settlement.CashflowType;
import cdm.product.common.settlement.PaymentDiscounting;
import cdm.product.common.settlement.ResolvablePriceQuantity;
import cdm.product.common.settlement.SettlementDate;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperS;
import java.math.BigDecimal;
import java.util.Optional;
import javax.inject.Inject;
@ImplementedBy(Create_Cashflow.Create_CashflowDefault.class)
public abstract class Create_Cashflow implements RosettaFunction {
@Inject protected ModelObjectValidator objectValidator;
/**
* @param amount
* @param currency
* @param settlementDate
* @param payerReceiver
* @param cashflowType
* @param paymentDiscounting
* @return cashflow
*/
public Cashflow evaluate(BigDecimal amount, String currency, SettlementDate settlementDate, PayerReceiver payerReceiver, CashflowType cashflowType, PaymentDiscounting paymentDiscounting) {
Cashflow.CashflowBuilder cashflowBuilder = doEvaluate(amount, currency, settlementDate, payerReceiver, cashflowType, paymentDiscounting);
final Cashflow cashflow;
if (cashflowBuilder == null) {
cashflow = null;
} else {
cashflow = cashflowBuilder.build();
objectValidator.validate(Cashflow.class, cashflow);
}
return cashflow;
}
protected abstract Cashflow.CashflowBuilder doEvaluate(BigDecimal amount, String currency, SettlementDate settlementDate, PayerReceiver payerReceiver, CashflowType cashflowType, PaymentDiscounting paymentDiscounting);
public static class Create_CashflowDefault extends Create_Cashflow {
@Override
protected Cashflow.CashflowBuilder doEvaluate(BigDecimal amount, String currency, SettlementDate settlementDate, PayerReceiver payerReceiver, CashflowType cashflowType, PaymentDiscounting paymentDiscounting) {
Cashflow.CashflowBuilder cashflow = Cashflow.builder();
return assignOutput(cashflow, amount, currency, settlementDate, payerReceiver, cashflowType, paymentDiscounting);
}
protected Cashflow.CashflowBuilder assignOutput(Cashflow.CashflowBuilder cashflow, BigDecimal amount, String currency, SettlementDate settlementDate, PayerReceiver payerReceiver, CashflowType cashflowType, PaymentDiscounting paymentDiscounting) {
cashflow
.getOrCreateSettlementTerms()
.setSettlementDate(MapperS.of(settlementDate).get());
cashflow
.setPayerReceiver(MapperS.of(payerReceiver).get());
cashflow
.setCashflowType(MapperS.of(cashflowType).get());
cashflow
.setPaymentDiscounting(MapperS.of(paymentDiscounting).get());
cashflow
.setPriceQuantity(MapperS.of(ResolvablePriceQuantity.builder()
.setQuantityScheduleValue(MapperS.of(NonNegativeQuantitySchedule.builder()
.setValue(MapperS.of(amount).get())
.setUnit(MapperS.of(UnitType.builder()
.setCurrencyValue(MapperS.of(currency).get())
.build())
.get())
.build())
.get())
.build())
.get());
return Optional.ofNullable(cashflow)
.map(o -> o.prune())
.orElse(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy