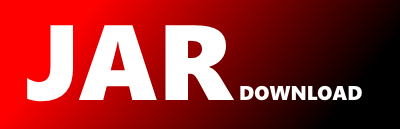
cdm.event.common.functions.Create_SubstitutionInstruction Maven / Gradle / Ivy
package cdm.event.common.functions;
import cdm.base.datetime.AdjustableOrRelativeDate;
import cdm.event.common.CollateralPortfolio;
import cdm.event.common.TermsChangeInstruction;
import cdm.event.common.TermsChangeInstruction.TermsChangeInstructionBuilder;
import cdm.product.template.ContractualProduct;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperS;
import java.util.Optional;
import javax.inject.Inject;
@ImplementedBy(Create_SubstitutionInstruction.Create_SubstitutionInstructionDefault.class)
public abstract class Create_SubstitutionInstruction implements RosettaFunction {
@Inject protected ModelObjectValidator objectValidator;
// RosettaFunction dependencies
//
@Inject protected Create_EffectiveOrTerminationDateTermChangeInstruction create_EffectiveOrTerminationDateTermChangeInstruction;
/**
* @param contractualProduct The original contractual product to be used as the basis of the new trade.
* @param effectiveDate The effective date of the substitution.
* @param newCollateralPortfolio New collateral portfolio to substitute for the original collateral.
* @return termsChangeInstruction
*/
public TermsChangeInstruction evaluate(ContractualProduct contractualProduct, AdjustableOrRelativeDate effectiveDate, CollateralPortfolio newCollateralPortfolio) {
TermsChangeInstruction.TermsChangeInstructionBuilder termsChangeInstructionBuilder = doEvaluate(contractualProduct, effectiveDate, newCollateralPortfolio);
final TermsChangeInstruction termsChangeInstruction;
if (termsChangeInstructionBuilder == null) {
termsChangeInstruction = null;
} else {
termsChangeInstruction = termsChangeInstructionBuilder.build();
objectValidator.validate(TermsChangeInstruction.class, termsChangeInstruction);
}
return termsChangeInstruction;
}
protected abstract TermsChangeInstruction.TermsChangeInstructionBuilder doEvaluate(ContractualProduct contractualProduct, AdjustableOrRelativeDate effectiveDate, CollateralPortfolio newCollateralPortfolio);
public static class Create_SubstitutionInstructionDefault extends Create_SubstitutionInstruction {
@Override
protected TermsChangeInstruction.TermsChangeInstructionBuilder doEvaluate(ContractualProduct contractualProduct, AdjustableOrRelativeDate effectiveDate, CollateralPortfolio newCollateralPortfolio) {
TermsChangeInstruction.TermsChangeInstructionBuilder termsChangeInstruction = TermsChangeInstruction.builder();
return assignOutput(termsChangeInstruction, contractualProduct, effectiveDate, newCollateralPortfolio);
}
protected TermsChangeInstruction.TermsChangeInstructionBuilder assignOutput(TermsChangeInstruction.TermsChangeInstructionBuilder termsChangeInstruction, ContractualProduct contractualProduct, AdjustableOrRelativeDate effectiveDate, CollateralPortfolio newCollateralPortfolio) {
termsChangeInstruction = toBuilder(MapperS.of(create_EffectiveOrTerminationDateTermChangeInstruction.evaluate(MapperS.of(contractualProduct).get(), MapperS.of(effectiveDate).get(), null)).get());
termsChangeInstruction
.getOrCreateProduct()
.getOrCreateContractualProduct()
.getOrCreateEconomicTerms()
.getOrCreateCollateral()
.setCollateralPortfolioValue(MapperS.of(newCollateralPortfolio).getMulti());
return Optional.ofNullable(termsChangeInstruction)
.map(o -> o.prune())
.orElse(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy