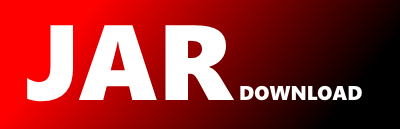
cdm.event.common.functions.Create_TradeState Maven / Gradle / Ivy
package cdm.event.common.functions;
import cdm.base.staticdata.party.AncillaryParty;
import cdm.base.staticdata.party.Counterparty;
import cdm.base.staticdata.party.PartyRole;
import cdm.event.common.ContractFormationInstruction;
import cdm.event.common.ExecutionInstruction;
import cdm.event.common.IndexTransitionInstruction;
import cdm.event.common.ObservationInstruction;
import cdm.event.common.PartyChangeInstruction;
import cdm.event.common.PrimitiveInstruction;
import cdm.event.common.QuantityChangeInstruction;
import cdm.event.common.ResetInstruction;
import cdm.event.common.SplitInstruction;
import cdm.event.common.State;
import cdm.event.common.StockSplitInstruction;
import cdm.event.common.TermsChangeInstruction;
import cdm.event.common.TradeIdentifier;
import cdm.event.common.TradeState;
import cdm.event.common.TradeState.TradeStateBuilder;
import cdm.event.common.TransferInstruction;
import cdm.event.position.PositionStatusEnum;
import cdm.legaldocumentation.common.ClosedState;
import cdm.legaldocumentation.common.ClosedStateEnum;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.expression.CardinalityOperator;
import com.rosetta.model.lib.functions.ConditionValidator;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.Mapper;
import com.rosetta.model.lib.mapper.MapperS;
import com.rosetta.model.lib.mapper.MapperUtils;
import com.rosetta.model.lib.records.Date;
import java.util.Optional;
import javax.inject.Inject;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(Create_TradeState.Create_TradeStateDefault.class)
public abstract class Create_TradeState implements RosettaFunction {
@Inject protected ConditionValidator conditionValidator;
@Inject protected ModelObjectValidator objectValidator;
// RosettaFunction dependencies
//
@Inject protected Create_ContractFormation create_ContractFormation;
@Inject protected Create_Execution create_Execution;
@Inject protected Create_IndexTransitionTermsChange create_IndexTransitionTermsChange;
@Inject protected Create_Observation create_Observation;
@Inject protected Create_PartyChange create_PartyChange;
@Inject protected Create_QuantityChange create_QuantityChange;
@Inject protected Create_Reset create_Reset;
@Inject protected Create_StockSplit create_StockSplit;
@Inject protected Create_TermsChange create_TermsChange;
@Inject protected Create_Transfer create_Transfer;
/**
* @param primitiveInstruction The set of primitive instructions to apply to the trade.
* @param before The original trade on which the primitive instructions are applied
* @return after The returned trade state must be of single cardinality. Where a different trade is created and the original trade must be persisted (for instance showing as 'closed'), it should be preceded by a split instruction.
*/
public TradeState evaluate(PrimitiveInstruction primitiveInstruction, TradeState before) {
// pre-conditions
conditionValidator.validate(() ->
notExists(MapperS.of(primitiveInstruction).map("getSplit", _primitiveInstruction -> _primitiveInstruction.getSplit())),
"The primitive instruction cannot contain a split, as this function is designed to return a single trade state.");
TradeState.TradeStateBuilder afterBuilder = doEvaluate(primitiveInstruction, before);
final TradeState after;
if (afterBuilder == null) {
after = null;
} else {
after = afterBuilder.build();
objectValidator.validate(TradeState.class, after);
}
return after;
}
protected abstract TradeState.TradeStateBuilder doEvaluate(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> execution(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> quantityChange(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> termsChange(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> partyChange(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> contractFormation(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> transfer(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> reset(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> indexTransition(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> observation(PrimitiveInstruction primitiveInstruction, TradeState before);
protected abstract Mapper extends TradeState> stockSplit(PrimitiveInstruction primitiveInstruction, TradeState before);
public static class Create_TradeStateDefault extends Create_TradeState {
@Override
protected TradeState.TradeStateBuilder doEvaluate(PrimitiveInstruction primitiveInstruction, TradeState before) {
TradeState.TradeStateBuilder after = TradeState.builder();
return assignOutput(after, primitiveInstruction, before);
}
protected TradeState.TradeStateBuilder assignOutput(TradeState.TradeStateBuilder after, PrimitiveInstruction primitiveInstruction, TradeState before) {
after = toBuilder(MapperS.of(stockSplit(primitiveInstruction, before).get()).get());
after
.getOrCreateState()
.setClosedState(MapperUtils.runSinglePolymorphic(() -> {
if (areEqual(MapperS.of(contractFormation(primitiveInstruction, before).get()).map("getState", tradeState -> tradeState.getState()).map("getPositionState", state -> state.getPositionState()), MapperS.of(PositionStatusEnum.CLOSED), CardinalityOperator.All).getOrDefault(false)) {
return MapperS.of(ClosedState.builder()
.setState(MapperS.of(ClosedStateEnum.TERMINATED).get())
.setActivityDate(MapperS.ofNull().get())
.build())
;
}
else {
return null;
}
}).get());
return Optional.ofNullable(after)
.map(o -> o.prune())
.orElse(null);
}
@Override
protected Mapper extends TradeState> execution(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getExecution", _primitiveInstruction -> _primitiveInstruction.getExecution())).getOrDefault(false)) {
return MapperS.of(before);
}
else {
return MapperS.of(create_Execution.evaluate(MapperS.of(primitiveInstruction).map("getExecution", _primitiveInstruction -> _primitiveInstruction.getExecution()).get()));
}
});
}
@Override
protected Mapper extends TradeState> quantityChange(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getQuantityChange", _primitiveInstruction -> _primitiveInstruction.getQuantityChange())).getOrDefault(false)) {
return MapperS.of(execution(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_QuantityChange.evaluate(MapperS.of(primitiveInstruction).map("getQuantityChange", _primitiveInstruction -> _primitiveInstruction.getQuantityChange()).get(), MapperS.of(execution(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> termsChange(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getTermsChange", _primitiveInstruction -> _primitiveInstruction.getTermsChange())).getOrDefault(false)) {
return MapperS.of(quantityChange(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_TermsChange.evaluate(MapperS.of(primitiveInstruction).map("getTermsChange", _primitiveInstruction -> _primitiveInstruction.getTermsChange()).get(), MapperS.of(quantityChange(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> partyChange(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getPartyChange", _primitiveInstruction -> _primitiveInstruction.getPartyChange())).getOrDefault(false)) {
return MapperS.of(termsChange(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_PartyChange.evaluate(MapperS.of(primitiveInstruction).map("getPartyChange", _primitiveInstruction -> _primitiveInstruction.getPartyChange()).map("getCounterparty", partyChangeInstruction -> partyChangeInstruction.getCounterparty()).get(), MapperS.of(primitiveInstruction).map("getPartyChange", _primitiveInstruction -> _primitiveInstruction.getPartyChange()).map("getAncillaryParty", partyChangeInstruction -> partyChangeInstruction.getAncillaryParty()).get(), MapperS.of(primitiveInstruction).map("getPartyChange", _primitiveInstruction -> _primitiveInstruction.getPartyChange()).map("getPartyRole", partyChangeInstruction -> partyChangeInstruction.getPartyRole()).get(), MapperS.of(primitiveInstruction).map("getPartyChange", _primitiveInstruction -> _primitiveInstruction.getPartyChange()).mapC("getTradeId", partyChangeInstruction -> partyChangeInstruction.getTradeId()).getMulti(), MapperS.of(termsChange(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> contractFormation(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (exists(MapperS.of(primitiveInstruction)).and(notExists(MapperS.of(primitiveInstruction).map("getContractFormation", _primitiveInstruction -> _primitiveInstruction.getContractFormation()))).getOrDefault(false)) {
return MapperS.of(partyChange(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_ContractFormation.evaluate(MapperS.of(primitiveInstruction).map("getContractFormation", _primitiveInstruction -> _primitiveInstruction.getContractFormation()).get(), MapperS.of(partyChange(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> transfer(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getTransfer", _primitiveInstruction -> _primitiveInstruction.getTransfer())).getOrDefault(false)) {
return MapperS.of(contractFormation(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_Transfer.evaluate(MapperS.of(primitiveInstruction).map("getTransfer", _primitiveInstruction -> _primitiveInstruction.getTransfer()).get(), MapperS.of(contractFormation(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> reset(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getReset", _primitiveInstruction -> _primitiveInstruction.getReset())).getOrDefault(false)) {
return MapperS.of(transfer(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_Reset.evaluate(MapperS.of(primitiveInstruction).map("getReset", _primitiveInstruction -> _primitiveInstruction.getReset()).get(), MapperS.of(transfer(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> indexTransition(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getIndexTransition", _primitiveInstruction -> _primitiveInstruction.getIndexTransition())).getOrDefault(false)) {
return MapperS.of(reset(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_IndexTransitionTermsChange.evaluate(MapperS.of(primitiveInstruction).map("getIndexTransition", _primitiveInstruction -> _primitiveInstruction.getIndexTransition()).get(), MapperS.of(reset(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> observation(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getObservation", _primitiveInstruction -> _primitiveInstruction.getObservation())).getOrDefault(false)) {
return MapperS.of(indexTransition(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_Observation.evaluate(MapperS.of(primitiveInstruction).map("getObservation", _primitiveInstruction -> _primitiveInstruction.getObservation()).get(), MapperS.of(indexTransition(primitiveInstruction, before).get()).get()));
}
});
}
@Override
protected Mapper extends TradeState> stockSplit(PrimitiveInstruction primitiveInstruction, TradeState before) {
return MapperUtils.runSinglePolymorphic(() -> {
if (notExists(MapperS.of(primitiveInstruction).map("getStockSplit", _primitiveInstruction -> _primitiveInstruction.getStockSplit())).getOrDefault(false)) {
return MapperS.of(observation(primitiveInstruction, before).get());
}
else {
return MapperS.of(create_StockSplit.evaluate(MapperS.of(primitiveInstruction).map("getStockSplit", _primitiveInstruction -> _primitiveInstruction.getStockSplit()).get(), MapperS.of(observation(primitiveInstruction, before).get()).get()));
}
});
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy