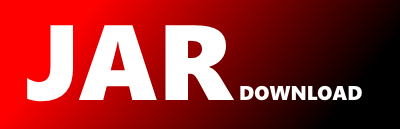
cdm.event.common.functions.FindMatchingIndexTransitionInstruction Maven / Gradle / Ivy
package cdm.event.common.functions;
import cdm.base.datetime.Period;
import cdm.base.datetime.PeriodEnum;
import cdm.base.math.NonNegativeQuantitySchedule;
import cdm.base.math.UnitType;
import cdm.base.math.metafields.FieldWithMetaNonNegativeQuantitySchedule;
import cdm.observable.asset.FloatingRateOption;
import cdm.observable.asset.Observable;
import cdm.observable.asset.PriceSchedule;
import cdm.observable.asset.metafields.FieldWithMetaFloatingRateOption;
import cdm.observable.asset.metafields.FieldWithMetaPriceSchedule;
import cdm.product.common.settlement.PriceQuantity;
import cdm.product.common.settlement.PriceQuantity.PriceQuantityBuilder;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.expression.CardinalityOperator;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperC;
import com.rosetta.model.lib.mapper.MapperS;
import com.rosetta.model.metafields.FieldWithMetaString;
import java.util.List;
import java.util.Optional;
import javax.inject.Inject;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(FindMatchingIndexTransitionInstruction.FindMatchingIndexTransitionInstructionDefault.class)
public abstract class FindMatchingIndexTransitionInstruction implements RosettaFunction {
@Inject protected ModelObjectValidator objectValidator;
/**
* @param instructions
* @param priceQuantity
* @return matchingInstruction
*/
public PriceQuantity evaluate(List extends PriceQuantity> instructions, PriceQuantity priceQuantity) {
PriceQuantity.PriceQuantityBuilder matchingInstructionBuilder = doEvaluate(instructions, priceQuantity);
final PriceQuantity matchingInstruction;
if (matchingInstructionBuilder == null) {
matchingInstruction = null;
} else {
matchingInstruction = matchingInstructionBuilder.build();
objectValidator.validate(PriceQuantity.class, matchingInstruction);
}
return matchingInstruction;
}
protected abstract PriceQuantity.PriceQuantityBuilder doEvaluate(List extends PriceQuantity> instructions, PriceQuantity priceQuantity);
public static class FindMatchingIndexTransitionInstructionDefault extends FindMatchingIndexTransitionInstruction {
@Override
protected PriceQuantity.PriceQuantityBuilder doEvaluate(List extends PriceQuantity> instructions, PriceQuantity priceQuantity) {
PriceQuantity.PriceQuantityBuilder matchingInstruction = PriceQuantity.builder();
return assignOutput(matchingInstruction, instructions, priceQuantity);
}
protected PriceQuantity.PriceQuantityBuilder assignOutput(PriceQuantity.PriceQuantityBuilder matchingInstruction, List extends PriceQuantity> instructions, PriceQuantity priceQuantity) {
matchingInstruction = toBuilder(MapperC.of(instructions)
.filterItemNullSafe(item -> (Boolean)areEqual(item.map("getObservable", _priceQuantity -> _priceQuantity.getObservable()).map("getRateOption", observable -> observable.getRateOption()).map("getValue", _f->_f.getValue()).map("getIndexTenor", floatingRateOption -> floatingRateOption.getIndexTenor()).map("getPeriod", period -> period.getPeriod()), MapperS.of(priceQuantity).map("getObservable", _priceQuantity -> _priceQuantity.getObservable()).map("getRateOption", observable -> observable.getRateOption()).map("getValue", _f->_f.getValue()).map("getIndexTenor", floatingRateOption -> floatingRateOption.getIndexTenor()).map("getPeriod", period -> period.getPeriod()), CardinalityOperator.All).and(areEqual(item.map("getObservable", _priceQuantity -> _priceQuantity.getObservable()).map("getRateOption", observable -> observable.getRateOption()).map("getValue", _f->_f.getValue()).map("getIndexTenor", floatingRateOption -> floatingRateOption.getIndexTenor()).map("getPeriodMultiplier", period -> period.getPeriodMultiplier()), MapperS.of(priceQuantity).map("getObservable", _priceQuantity -> _priceQuantity.getObservable()).map("getRateOption", observable -> observable.getRateOption()).map("getValue", _f->_f.getValue()).map("getIndexTenor", floatingRateOption -> floatingRateOption.getIndexTenor()).map("getPeriodMultiplier", period -> period.getPeriodMultiplier()), CardinalityOperator.All)).and(areEqual(item.mapC("getQuantity", _priceQuantity -> _priceQuantity.getQuantity()).map("getValue", _f->_f.getValue()).map("getUnit", measureBase -> measureBase.getUnit()).map("getCurrency", unitType -> unitType.getCurrency()).map("getValue", _f->_f.getValue()), MapperS.of(priceQuantity).mapC("getQuantity", _priceQuantity -> _priceQuantity.getQuantity()).map("getValue", _f->_f.getValue()).map("getUnit", measureBase -> measureBase.getUnit()).map("getCurrency", unitType -> unitType.getCurrency()).map("getValue", _f->_f.getValue()), CardinalityOperator.All).or(areEqual(item.mapC("getPrice", _priceQuantity -> _priceQuantity.getPrice()).map("getValue", _f->_f.getValue()).map("getUnit", measureBase -> measureBase.getUnit()).map("getCurrency", unitType -> unitType.getCurrency()).map("getValue", _f->_f.getValue()), MapperS.of(priceQuantity).mapC("getPrice", _priceQuantity -> _priceQuantity.getPrice()).map("getValue", _f->_f.getValue()).map("getUnit", measureBase -> measureBase.getUnit()).map("getCurrency", unitType -> unitType.getCurrency()).map("getValue", _f->_f.getValue()), CardinalityOperator.All))).get())
.apply(item -> item
.first()).get());
return Optional.ofNullable(matchingInstruction)
.map(o -> o.prune())
.orElse(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy