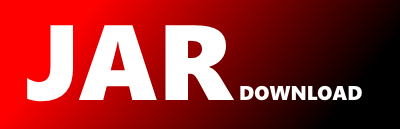
src.cdm.product.collateral.AssetCriteria.py Maven / Gradle / Ivy
# pylint: disable=line-too-long, invalid-name, missing-function-docstring
# pylint: disable=bad-indentation, trailing-whitespace, superfluous-parens
# pylint: disable=wrong-import-position, unused-import, unused-wildcard-import
# pylint: disable=wildcard-import, wrong-import-order, missing-class-docstring
# pylint: disable=missing-module-docstring
from __future__ import annotations
from typing import List, Optional
import datetime
import inspect
from decimal import Decimal
from pydantic import Field
from rosetta.runtime.utils import (
BaseDataClass, rosetta_condition, rosetta_resolve_attr
)
from rosetta.runtime.utils import *
__all__ = ['AssetCriteria']
class AssetCriteria(BaseDataClass):
"""
Represents a set of criteria used to specify eligible collateral assets.
"""
collateralAssetType: List[cdm.base.staticdata.asset.common.AssetType.AssetType] = Field([], description="Represents a filter based on the asset product type.")
"""
Represents a filter based on the asset product type.
"""
assetCountryOfOrigin: List[cdm.base.staticdata.asset.common.ISOCountryCodeEnum.ISOCountryCodeEnum] = Field([], description="Represents a filter on the asset country of origin based on the ISO Standard 3166.")
"""
Represents a filter on the asset country of origin based on the ISO Standard 3166.
"""
denominatedCurrency: List[cdm.base.staticdata.asset.common.CurrencyCodeEnum.CurrencyCodeEnum] = Field([], description="Represents a filter on the underlying asset denominated currency based on ISO Standards.")
"""
Represents a filter on the underlying asset denominated currency based on ISO Standards.
"""
agencyRating: List[cdm.product.collateral.AgencyRatingCriteria.AgencyRatingCriteria] = Field([], description="Represents an agency rating based on default risk and creditors claim in event of default associated with specific instrument.")
"""
Represents an agency rating based on default risk and creditors claim in event of default associated with specific instrument.
"""
maturityType: Optional[cdm.base.staticdata.asset.common.MaturityTypeEnum.MaturityTypeEnum] = Field(None, description="Specifies whether the maturity range is the remaining or original maturity.")
"""
Specifies whether the maturity range is the remaining or original maturity.
"""
maturityRange: Optional[cdm.base.datetime.PeriodRange.PeriodRange] = Field(None, description="Represents a filter based on the underlying asset maturity.")
"""
Represents a filter based on the underlying asset maturity.
"""
productIdentifier: List[cdm.base.staticdata.asset.common.ProductIdentifier.ProductIdentifier] = Field([], description="Represents a filter based on specific instrument identifiers (e.g. specific ISINs, CUSIPs etc).")
"""
Represents a filter based on specific instrument identifiers (e.g. specific ISINs, CUSIPs etc).
"""
collateralTaxonomy: List[cdm.base.staticdata.asset.common.CollateralTaxonomy.CollateralTaxonomy] = Field([], description="Specifies the collateral taxonomy,which is composed of a taxonomy value and a taxonomy source.")
"""
Specifies the collateral taxonomy,which is composed of a taxonomy value and a taxonomy source.
"""
domesticCurrencyIssued: Optional[bool] = Field(None, description="Identifies that the Security must be denominated in the domestic currency of the issuer.")
"""
Identifies that the Security must be denominated in the domestic currency of the issuer.
"""
listing: Optional[cdm.product.collateral.ListingType.ListingType] = Field(None, description="Specifies the exchange, index or sector specific to listing of a security.")
"""
Specifies the exchange, index or sector specific to listing of a security.
"""
@rosetta_condition
def condition_0_AssetCriteriaChoice(self):
"""
If any are specified, only one of AssetType, ProductTaxonomy or ProductIdentifer should exist.
"""
item = self
return self.check_one_of_constraint('collateralAssetType', 'collateralTaxonomy', 'productIdentifier', necessity=False)
import cdm
import cdm.base.staticdata.asset.common.AssetType
import cdm.base.staticdata.asset.common.ISOCountryCodeEnum
import cdm.base.staticdata.asset.common.CurrencyCodeEnum
import cdm.product.collateral.AgencyRatingCriteria
import cdm.base.staticdata.asset.common.MaturityTypeEnum
import cdm.base.datetime.PeriodRange
import cdm.base.staticdata.asset.common.ProductIdentifier
import cdm.base.staticdata.asset.common.CollateralTaxonomy
import cdm.product.collateral.ListingType
© 2015 - 2025 Weber Informatics LLC | Privacy Policy