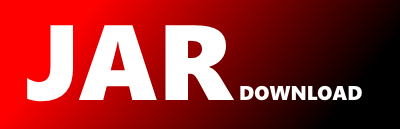
src.cdm.product.template.ForwardPayout.py Maven / Gradle / Ivy
# pylint: disable=line-too-long, invalid-name, missing-function-docstring
# pylint: disable=bad-indentation, trailing-whitespace, superfluous-parens
# pylint: disable=wrong-import-position, unused-import, unused-wildcard-import
# pylint: disable=wildcard-import, wrong-import-order, missing-class-docstring
# pylint: disable=missing-module-docstring
from __future__ import annotations
from typing import List, Optional
import datetime
import inspect
from decimal import Decimal
from pydantic import Field
from rosetta.runtime.utils import (
BaseDataClass, rosetta_condition, rosetta_resolve_attr
)
from rosetta.runtime.utils import *
__all__ = ['ForwardPayout']
from cdm.product.common.settlement.PayoutBase import PayoutBase
class ForwardPayout(PayoutBase):
"""
Represents a forward settling payout. The underlier attribute captures the underlying payout, which is settled according to the settlementTerms attribute (which is part of PayoutBase). Both FX Spot and FX Forward should use this component.
"""
underlier: cdm.product.template.Product.Product = Field(..., description="Underlying product that the forward is written on, which can be of any type: FX, a contractual product, a security, etc.")
"""
Underlying product that the forward is written on, which can be of any type: FX, a contractual product, a security, etc.
"""
deliveryTerm: Optional[str] = Field(None, description="Also called contract month or delivery month. However, it's not always a month. It is usually expressed using a code, e.g. Z23 would be the Dec 2023 contract, (Z = December). For crude oil, the corresponding contract might be called CLZ23.")
"""
Also called contract month or delivery month. However, it's not always a month. It is usually expressed using a code, e.g. Z23 would be the Dec 2023 contract, (Z = December). For crude oil, the corresponding contract might be called CLZ23.
"""
delivery: Optional[cdm.product.asset.AssetDeliveryInformation.AssetDeliveryInformation] = Field(None, description="Contains the information relative to the delivery of the asset.")
"""
Contains the information relative to the delivery of the asset.
"""
schedule: Optional[cdm.product.template.CalculationSchedule.CalculationSchedule] = Field(None, description="Allows the full representation of a payout by defining a set of schedule periods. It supports standard schedule customization by expressing all the dates, quantities, and pricing data in a non-parametric way.")
"""
Allows the full representation of a payout by defining a set of schedule periods. It supports standard schedule customization by expressing all the dates, quantities, and pricing data in a non-parametric way.
"""
@rosetta_condition
def condition_0_SettlementTerms(self):
"""
For foreign exchange contracts, the settlement terms must exist.
"""
item = self
def _then_fn0():
return rosetta_attr_exists(rosetta_resolve_attr(self, "settlementTerms"))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "index"), "ForeignExchangeRate")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_1_SettlementDate(self):
"""
For foreign exchange contracts, either the settlementDate is set or the cashflowDates, but not both. When the cashflowDates are set, they must be the same for the 2 legs of the currency pair.
"""
item = self
def _then_fn0():
return (((rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "settlementTerms"), "settlementDate"), "valueDate")) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency1"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate")))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency2"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate")))) or ((((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "settlementTerms"), "settlementDate"), "valueDate"))) and rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency1"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate"))) and rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency2"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate"))) and all_elements(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency1"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate"), "=", rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange"), "exchangedCurrency2"), "settlementTerms"), "settlementDate"), "adjustableOrRelativeDate"))))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "index"), "ForeignExchangeRate")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_2_FxSettlement(self):
"""
For foreign exchange contracts, the settlement type must be either fx non-deliverable settlement or not specified, which implies physical settlement in the case of foreign exchange.
"""
item = self
def _then_fn0():
return (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(self, "settlementTerms"), "physicalSettlementTerms")))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(self, "underlier"), "foreignExchange")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_3_DeliveryCapacity(self):
"""
Checks that only one of the representations of delivery capacity is present simultaneously.
"""
item = self
def _then_fn3():
return (((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "deliveryCapacity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "deliveryCapacity")))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "deliveryCapacity"))))
def _else_fn3():
return True
def _then_fn2():
return ((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "deliveryCapacity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "deliveryCapacity"))))
def _else_fn2():
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "deliveryCapacity")), _then_fn3, _else_fn3)
def _then_fn1():
return (((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "deliveryCapacity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "deliveryCapacity")))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "deliveryCapacity"))))
def _else_fn1():
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "deliveryCapacity")), _then_fn2, _else_fn2)
def _then_fn0():
return (((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "deliveryCapacity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "deliveryCapacity")))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "deliveryCapacity"))))
def _else_fn0():
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "deliveryCapacity")), _then_fn1, _else_fn1)
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "deliveryCapacity")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_4_PriceTimeIntervalQuantity(self):
"""
Checks that only one of the representations of price time interval quantity is present simultaneously.
"""
item = self
def _then_fn2():
return ((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "priceTimeIntervalQuantity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "priceTimeIntervalQuantity"))))
def _else_fn2():
return True
def _then_fn1():
return ((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "priceTimeIntervalQuantity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "priceTimeIntervalQuantity"))))
def _else_fn1():
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "priceTimeIntervalQuantity")), _then_fn2, _else_fn2)
def _then_fn0():
return ((not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "priceTimeIntervalQuantity"))) and (not rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "profile"), "block"), "priceTimeIntervalQuantity"))))
def _else_fn0():
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "delivery"), "periods"), "profile"), "block"), "priceTimeIntervalQuantity")), _then_fn1, _else_fn1)
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(rosetta_resolve_attr(self, "schedule"), "schedulePeriod"), "deliveryPeriod"), "priceTimeIntervalQuantity")), _then_fn0, _else_fn0)
import cdm
import cdm.product.template.Product
import cdm.product.asset.AssetDeliveryInformation
import cdm.product.template.CalculationSchedule
© 2015 - 2025 Weber Informatics LLC | Privacy Policy