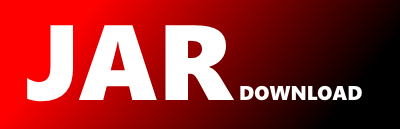
cdm.margin.schedule.functions.AuxiliarEffectiveDate Maven / Gradle / Ivy
package cdm.margin.schedule.functions;
import cdm.base.datetime.AdjustableDate;
import cdm.base.datetime.AdjustableOrRelativeDate;
import cdm.event.common.Trade;
import cdm.product.asset.InterestRatePayout;
import cdm.product.common.schedule.CalculationPeriodDates;
import cdm.product.template.EconomicTerms;
import cdm.product.template.NonTransferableProduct;
import cdm.product.template.OptionPayout;
import cdm.product.template.Payout;
import cdm.product.template.Product;
import cdm.product.template.Underlier;
import cdm.product.template.util.ProductDeepPathUtil;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperC;
import com.rosetta.model.lib.mapper.MapperS;
import com.rosetta.model.lib.records.Date;
import javax.inject.Inject;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(AuxiliarEffectiveDate.AuxiliarEffectiveDateDefault.class)
public abstract class AuxiliarEffectiveDate implements RosettaFunction {
// RosettaFunction dependencies
//
@Inject protected AdjustableDateResolution adjustableDateResolution;
@Inject protected ProductDeepPathUtil productDeepPathUtil;
/**
* @param trade
* @return effectiveDate
*/
public Date evaluate(Trade trade) {
Date effectiveDate = doEvaluate(trade);
return effectiveDate;
}
protected abstract Date doEvaluate(Trade trade);
protected abstract MapperS extends EconomicTerms> economicTerms(Trade trade);
public static class AuxiliarEffectiveDateDefault extends AuxiliarEffectiveDate {
@Override
protected Date doEvaluate(Trade trade) {
Date effectiveDate = null;
return assignOutput(effectiveDate, trade);
}
protected Date assignOutput(Date effectiveDate, Trade trade) {
if (exists(economicTerms(trade).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getInterestRatePayout", payout -> payout.getInterestRatePayout()).map("getCalculationPeriodDates", interestRatePayout -> interestRatePayout.getCalculationPeriodDates()).map("getEffectiveDate", calculationPeriodDates -> calculationPeriodDates.getEffectiveDate()).map("getAdjustableDate", adjustableOrRelativeDate -> adjustableOrRelativeDate.getAdjustableDate())).getOrDefault(false)) {
final MapperC thenResult0 = economicTerms(trade).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getInterestRatePayout", payout -> payout.getInterestRatePayout()).map("getCalculationPeriodDates", interestRatePayout -> interestRatePayout.getCalculationPeriodDates()).map("getEffectiveDate", calculationPeriodDates -> calculationPeriodDates.getEffectiveDate()).map("getAdjustableDate", adjustableOrRelativeDate -> adjustableOrRelativeDate.getAdjustableDate())
.mapItem(item -> MapperS.of(adjustableDateResolution.evaluate(item.get())));
effectiveDate = thenResult0
.min().get();
} else if (exists(MapperS.of(economicTerms(trade).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getOptionPayout", payout -> payout.getOptionPayout()).get()).map("getUnderlier", optionPayout -> optionPayout.getUnderlier()).map("getProduct", underlier -> underlier.getProduct()).map("chooseEconomicTerms", product -> productDeepPathUtil.chooseEconomicTerms(product)).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getInterestRatePayout", payout -> payout.getInterestRatePayout()).map("getCalculationPeriodDates", interestRatePayout -> interestRatePayout.getCalculationPeriodDates()).map("getEffectiveDate", calculationPeriodDates -> calculationPeriodDates.getEffectiveDate()).map("getAdjustableDate", adjustableOrRelativeDate -> adjustableOrRelativeDate.getAdjustableDate())).getOrDefault(false)) {
final MapperC thenResult1 = MapperS.of(economicTerms(trade).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getOptionPayout", payout -> payout.getOptionPayout()).get()).map("getUnderlier", optionPayout -> optionPayout.getUnderlier()).map("getProduct", underlier -> underlier.getProduct()).map("chooseEconomicTerms", product -> productDeepPathUtil.chooseEconomicTerms(product)).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getInterestRatePayout", payout -> payout.getInterestRatePayout()).map("getCalculationPeriodDates", interestRatePayout -> interestRatePayout.getCalculationPeriodDates()).map("getEffectiveDate", calculationPeriodDates -> calculationPeriodDates.getEffectiveDate()).map("getAdjustableDate", adjustableOrRelativeDate -> adjustableOrRelativeDate.getAdjustableDate())
.mapItem(item -> MapperS.of(adjustableDateResolution.evaluate(item.get())));
effectiveDate = thenResult1
.min().get();
} else {
effectiveDate = null;
}
return effectiveDate;
}
@Override
protected MapperS extends EconomicTerms> economicTerms(Trade trade) {
return MapperS.of(trade).map("getProduct", _trade -> _trade.getProduct()).map("getEconomicTerms", nonTransferableProduct -> nonTransferableProduct.getEconomicTerms());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy