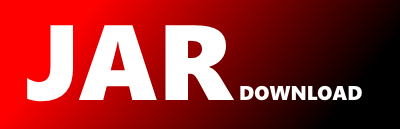
cdm.margin.schedule.functions.StandardizedScheduleCommodityForwardNotionalAmount Maven / Gradle / Ivy
package cdm.margin.schedule.functions;
import cdm.base.math.Measure;
import cdm.base.math.NonNegativeQuantitySchedule;
import cdm.base.math.metafields.ReferenceWithMetaNonNegativeQuantitySchedule;
import cdm.observable.asset.PriceSchedule;
import cdm.observable.asset.metafields.ReferenceWithMetaPriceSchedule;
import cdm.product.common.settlement.FixedPrice;
import cdm.product.common.settlement.ResolvablePriceQuantity;
import cdm.product.template.EconomicTerms;
import cdm.product.template.FixedPricePayout;
import cdm.product.template.Payout;
import cdm.product.template.SettlementPayout;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.expression.MapperMaths;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperS;
import java.math.BigDecimal;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(StandardizedScheduleCommodityForwardNotionalAmount.StandardizedScheduleCommodityForwardNotionalAmountDefault.class)
public abstract class StandardizedScheduleCommodityForwardNotionalAmount implements RosettaFunction {
/**
* @param economicTerms
* @return amount
*/
public BigDecimal evaluate(EconomicTerms economicTerms) {
BigDecimal amount = doEvaluate(economicTerms);
return amount;
}
protected abstract BigDecimal doEvaluate(EconomicTerms economicTerms);
protected abstract MapperS forwardPrice(EconomicTerms economicTerms);
protected abstract MapperS notionalQuantity(EconomicTerms economicTerms);
public static class StandardizedScheduleCommodityForwardNotionalAmountDefault extends StandardizedScheduleCommodityForwardNotionalAmount {
@Override
protected BigDecimal doEvaluate(EconomicTerms economicTerms) {
BigDecimal amount = null;
return assignOutput(amount, economicTerms);
}
protected BigDecimal assignOutput(BigDecimal amount, EconomicTerms economicTerms) {
amount = MapperMaths.multiply(forwardPrice(economicTerms), notionalQuantity(economicTerms)).get();
return amount;
}
@Override
protected MapperS forwardPrice(EconomicTerms economicTerms) {
return MapperS.of(MapperS.of(economicTerms).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getFixedPricePayout", payout -> payout.getFixedPricePayout()).get()).map("getFixedPrice", fixedPricePayout -> fixedPricePayout.getFixedPrice()).map("getPrice", fixedPrice -> fixedPrice.getPrice()).map("getValue", _f->_f.getValue()).map("getValue", priceSchedule -> priceSchedule.getValue());
}
@Override
protected MapperS notionalQuantity(EconomicTerms economicTerms) {
final MapperS thenResult = MapperS.of(MapperS.of(economicTerms).map("getPayout", _economicTerms -> _economicTerms.getPayout()).mapC("getSettlementPayout", payout -> payout.getSettlementPayout()).get()).map("getPriceQuantity", settlementPayout -> settlementPayout.getPriceQuantity()).map("getQuantitySchedule", resolvablePriceQuantity -> resolvablePriceQuantity.getQuantitySchedule()).map("getValue", _f->_f.getValue());
final MapperS ifThenElseResult;
if (exists(thenResult.map("getMultiplier", nonNegativeQuantitySchedule -> nonNegativeQuantitySchedule.getMultiplier())).getOrDefault(false)) {
ifThenElseResult = MapperMaths.multiply(thenResult.map("getValue", nonNegativeQuantitySchedule -> nonNegativeQuantitySchedule.getValue()), thenResult.map("getMultiplier", nonNegativeQuantitySchedule -> nonNegativeQuantitySchedule.getMultiplier()).map("getValue", measure -> measure.getValue()));
} else {
ifThenElseResult = thenResult.map("getValue", nonNegativeQuantitySchedule -> nonNegativeQuantitySchedule.getValue());
}
return ifThenElseResult;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy