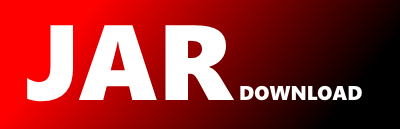
cdm.base.datetime.functions.ConvertToAdjustableOrRelativeDate Maven / Gradle / Ivy
package cdm.base.datetime.functions;
import cdm.base.datetime.AdjustableOrAdjustedOrRelativeDate;
import cdm.base.datetime.AdjustableOrRelativeDate;
import cdm.base.datetime.AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder;
import cdm.base.datetime.BusinessCenters;
import cdm.base.datetime.BusinessDayAdjustments;
import cdm.base.datetime.BusinessDayConventionEnum;
import cdm.base.datetime.DayTypeEnum;
import cdm.base.datetime.PeriodEnum;
import cdm.base.datetime.RelativeDateOffset;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperS;
import com.rosetta.model.lib.records.Date;
import com.rosetta.model.metafields.FieldWithMetaDate;
import com.rosetta.model.metafields.ReferenceWithMetaDate;
import java.util.Optional;
import javax.inject.Inject;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(ConvertToAdjustableOrRelativeDate.ConvertToAdjustableOrRelativeDateDefault.class)
public abstract class ConvertToAdjustableOrRelativeDate implements RosettaFunction {
@Inject protected ModelObjectValidator objectValidator;
/**
* @param adjustableOrAdjustedOrRelativeDate
* @return adjustableOrRelativeDate
*/
public AdjustableOrRelativeDate evaluate(AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate) {
AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder adjustableOrRelativeDateBuilder = doEvaluate(adjustableOrAdjustedOrRelativeDate);
final AdjustableOrRelativeDate adjustableOrRelativeDate;
if (adjustableOrRelativeDateBuilder == null) {
adjustableOrRelativeDate = null;
} else {
adjustableOrRelativeDate = adjustableOrRelativeDateBuilder.build();
objectValidator.validate(AdjustableOrRelativeDate.class, adjustableOrRelativeDate);
}
return adjustableOrRelativeDate;
}
protected abstract AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder doEvaluate(AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate);
protected abstract MapperS extends RelativeDateOffset> relativeDate(AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate);
public static class ConvertToAdjustableOrRelativeDateDefault extends ConvertToAdjustableOrRelativeDate {
@Override
protected AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder doEvaluate(AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate) {
AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder adjustableOrRelativeDate = AdjustableOrRelativeDate.builder();
return assignOutput(adjustableOrRelativeDate, adjustableOrAdjustedOrRelativeDate);
}
protected AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder assignOutput(AdjustableOrRelativeDate.AdjustableOrRelativeDateBuilder adjustableOrRelativeDate, AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate) {
final FieldWithMetaDate fieldWithMetaDate = MapperS.of(adjustableOrAdjustedOrRelativeDate).map("getAdjustedDate", _adjustableOrAdjustedOrRelativeDate -> _adjustableOrAdjustedOrRelativeDate.getAdjustedDate()).get();
adjustableOrRelativeDate
.getOrCreateAdjustableDate()
.setAdjustedDateValue((fieldWithMetaDate == null ? null : fieldWithMetaDate.getValue()));
adjustableOrRelativeDate
.getOrCreateAdjustableDate()
.setUnadjustedDate(MapperS.of(adjustableOrAdjustedOrRelativeDate).map("getUnadjustedDate", _adjustableOrAdjustedOrRelativeDate -> _adjustableOrAdjustedOrRelativeDate.getUnadjustedDate()).get());
adjustableOrRelativeDate
.getOrCreateAdjustableDate()
.setDateAdjustments(MapperS.of(adjustableOrAdjustedOrRelativeDate).map("getDateAdjustments", _adjustableOrAdjustedOrRelativeDate -> _adjustableOrAdjustedOrRelativeDate.getDateAdjustments()).get());
Date ifThenElseResult0 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult0 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getAdjustedDate", relativeDateOffset -> relativeDateOffset.getAdjustedDate()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setAdjustedDate(ifThenElseResult0);
BusinessCenters ifThenElseResult1 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult1 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getBusinessCenters", relativeDateOffset -> relativeDateOffset.getBusinessCenters()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setBusinessCenters(ifThenElseResult1);
BusinessDayConventionEnum ifThenElseResult2 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult2 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getBusinessDayConvention", relativeDateOffset -> relativeDateOffset.getBusinessDayConvention()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setBusinessDayConvention(ifThenElseResult2);
Date ifThenElseResult3 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
final ReferenceWithMetaDate referenceWithMetaDate = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getDateRelativeTo", relativeDateOffset -> relativeDateOffset.getDateRelativeTo()).get();
ifThenElseResult3 = referenceWithMetaDate == null ? null : referenceWithMetaDate.getValue();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setDateRelativeToValue(ifThenElseResult3);
DayTypeEnum ifThenElseResult4 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult4 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getDayType", relativeDateOffset -> relativeDateOffset.getDayType()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setDayType(ifThenElseResult4);
PeriodEnum ifThenElseResult5 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult5 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getPeriod", relativeDateOffset -> relativeDateOffset.getPeriod()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setPeriod(ifThenElseResult5);
Integer ifThenElseResult6 = null;
if (exists(relativeDate(adjustableOrAdjustedOrRelativeDate)).getOrDefault(false)) {
ifThenElseResult6 = relativeDate(adjustableOrAdjustedOrRelativeDate).map("getPeriodMultiplier", relativeDateOffset -> relativeDateOffset.getPeriodMultiplier()).get();
}
adjustableOrRelativeDate
.getOrCreateRelativeDate()
.setPeriodMultiplier(ifThenElseResult6);
return Optional.ofNullable(adjustableOrRelativeDate)
.map(o -> o.prune())
.orElse(null);
}
@Override
protected MapperS extends RelativeDateOffset> relativeDate(AdjustableOrAdjustedOrRelativeDate adjustableOrAdjustedOrRelativeDate) {
return MapperS.of(adjustableOrAdjustedOrRelativeDate).map("getRelativeDate", _adjustableOrAdjustedOrRelativeDate -> _adjustableOrAdjustedOrRelativeDate.getRelativeDate());
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy