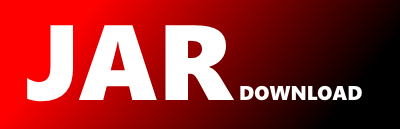
cdm.event.common.functions.Create_BusinessEvent Maven / Gradle / Ivy
package cdm.event.common.functions;
import cdm.event.common.BusinessEvent;
import cdm.event.common.BusinessEvent.BusinessEventBuilder;
import cdm.event.common.EventIntentEnum;
import cdm.event.common.ExerciseInstruction;
import cdm.event.common.Instruction;
import cdm.event.common.PrimitiveInstruction;
import cdm.event.common.SplitInstruction;
import cdm.event.common.TradeState;
import cdm.event.common.metafields.ReferenceWithMetaTradeState;
import com.google.inject.ImplementedBy;
import com.rosetta.model.lib.functions.ModelObjectValidator;
import com.rosetta.model.lib.functions.RosettaFunction;
import com.rosetta.model.lib.mapper.MapperC;
import com.rosetta.model.lib.mapper.MapperListOfLists;
import com.rosetta.model.lib.mapper.MapperS;
import com.rosetta.model.lib.records.Date;
import java.util.Collections;
import java.util.List;
import java.util.Optional;
import javax.inject.Inject;
import static com.rosetta.model.lib.expression.ExpressionOperators.*;
@ImplementedBy(Create_BusinessEvent.Create_BusinessEventDefault.class)
public abstract class Create_BusinessEvent implements RosettaFunction {
@Inject protected ModelObjectValidator objectValidator;
// RosettaFunction dependencies
//
@Inject protected Create_Exercise create_Exercise;
@Inject protected Create_Split create_Split;
@Inject protected Create_TradeState create_TradeState;
/**
* @param instruction
* @param intent
* @param eventDate
* @param effectiveDate
* @return businessEvent
*/
public BusinessEvent evaluate(List extends Instruction> instruction, EventIntentEnum intent, Date eventDate, Date effectiveDate) {
BusinessEvent.BusinessEventBuilder businessEventBuilder = doEvaluate(instruction, intent, eventDate, effectiveDate);
final BusinessEvent businessEvent;
if (businessEventBuilder == null) {
businessEvent = null;
} else {
businessEvent = businessEventBuilder.build();
objectValidator.validate(BusinessEvent.class, businessEvent);
}
return businessEvent;
}
protected abstract BusinessEvent.BusinessEventBuilder doEvaluate(List extends Instruction> instruction, EventIntentEnum intent, Date eventDate, Date effectiveDate);
public static class Create_BusinessEventDefault extends Create_BusinessEvent {
@Override
protected BusinessEvent.BusinessEventBuilder doEvaluate(List extends Instruction> instruction, EventIntentEnum intent, Date eventDate, Date effectiveDate) {
if (instruction == null) {
instruction = Collections.emptyList();
}
BusinessEvent.BusinessEventBuilder businessEvent = BusinessEvent.builder();
return assignOutput(businessEvent, instruction, intent, eventDate, effectiveDate);
}
protected BusinessEvent.BusinessEventBuilder assignOutput(BusinessEvent.BusinessEventBuilder businessEvent, List extends Instruction> instruction, EventIntentEnum intent, Date eventDate, Date effectiveDate) {
businessEvent
.addInstruction(instruction);
businessEvent
.setIntent(intent);
businessEvent
.setEventDate(eventDate);
businessEvent
.setEffectiveDate(effectiveDate);
final MapperListOfLists thenResult = MapperC.of(instruction)
.mapItemToList(item -> {
if (exists(item.map("getPrimitiveInstruction", _instruction -> _instruction.getPrimitiveInstruction()).map("getSplit", primitiveInstruction -> primitiveInstruction.getSplit())).getOrDefault(false)) {
final ReferenceWithMetaTradeState referenceWithMetaTradeState0 = item.map("getBefore", _instruction -> _instruction.getBefore()).get();
return MapperC.of(create_Split.evaluate(item.map("getPrimitiveInstruction", _instruction -> _instruction.getPrimitiveInstruction()).map("getSplit", primitiveInstruction -> primitiveInstruction.getSplit()).mapC("getBreakdown", splitInstruction -> splitInstruction.getBreakdown()).getMulti(), (referenceWithMetaTradeState0 == null ? null : referenceWithMetaTradeState0.getValue())));
}
if (exists(item.map("getPrimitiveInstruction", _instruction -> _instruction.getPrimitiveInstruction()).map("getExercise", primitiveInstruction -> primitiveInstruction.getExercise())).getOrDefault(false)) {
final ReferenceWithMetaTradeState referenceWithMetaTradeState1 = item.map("getBefore", _instruction -> _instruction.getBefore()).get();
return MapperC.of(create_Exercise.evaluate(item.map("getPrimitiveInstruction", _instruction -> _instruction.getPrimitiveInstruction()).map("getExercise", primitiveInstruction -> primitiveInstruction.getExercise()).get(), (referenceWithMetaTradeState1 == null ? null : referenceWithMetaTradeState1.getValue())));
}
final ReferenceWithMetaTradeState referenceWithMetaTradeState2 = item.map("getBefore", _instruction -> _instruction.getBefore()).get();
return MapperC.of(MapperS.of(create_TradeState.evaluate(item.map("getPrimitiveInstruction", _instruction -> _instruction.getPrimitiveInstruction()).get(), (referenceWithMetaTradeState2 == null ? null : referenceWithMetaTradeState2.getValue()))));
});
businessEvent
.addAfter(thenResult
.flattenList().getMulti());
return Optional.ofNullable(businessEvent)
.map(o -> o.prune())
.orElse(null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy