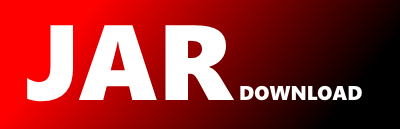
src.cdm.product.collateral.CollateralInterestCalculationParameters.py Maven / Gradle / Ivy
# pylint: disable=line-too-long, invalid-name, missing-function-docstring
# pylint: disable=bad-indentation, trailing-whitespace, superfluous-parens
# pylint: disable=wrong-import-position, unused-import, unused-wildcard-import
# pylint: disable=wildcard-import, wrong-import-order, missing-class-docstring
# pylint: disable=missing-module-docstring
from __future__ import annotations
from typing import List, Optional
import datetime
import inspect
from decimal import Decimal
from pydantic import Field
from rosetta.runtime.utils import (
BaseDataClass, rosetta_condition, rosetta_resolve_attr
)
from rosetta.runtime.utils import *
__all__ = ['CollateralInterestCalculationParameters']
class CollateralInterestCalculationParameters(BaseDataClass):
"""
Represents parameters for calculating the amount the floating interest calculation, e.g. for a single currency or defaults for all currencies.
"""
fixedRate: Optional[Decimal] = Field(None, description="Specifies the applicable fixed rate if used.")
"""
Specifies the applicable fixed rate if used.
"""
floatingRate: Optional[cdm.product.collateral.CollateralAgreementFloatingRate.CollateralAgreementFloatingRate] = Field(None, description="Specifies the floating interest rate to be used.")
"""
Specifies the floating interest rate to be used.
"""
inBaseCurrency: bool = Field(..., description="If True, specifies that the interest transfers should be converted to base currency equivalent, or if False specifies that the transfer should be in the currency of the collateral.")
"""
If True, specifies that the interest transfers should be converted to base currency equivalent, or if False specifies that the transfer should be in the currency of the collateral.
"""
compoundingType: Optional[cdm.base.datetime.CompoundingTypeEnum.CompoundingTypeEnum] = Field(None, description="Specifies the type of compounding to be applied (None, Business, Calendar).")
"""
Specifies the type of compounding to be applied (None, Business, Calendar).
"""
compoundingBusinessCenter: List[cdm.base.datetime.BusinessCenterEnum.BusinessCenterEnum] = Field([], description="Specifies the applicable business centers for compounding.")
"""
Specifies the applicable business centers for compounding.
"""
dayCountFraction: cdm.base.datetime.daycount.DayCountFractionEnum.DayCountFractionEnum = Field(..., description="Specifies the day count fraction to use for that currency.")
"""
Specifies the day count fraction to use for that currency.
"""
rounding: Optional[cdm.base.math.Rounding.Rounding] = Field(None, description="Specifies the rounding rules for settling in that currency.")
"""
Specifies the rounding rules for settling in that currency.
"""
roundingFrequency: Optional[cdm.base.datetime.RoundingFrequencyEnum.RoundingFrequencyEnum] = Field(None, description="Specifies when/how often is rounding applied?")
"""
Specifies when/how often is rounding applied?
"""
withholdingTaxRate: Optional[Decimal] = Field(None, description="Specifies the withholding tax rate if a withholding tax is applicable.")
"""
Specifies the withholding tax rate if a withholding tax is applicable.
"""
@rosetta_condition
def condition_0_InterestRate(self):
item = self
return self.check_one_of_constraint('fixedRate', 'floatingRate', necessity=True)
@rosetta_condition
def condition_1_DCF(self):
item = self
return (all_elements(rosetta_resolve_attr(self, "dayCountFraction"), "=", rosetta_resolve_attr(DayCountFractionEnum, "ACT_360")) or all_elements(rosetta_resolve_attr(self, "dayCountFraction"), "=", rosetta_resolve_attr(DayCountFractionEnum, "ACT_365_FIXED")))
@rosetta_condition
def condition_2_CompoundingBC1(self):
item = self
def _then_fn0():
return rosetta_attr_exists(rosetta_resolve_attr(self, "compoundingBusinessCenter"))
def _else_fn0():
return True
return if_cond_fn(all_elements(rosetta_resolve_attr(self, "compoundingType"), "=", rosetta_resolve_attr(CompoundingTypeEnum, "BUSINESS")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_3_CompoundingBC2(self):
item = self
def _then_fn0():
return (not rosetta_attr_exists(rosetta_resolve_attr(self, "compoundingBusinessCenter")))
def _else_fn0():
return True
return if_cond_fn(any_elements(rosetta_resolve_attr(self, "compoundingType"), "<>", rosetta_resolve_attr(CompoundingTypeEnum, "BUSINESS")), _then_fn0, _else_fn0)
import cdm
import cdm.product.collateral.CollateralAgreementFloatingRate
import cdm.base.datetime.CompoundingTypeEnum
import cdm.base.datetime.BusinessCenterEnum
import cdm.base.datetime.daycount.DayCountFractionEnum
import cdm.base.math.Rounding
import cdm.base.datetime.RoundingFrequencyEnum
from cdm.base.datetime.daycount.DayCountFractionEnum import DayCountFractionEnum
from cdm.base.datetime.CompoundingTypeEnum import CompoundingTypeEnum
© 2015 - 2025 Weber Informatics LLC | Privacy Policy