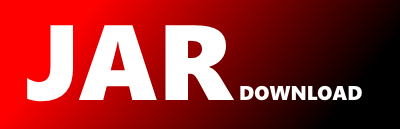
src.cdm.product.collateral.CollateralValuationTreatment.py Maven / Gradle / Ivy
# pylint: disable=line-too-long, invalid-name, missing-function-docstring
# pylint: disable=bad-indentation, trailing-whitespace, superfluous-parens
# pylint: disable=wrong-import-position, unused-import, unused-wildcard-import
# pylint: disable=wildcard-import, wrong-import-order, missing-class-docstring
# pylint: disable=missing-module-docstring
from __future__ import annotations
from typing import List, Optional
import datetime
import inspect
from decimal import Decimal
from pydantic import Field
from rosetta.runtime.utils import (
BaseDataClass, rosetta_condition, rosetta_resolve_attr
)
from rosetta.runtime.utils import *
__all__ = ['CollateralValuationTreatment']
class CollateralValuationTreatment(BaseDataClass):
"""
Specification of the valuation treatment for the specified collateral.
"""
haircutPercentage: Optional[Decimal] = Field(None, description="Specifies a haircut percentage to be applied to the value of asset and used as a discount factor to the value of the collateral asset,expressed as a percentage in decimal terms. As an example a 0.5% haircut would be represented as a decimal number 0.005.")
"""
Specifies a haircut percentage to be applied to the value of asset and used as a discount factor to the value of the collateral asset,expressed as a percentage in decimal terms. As an example a 0.5% haircut would be represented as a decimal number 0.005.
"""
marginPercentage: Optional[Decimal] = Field(None, description="Specifies a percentage value of transaction needing to be posted as collateral expressed as a valuation. As an example a 104% requirement would be represented as a decimal number 1.04.")
"""
Specifies a percentage value of transaction needing to be posted as collateral expressed as a valuation. As an example a 104% requirement would be represented as a decimal number 1.04.
"""
fxHaircutPercentage: Optional[Decimal] = Field(None, description="Specifies an FX haircut applied to a specific asset which is agreed between the parties (for example, if pledgor eligible collateral is not denominated in the termination currency or under other specified cases in collateral support documents both for initial margin and variation margin).The percentage value is expressed as the discount haircut to the value of the collateral- as an example an 8% FX haircut would be expressed as 0.08.")
"""
Specifies an FX haircut applied to a specific asset which is agreed between the parties (for example, if pledgor eligible collateral is not denominated in the termination currency or under other specified cases in collateral support documents both for initial margin and variation margin).The percentage value is expressed as the discount haircut to the value of the collateral- as an example an 8% FX haircut would be expressed as 0.08.
"""
additionalHaircutPercentage: Optional[Decimal] = Field(None, description="Specifies a percentage value of any additional haircut to be applied to a collateral asset,the percentage value is expressed as the discount haircut to the value of the collateral- as an example a 5% haircut would be expressed as 0.05. ")
"""
Specifies a percentage value of any additional haircut to be applied to a collateral asset,the percentage value is expressed as the discount haircut to the value of the collateral- as an example a 5% haircut would be expressed as 0.05.
"""
@rosetta_condition
def condition_0_HaircutPercentage(self):
"""
A data rule to validate that if a Valuation Percentage is specified it should be greater than or equal to 0 and less than 1.
"""
item = self
def _then_fn0():
return (all_elements(rosetta_resolve_attr(self, "haircutPercentage"), ">=", 0) and all_elements(rosetta_resolve_attr(self, "haircutPercentage"), "<", 1))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(self, "haircutPercentage")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_1_MarginPercentage(self):
"""
A data rule to validate that if a Margin Percentage is specified it should be greater than 1.
"""
item = self
def _then_fn0():
return all_elements(rosetta_resolve_attr(self, "marginPercentage"), ">=", 1)
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(self, "marginPercentage")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_2_FxHaircutPercentage(self):
"""
A data rule to validate that if an FX Haircut Percentage is specified it should be between 0 and less than 1.
"""
item = self
def _then_fn0():
return (all_elements(rosetta_resolve_attr(self, "fxHaircutPercentage"), ">", 0) and all_elements(rosetta_resolve_attr(self, "fxHaircutPercentage"), "<", 1))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(self, "fxHaircutPercentage")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_3_AdditionalHaircutPercentage(self):
"""
A data rule to validate that if an FX Haircut Percentage is specified it should be between 0 and less than 1.
"""
item = self
def _then_fn0():
return (all_elements(rosetta_resolve_attr(self, "additionalHaircutPercentage"), ">", 0) and all_elements(rosetta_resolve_attr(self, "additionalHaircutPercentage"), "<", 1))
def _else_fn0():
return True
return if_cond_fn(rosetta_attr_exists(rosetta_resolve_attr(self, "additionalHaircutPercentage")), _then_fn0, _else_fn0)
@rosetta_condition
def condition_4_HaircutPercentageOrMarginPercentage(self):
"""
Choice rule requiring that either a haircut percentage or margin percentage is specified.
"""
item = self
return self.check_one_of_constraint('haircutPercentage', 'marginPercentage', necessity=True)
import cdm
© 2015 - 2025 Weber Informatics LLC | Privacy Policy