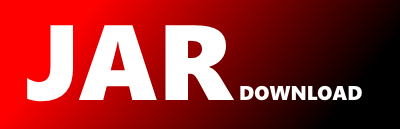
org.fpml.fpml_5.confirmation.CommodityBasketUnderlyingByNotional Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.04.04 at 04:56:21 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for CommodityBasketUnderlyingByNotional complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="CommodityBasketUnderlyingByNotional">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}CommodityBasketUnderlyingBase">
* <sequence>
* <element name="fx" type="{http://www.fpml.org/FpML-5/confirmation}CommodityFx" minOccurs="0"/>
* <element name="conversionFactor" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}CommodityNotionalQuantity.model"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "CommodityBasketUnderlyingByNotional", propOrder = {
"fx",
"conversionFactor",
"notionalQuantitySchedule",
"notionalQuantity",
"settlementPeriodsNotionalQuantity",
"totalNotionalQuantity",
"quantityReference"
})
public class CommodityBasketUnderlyingByNotional
extends CommodityBasketUnderlyingBase
{
protected CommodityFx fx;
protected BigDecimal conversionFactor;
protected CommodityNotionalQuantitySchedule notionalQuantitySchedule;
protected CommodityNotionalQuantity notionalQuantity;
protected List settlementPeriodsNotionalQuantity;
protected BigDecimal totalNotionalQuantity;
protected QuantityReference quantityReference;
/**
* Gets the value of the fx property.
*
* @return
* possible object is
* {@link CommodityFx }
*
*/
public CommodityFx getFx() {
return fx;
}
/**
* Sets the value of the fx property.
*
* @param value
* allowed object is
* {@link CommodityFx }
*
*/
public void setFx(CommodityFx value) {
this.fx = value;
}
/**
* Gets the value of the conversionFactor property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getConversionFactor() {
return conversionFactor;
}
/**
* Sets the value of the conversionFactor property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setConversionFactor(BigDecimal value) {
this.conversionFactor = value;
}
/**
* Gets the value of the notionalQuantitySchedule property.
*
* @return
* possible object is
* {@link CommodityNotionalQuantitySchedule }
*
*/
public CommodityNotionalQuantitySchedule getNotionalQuantitySchedule() {
return notionalQuantitySchedule;
}
/**
* Sets the value of the notionalQuantitySchedule property.
*
* @param value
* allowed object is
* {@link CommodityNotionalQuantitySchedule }
*
*/
public void setNotionalQuantitySchedule(CommodityNotionalQuantitySchedule value) {
this.notionalQuantitySchedule = value;
}
/**
* Gets the value of the notionalQuantity property.
*
* @return
* possible object is
* {@link CommodityNotionalQuantity }
*
*/
public CommodityNotionalQuantity getNotionalQuantity() {
return notionalQuantity;
}
/**
* Sets the value of the notionalQuantity property.
*
* @param value
* allowed object is
* {@link CommodityNotionalQuantity }
*
*/
public void setNotionalQuantity(CommodityNotionalQuantity value) {
this.notionalQuantity = value;
}
/**
* Gets the value of the settlementPeriodsNotionalQuantity property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the settlementPeriodsNotionalQuantity property.
*
*
* For example, to add a new item, do as follows:
*
* getSettlementPeriodsNotionalQuantity().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CommoditySettlementPeriodsNotionalQuantity }
*
*
*/
public List getSettlementPeriodsNotionalQuantity() {
if (settlementPeriodsNotionalQuantity == null) {
settlementPeriodsNotionalQuantity = new ArrayList();
}
return this.settlementPeriodsNotionalQuantity;
}
/**
* Gets the value of the totalNotionalQuantity property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getTotalNotionalQuantity() {
return totalNotionalQuantity;
}
/**
* Sets the value of the totalNotionalQuantity property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setTotalNotionalQuantity(BigDecimal value) {
this.totalNotionalQuantity = value;
}
/**
* Gets the value of the quantityReference property.
*
* @return
* possible object is
* {@link QuantityReference }
*
*/
public QuantityReference getQuantityReference() {
return quantityReference;
}
/**
* Sets the value of the quantityReference property.
*
* @param value
* allowed object is
* {@link QuantityReference }
*
*/
public void setQuantityReference(QuantityReference value) {
this.quantityReference = value;
}
}