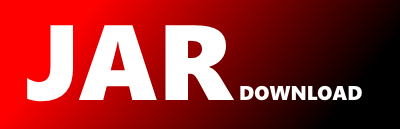
org.fpml.fpml_5.confirmation.FxFlexibleForward Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.04.04 at 04:56:21 PM UTC
//
package org.fpml.fpml_5.confirmation;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* Product model for a flexible-term fx forward (also known as callable forward, window forward). This is a term forward transaction over a specific period, allowing the client full flexibility on the timing of the transactional flow(s). The product allows for (full or partial) execution at a predetermined forward rate, at any time between the start date and the expiry date. Although, the product is an outright, it has some option-like characteristics, leading to the use of option components in the model: (i) the BuyerSeller model expresses the roles of the parties in the overall transaction - the client "buys" the product (ii) the PutCallCurrency model expresses the buyer's perspective on the exchanged currencies i.e. the client may buy (call) or sell (put) the notional currency for the alternative currency.
*
* Java class for FxFlexibleForward complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FxFlexibleForward">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}Product">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}BuyerSeller.model"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}PutCallCurrency.model"/>
* <sequence>
* <element name="notionalAmount" type="{http://www.fpml.org/FpML-5/confirmation}PositiveMoney"/>
* <element name="minimumExecutionAmount" type="{http://www.fpml.org/FpML-5/confirmation}PositiveMoney" minOccurs="0"/>
* <element name="settlementAmount" type="{http://www.fpml.org/FpML-5/confirmation}NonNegativeMoney" minOccurs="0"/>
* </sequence>
* <element name="executionPeriodDates" type="{http://www.fpml.org/FpML-5/confirmation}FxFlexibleForwardExecutionPeriod"/>
* <sequence minOccurs="0">
* <element name="earliestExecutionTime" type="{http://www.fpml.org/FpML-5/confirmation}BusinessCenterTime"/>
* <element name="latestExecutionTime" type="{http://www.fpml.org/FpML-5/confirmation}BusinessCenterTime"/>
* </sequence>
* <element name="settlementDateOffset" type="{http://www.fpml.org/FpML-5/confirmation}RelativeDateOffset" minOccurs="0"/>
* <element name="finalSettlementDate" type="{http://www.w3.org/2001/XMLSchema}date" minOccurs="0"/>
* <element name="forwardRate" type="{http://www.fpml.org/FpML-5/confirmation}FxFlexibleForwardRate"/>
* <element name="additionalPayment" type="{http://www.fpml.org/FpML-5/confirmation}Payment" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FxFlexibleForward", propOrder = {
"buyerPartyReference",
"buyerAccountReference",
"sellerPartyReference",
"sellerAccountReference",
"putCurrency",
"callCurrency",
"notionalAmount",
"minimumExecutionAmount",
"settlementAmount",
"executionPeriodDates",
"earliestExecutionTime",
"latestExecutionTime",
"settlementDateOffset",
"finalSettlementDate",
"forwardRate",
"additionalPayment"
})
public class FxFlexibleForward
extends Product
{
@XmlElement(required = true)
protected PartyReference buyerPartyReference;
protected AccountReference buyerAccountReference;
@XmlElement(required = true)
protected PartyReference sellerPartyReference;
protected AccountReference sellerAccountReference;
@XmlElement(required = true)
protected Currency putCurrency;
@XmlElement(required = true)
protected Currency callCurrency;
@XmlElement(required = true)
protected PositiveMoney notionalAmount;
protected PositiveMoney minimumExecutionAmount;
protected NonNegativeMoney settlementAmount;
@XmlElement(required = true)
protected FxFlexibleForwardExecutionPeriod executionPeriodDates;
protected BusinessCenterTime earliestExecutionTime;
protected BusinessCenterTime latestExecutionTime;
protected RelativeDateOffset settlementDateOffset;
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar finalSettlementDate;
@XmlElement(required = true)
protected FxFlexibleForwardRate forwardRate;
protected Payment additionalPayment;
/**
* Gets the value of the buyerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBuyerPartyReference() {
return buyerPartyReference;
}
/**
* Sets the value of the buyerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBuyerPartyReference(PartyReference value) {
this.buyerPartyReference = value;
}
/**
* Gets the value of the buyerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getBuyerAccountReference() {
return buyerAccountReference;
}
/**
* Sets the value of the buyerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setBuyerAccountReference(AccountReference value) {
this.buyerAccountReference = value;
}
/**
* Gets the value of the sellerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getSellerPartyReference() {
return sellerPartyReference;
}
/**
* Sets the value of the sellerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setSellerPartyReference(PartyReference value) {
this.sellerPartyReference = value;
}
/**
* Gets the value of the sellerAccountReference property.
*
* @return
* possible object is
* {@link AccountReference }
*
*/
public AccountReference getSellerAccountReference() {
return sellerAccountReference;
}
/**
* Sets the value of the sellerAccountReference property.
*
* @param value
* allowed object is
* {@link AccountReference }
*
*/
public void setSellerAccountReference(AccountReference value) {
this.sellerAccountReference = value;
}
/**
* Gets the value of the putCurrency property.
*
* @return
* possible object is
* {@link Currency }
*
*/
public Currency getPutCurrency() {
return putCurrency;
}
/**
* Sets the value of the putCurrency property.
*
* @param value
* allowed object is
* {@link Currency }
*
*/
public void setPutCurrency(Currency value) {
this.putCurrency = value;
}
/**
* Gets the value of the callCurrency property.
*
* @return
* possible object is
* {@link Currency }
*
*/
public Currency getCallCurrency() {
return callCurrency;
}
/**
* Sets the value of the callCurrency property.
*
* @param value
* allowed object is
* {@link Currency }
*
*/
public void setCallCurrency(Currency value) {
this.callCurrency = value;
}
/**
* Gets the value of the notionalAmount property.
*
* @return
* possible object is
* {@link PositiveMoney }
*
*/
public PositiveMoney getNotionalAmount() {
return notionalAmount;
}
/**
* Sets the value of the notionalAmount property.
*
* @param value
* allowed object is
* {@link PositiveMoney }
*
*/
public void setNotionalAmount(PositiveMoney value) {
this.notionalAmount = value;
}
/**
* Gets the value of the minimumExecutionAmount property.
*
* @return
* possible object is
* {@link PositiveMoney }
*
*/
public PositiveMoney getMinimumExecutionAmount() {
return minimumExecutionAmount;
}
/**
* Sets the value of the minimumExecutionAmount property.
*
* @param value
* allowed object is
* {@link PositiveMoney }
*
*/
public void setMinimumExecutionAmount(PositiveMoney value) {
this.minimumExecutionAmount = value;
}
/**
* Gets the value of the settlementAmount property.
*
* @return
* possible object is
* {@link NonNegativeMoney }
*
*/
public NonNegativeMoney getSettlementAmount() {
return settlementAmount;
}
/**
* Sets the value of the settlementAmount property.
*
* @param value
* allowed object is
* {@link NonNegativeMoney }
*
*/
public void setSettlementAmount(NonNegativeMoney value) {
this.settlementAmount = value;
}
/**
* Gets the value of the executionPeriodDates property.
*
* @return
* possible object is
* {@link FxFlexibleForwardExecutionPeriod }
*
*/
public FxFlexibleForwardExecutionPeriod getExecutionPeriodDates() {
return executionPeriodDates;
}
/**
* Sets the value of the executionPeriodDates property.
*
* @param value
* allowed object is
* {@link FxFlexibleForwardExecutionPeriod }
*
*/
public void setExecutionPeriodDates(FxFlexibleForwardExecutionPeriod value) {
this.executionPeriodDates = value;
}
/**
* Gets the value of the earliestExecutionTime property.
*
* @return
* possible object is
* {@link BusinessCenterTime }
*
*/
public BusinessCenterTime getEarliestExecutionTime() {
return earliestExecutionTime;
}
/**
* Sets the value of the earliestExecutionTime property.
*
* @param value
* allowed object is
* {@link BusinessCenterTime }
*
*/
public void setEarliestExecutionTime(BusinessCenterTime value) {
this.earliestExecutionTime = value;
}
/**
* Gets the value of the latestExecutionTime property.
*
* @return
* possible object is
* {@link BusinessCenterTime }
*
*/
public BusinessCenterTime getLatestExecutionTime() {
return latestExecutionTime;
}
/**
* Sets the value of the latestExecutionTime property.
*
* @param value
* allowed object is
* {@link BusinessCenterTime }
*
*/
public void setLatestExecutionTime(BusinessCenterTime value) {
this.latestExecutionTime = value;
}
/**
* Gets the value of the settlementDateOffset property.
*
* @return
* possible object is
* {@link RelativeDateOffset }
*
*/
public RelativeDateOffset getSettlementDateOffset() {
return settlementDateOffset;
}
/**
* Sets the value of the settlementDateOffset property.
*
* @param value
* allowed object is
* {@link RelativeDateOffset }
*
*/
public void setSettlementDateOffset(RelativeDateOffset value) {
this.settlementDateOffset = value;
}
/**
* Gets the value of the finalSettlementDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getFinalSettlementDate() {
return finalSettlementDate;
}
/**
* Sets the value of the finalSettlementDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setFinalSettlementDate(XMLGregorianCalendar value) {
this.finalSettlementDate = value;
}
/**
* Gets the value of the forwardRate property.
*
* @return
* possible object is
* {@link FxFlexibleForwardRate }
*
*/
public FxFlexibleForwardRate getForwardRate() {
return forwardRate;
}
/**
* Sets the value of the forwardRate property.
*
* @param value
* allowed object is
* {@link FxFlexibleForwardRate }
*
*/
public void setForwardRate(FxFlexibleForwardRate value) {
this.forwardRate = value;
}
/**
* Gets the value of the additionalPayment property.
*
* @return
* possible object is
* {@link Payment }
*
*/
public Payment getAdditionalPayment() {
return additionalPayment;
}
/**
* Sets the value of the additionalPayment property.
*
* @param value
* allowed object is
* {@link Payment }
*
*/
public void setAdditionalPayment(Payment value) {
this.additionalPayment = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy