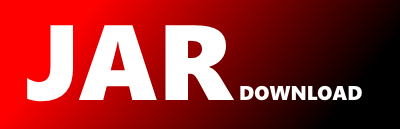
org.fpml.fpml_5.confirmation.Party Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.04.04 at 04:56:21 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlID;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.CollapsedStringAdapter;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
/**
* Parties can perform multiple roles in a trade lifecycle. For example, the principal parties obligated to make payments from time to time during the term of the trade, but may include other parties involved in, or incidental to, the trade, such as parties acting in the role of novation transferor/transferee, broker, calculation agent, etc. In FpML roles are defined in multiple places within a document.
*
* Java class for Party complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Party">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <choice>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}Party.model"/>
* <sequence>
* <element name="groupType" type="{http://www.fpml.org/FpML-5/confirmation}PartyGroupType"/>
* <element name="partyReference" type="{http://www.fpml.org/FpML-5/confirmation}PartyReference" maxOccurs="unbounded" minOccurs="2"/>
* </sequence>
* </choice>
* <attribute name="id" use="required" type="{http://www.w3.org/2001/XMLSchema}ID" />
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Party", propOrder = {
"partyId",
"partyName",
"classification",
"creditRating",
"country",
"region",
"jurisdiction",
"organizationType",
"contactInfo",
"businessUnit",
"person",
"groupType",
"partyReference"
})
public class Party {
protected List partyId;
protected PartyName partyName;
protected List classification;
protected List creditRating;
protected CountryCode country;
protected List region;
protected List jurisdiction;
protected OrganizationType organizationType;
protected ContactInformation contactInfo;
protected List businessUnit;
protected List person;
protected PartyGroupType groupType;
protected List partyReference;
@XmlAttribute(name = "id", required = true)
@XmlJavaTypeAdapter(CollapsedStringAdapter.class)
@XmlID
@XmlSchemaType(name = "ID")
protected String id;
/**
* Gets the value of the partyId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the partyId property.
*
*
* For example, to add a new item, do as follows:
*
* getPartyId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyId }
*
*
*/
public List getPartyId() {
if (partyId == null) {
partyId = new ArrayList();
}
return this.partyId;
}
/**
* Gets the value of the partyName property.
*
* @return
* possible object is
* {@link PartyName }
*
*/
public PartyName getPartyName() {
return partyName;
}
/**
* Sets the value of the partyName property.
*
* @param value
* allowed object is
* {@link PartyName }
*
*/
public void setPartyName(PartyName value) {
this.partyName = value;
}
/**
* Gets the value of the classification property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the classification property.
*
*
* For example, to add a new item, do as follows:
*
* getClassification().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link IndustryClassification }
*
*
*/
public List getClassification() {
if (classification == null) {
classification = new ArrayList();
}
return this.classification;
}
/**
* Gets the value of the creditRating property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the creditRating property.
*
*
* For example, to add a new item, do as follows:
*
* getCreditRating().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CreditRating }
*
*
*/
public List getCreditRating() {
if (creditRating == null) {
creditRating = new ArrayList();
}
return this.creditRating;
}
/**
* Gets the value of the country property.
*
* @return
* possible object is
* {@link CountryCode }
*
*/
public CountryCode getCountry() {
return country;
}
/**
* Sets the value of the country property.
*
* @param value
* allowed object is
* {@link CountryCode }
*
*/
public void setCountry(CountryCode value) {
this.country = value;
}
/**
* Gets the value of the region property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the region property.
*
*
* For example, to add a new item, do as follows:
*
* getRegion().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Region }
*
*
*/
public List getRegion() {
if (region == null) {
region = new ArrayList();
}
return this.region;
}
/**
* Gets the value of the jurisdiction property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the jurisdiction property.
*
*
* For example, to add a new item, do as follows:
*
* getJurisdiction().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link GoverningLaw }
*
*
*/
public List getJurisdiction() {
if (jurisdiction == null) {
jurisdiction = new ArrayList();
}
return this.jurisdiction;
}
/**
* Gets the value of the organizationType property.
*
* @return
* possible object is
* {@link OrganizationType }
*
*/
public OrganizationType getOrganizationType() {
return organizationType;
}
/**
* Sets the value of the organizationType property.
*
* @param value
* allowed object is
* {@link OrganizationType }
*
*/
public void setOrganizationType(OrganizationType value) {
this.organizationType = value;
}
/**
* Gets the value of the contactInfo property.
*
* @return
* possible object is
* {@link ContactInformation }
*
*/
public ContactInformation getContactInfo() {
return contactInfo;
}
/**
* Sets the value of the contactInfo property.
*
* @param value
* allowed object is
* {@link ContactInformation }
*
*/
public void setContactInfo(ContactInformation value) {
this.contactInfo = value;
}
/**
* Gets the value of the businessUnit property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the businessUnit property.
*
*
* For example, to add a new item, do as follows:
*
* getBusinessUnit().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BusinessUnit }
*
*
*/
public List getBusinessUnit() {
if (businessUnit == null) {
businessUnit = new ArrayList();
}
return this.businessUnit;
}
/**
* Gets the value of the person property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the person property.
*
*
* For example, to add a new item, do as follows:
*
* getPerson().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Person }
*
*
*/
public List getPerson() {
if (person == null) {
person = new ArrayList();
}
return this.person;
}
/**
* Gets the value of the groupType property.
*
* @return
* possible object is
* {@link PartyGroupType }
*
*/
public PartyGroupType getGroupType() {
return groupType;
}
/**
* Sets the value of the groupType property.
*
* @param value
* allowed object is
* {@link PartyGroupType }
*
*/
public void setGroupType(PartyGroupType value) {
this.groupType = value;
}
/**
* Gets the value of the partyReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the partyReference property.
*
*
* For example, to add a new item, do as follows:
*
* getPartyReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyReference }
*
*
*/
public List getPartyReference() {
if (partyReference == null) {
partyReference = new ArrayList();
}
return this.partyReference;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setId(String value) {
this.id = value;
}
}