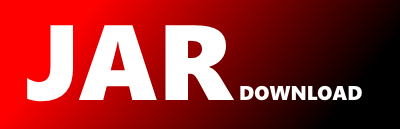
org.fpml.fpml_5.confirmation.RepoFarLeg Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.04.04 at 04:56:21 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* A transaction leg for a repo is equivalent to a single cash transaction. It is augmented here to carry some values that are of interest for the repo. Also note that the BuyerSeller model in this transaction must be the exact opposite of the one found in the near leg.
*
* Java class for RepoFarLeg complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="RepoFarLeg">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}RepoLegBase">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}SettlementAmountOrCurrency.model"/>
* <element name="fxRate" type="{http://www.fpml.org/FpML-5/confirmation}FxRate" minOccurs="0"/>
* <sequence minOccurs="0">
* <element name="deliveryMethod" type="{http://www.fpml.org/FpML-5/confirmation}DeliveryMethod"/>
* <element name="deliveryDate" type="{http://www.fpml.org/FpML-5/confirmation}AdjustableOrRelativeDate" minOccurs="0"/>
* <element name="collateral" type="{http://www.fpml.org/FpML-5/confirmation}CollateralValuation" maxOccurs="unbounded"/>
* </sequence>
* <element name="repoInterest" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "RepoFarLeg", propOrder = {
"settlementAmount",
"settlementCurrency",
"fxRate",
"deliveryMethod",
"deliveryDate",
"collateral",
"repoInterest"
})
public class RepoFarLeg
extends RepoLegBase
{
protected Money settlementAmount;
protected Currency settlementCurrency;
protected FxRate fxRate;
protected DeliveryMethod deliveryMethod;
protected AdjustableOrRelativeDate deliveryDate;
protected List collateral;
protected BigDecimal repoInterest;
/**
* Gets the value of the settlementAmount property.
*
* @return
* possible object is
* {@link Money }
*
*/
public Money getSettlementAmount() {
return settlementAmount;
}
/**
* Sets the value of the settlementAmount property.
*
* @param value
* allowed object is
* {@link Money }
*
*/
public void setSettlementAmount(Money value) {
this.settlementAmount = value;
}
/**
* Gets the value of the settlementCurrency property.
*
* @return
* possible object is
* {@link Currency }
*
*/
public Currency getSettlementCurrency() {
return settlementCurrency;
}
/**
* Sets the value of the settlementCurrency property.
*
* @param value
* allowed object is
* {@link Currency }
*
*/
public void setSettlementCurrency(Currency value) {
this.settlementCurrency = value;
}
/**
* Gets the value of the fxRate property.
*
* @return
* possible object is
* {@link FxRate }
*
*/
public FxRate getFxRate() {
return fxRate;
}
/**
* Sets the value of the fxRate property.
*
* @param value
* allowed object is
* {@link FxRate }
*
*/
public void setFxRate(FxRate value) {
this.fxRate = value;
}
/**
* Gets the value of the deliveryMethod property.
*
* @return
* possible object is
* {@link DeliveryMethod }
*
*/
public DeliveryMethod getDeliveryMethod() {
return deliveryMethod;
}
/**
* Sets the value of the deliveryMethod property.
*
* @param value
* allowed object is
* {@link DeliveryMethod }
*
*/
public void setDeliveryMethod(DeliveryMethod value) {
this.deliveryMethod = value;
}
/**
* Gets the value of the deliveryDate property.
*
* @return
* possible object is
* {@link AdjustableOrRelativeDate }
*
*/
public AdjustableOrRelativeDate getDeliveryDate() {
return deliveryDate;
}
/**
* Sets the value of the deliveryDate property.
*
* @param value
* allowed object is
* {@link AdjustableOrRelativeDate }
*
*/
public void setDeliveryDate(AdjustableOrRelativeDate value) {
this.deliveryDate = value;
}
/**
* Gets the value of the collateral property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the collateral property.
*
*
* For example, to add a new item, do as follows:
*
* getCollateral().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link CollateralValuation }
*
*
*/
public List getCollateral() {
if (collateral == null) {
collateral = new ArrayList();
}
return this.collateral;
}
/**
* Gets the value of the repoInterest property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getRepoInterest() {
return repoInterest;
}
/**
* Sets the value of the repoInterest property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setRepoInterest(BigDecimal value) {
this.repoInterest = value;
}
}