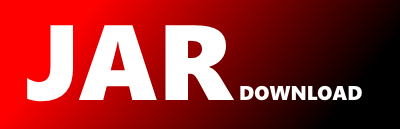
org.fpml.fpml_5.confirmation.Calculation Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.05.10 at 03:58:40 PM UTC
//
package org.fpml.fpml_5.confirmation;
import javax.xml.bind.JAXBElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlElementRef;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* A type definining the parameters used in the calculation of fixed or floating calculation period amounts.
*
* Java class for Calculation complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Calculation">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <choice>
* <element name="notionalSchedule" type="{http://www.fpml.org/FpML-5/confirmation}Notional"/>
* <element name="fxLinkedNotionalSchedule" type="{http://www.fpml.org/FpML-5/confirmation}FxLinkedNotionalSchedule"/>
* </choice>
* <choice>
* <sequence>
* <element name="fixedRateSchedule" type="{http://www.fpml.org/FpML-5/confirmation}Schedule"/>
* <element name="futureValueNotional" type="{http://www.fpml.org/FpML-5/confirmation}FutureValueAmount" minOccurs="0"/>
* </sequence>
* <element ref="{http://www.fpml.org/FpML-5/confirmation}rateCalculation"/>
* </choice>
* <element name="dayCountFraction" type="{http://www.fpml.org/FpML-5/confirmation}DayCountFraction"/>
* <element name="discounting" type="{http://www.fpml.org/FpML-5/confirmation}Discounting" minOccurs="0"/>
* <element name="compoundingMethod" type="{http://www.fpml.org/FpML-5/confirmation}CompoundingMethodEnum" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Calculation", propOrder = {
"notionalSchedule",
"fxLinkedNotionalSchedule",
"fixedRateSchedule",
"futureValueNotional",
"rateCalculation",
"dayCountFraction",
"discounting",
"compoundingMethod"
})
public class Calculation {
protected Notional notionalSchedule;
protected FxLinkedNotionalSchedule fxLinkedNotionalSchedule;
protected Schedule fixedRateSchedule;
protected FutureValueAmount futureValueNotional;
@XmlElementRef(name = "rateCalculation", namespace = "http://www.fpml.org/FpML-5/confirmation", type = JAXBElement.class, required = false)
protected JAXBElement extends Rate> rateCalculation;
@XmlElement(required = true)
protected DayCountFraction dayCountFraction;
protected Discounting discounting;
@XmlSchemaType(name = "token")
protected CompoundingMethodEnum compoundingMethod;
/**
* Gets the value of the notionalSchedule property.
*
* @return
* possible object is
* {@link Notional }
*
*/
public Notional getNotionalSchedule() {
return notionalSchedule;
}
/**
* Sets the value of the notionalSchedule property.
*
* @param value
* allowed object is
* {@link Notional }
*
*/
public void setNotionalSchedule(Notional value) {
this.notionalSchedule = value;
}
/**
* Gets the value of the fxLinkedNotionalSchedule property.
*
* @return
* possible object is
* {@link FxLinkedNotionalSchedule }
*
*/
public FxLinkedNotionalSchedule getFxLinkedNotionalSchedule() {
return fxLinkedNotionalSchedule;
}
/**
* Sets the value of the fxLinkedNotionalSchedule property.
*
* @param value
* allowed object is
* {@link FxLinkedNotionalSchedule }
*
*/
public void setFxLinkedNotionalSchedule(FxLinkedNotionalSchedule value) {
this.fxLinkedNotionalSchedule = value;
}
/**
* Gets the value of the fixedRateSchedule property.
*
* @return
* possible object is
* {@link Schedule }
*
*/
public Schedule getFixedRateSchedule() {
return fixedRateSchedule;
}
/**
* Sets the value of the fixedRateSchedule property.
*
* @param value
* allowed object is
* {@link Schedule }
*
*/
public void setFixedRateSchedule(Schedule value) {
this.fixedRateSchedule = value;
}
/**
* Gets the value of the futureValueNotional property.
*
* @return
* possible object is
* {@link FutureValueAmount }
*
*/
public FutureValueAmount getFutureValueNotional() {
return futureValueNotional;
}
/**
* Sets the value of the futureValueNotional property.
*
* @param value
* allowed object is
* {@link FutureValueAmount }
*
*/
public void setFutureValueNotional(FutureValueAmount value) {
this.futureValueNotional = value;
}
/**
* This element is the head of a substitution group. It is substituted by the floatingRateCalculation element for standard Floating Rate legs, or the inflationRateCalculation element for inflation swaps.
*
* @return
* possible object is
* {@link JAXBElement }{@code <}{@link FloatingRateCalculation }{@code >}
* {@link JAXBElement }{@code <}{@link InflationRateCalculation }{@code >}
* {@link JAXBElement }{@code <}{@link Rate }{@code >}
*
*/
public JAXBElement extends Rate> getRateCalculation() {
return rateCalculation;
}
/**
* Sets the value of the rateCalculation property.
*
* @param value
* allowed object is
* {@link JAXBElement }{@code <}{@link FloatingRateCalculation }{@code >}
* {@link JAXBElement }{@code <}{@link InflationRateCalculation }{@code >}
* {@link JAXBElement }{@code <}{@link Rate }{@code >}
*
*/
public void setRateCalculation(JAXBElement extends Rate> value) {
this.rateCalculation = value;
}
/**
* Gets the value of the dayCountFraction property.
*
* @return
* possible object is
* {@link DayCountFraction }
*
*/
public DayCountFraction getDayCountFraction() {
return dayCountFraction;
}
/**
* Sets the value of the dayCountFraction property.
*
* @param value
* allowed object is
* {@link DayCountFraction }
*
*/
public void setDayCountFraction(DayCountFraction value) {
this.dayCountFraction = value;
}
/**
* Gets the value of the discounting property.
*
* @return
* possible object is
* {@link Discounting }
*
*/
public Discounting getDiscounting() {
return discounting;
}
/**
* Sets the value of the discounting property.
*
* @param value
* allowed object is
* {@link Discounting }
*
*/
public void setDiscounting(Discounting value) {
this.discounting = value;
}
/**
* Gets the value of the compoundingMethod property.
*
* @return
* possible object is
* {@link CompoundingMethodEnum }
*
*/
public CompoundingMethodEnum getCompoundingMethod() {
return compoundingMethod;
}
/**
* Sets the value of the compoundingMethod property.
*
* @param value
* allowed object is
* {@link CompoundingMethodEnum }
*
*/
public void setCompoundingMethod(CompoundingMethodEnum value) {
this.compoundingMethod = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy