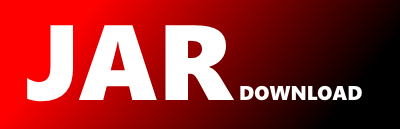
org.fpml.fpml_5.confirmation.DividendSwapOptionTransactionSupplement Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.05.10 at 03:58:40 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.math.BigDecimal;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Java class for DividendSwapOptionTransactionSupplement complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="DividendSwapOptionTransactionSupplement">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}OptionBase">
* <sequence>
* <element name="equityPremium" type="{http://www.fpml.org/FpML-5/confirmation}EquityPremium"/>
* <element name="equityExercise" type="{http://www.fpml.org/FpML-5/confirmation}EquityExerciseValuationSettlement"/>
* <element name="exchangeLookAlike" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="methodOfAdjustment" type="{http://www.fpml.org/FpML-5/confirmation}MethodOfAdjustmentEnum" minOccurs="0"/>
* <choice minOccurs="0">
* <element name="optionEntitlement" type="{http://www.fpml.org/FpML-5/confirmation}PositiveDecimal"/>
* <element name="multiplier" type="{http://www.fpml.org/FpML-5/confirmation}PositiveDecimal"/>
* </choice>
* <element name="clearingInstructions" type="{http://www.fpml.org/FpML-5/confirmation}SwaptionPhysicalSettlement" minOccurs="0"/>
* <element name="dividendSwapTransactionSupplement" type="{http://www.fpml.org/FpML-5/confirmation}DividendSwapTransactionSupplement"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "DividendSwapOptionTransactionSupplement", propOrder = {
"equityPremium",
"equityExercise",
"exchangeLookAlike",
"methodOfAdjustment",
"optionEntitlement",
"multiplier",
"clearingInstructions",
"dividendSwapTransactionSupplement"
})
public class DividendSwapOptionTransactionSupplement
extends OptionBase
{
@XmlElement(required = true)
protected EquityPremium equityPremium;
@XmlElement(required = true)
protected EquityExerciseValuationSettlement equityExercise;
protected Boolean exchangeLookAlike;
@XmlSchemaType(name = "token")
protected MethodOfAdjustmentEnum methodOfAdjustment;
protected BigDecimal optionEntitlement;
protected BigDecimal multiplier;
protected SwaptionPhysicalSettlement clearingInstructions;
@XmlElement(required = true)
protected DividendSwapTransactionSupplement dividendSwapTransactionSupplement;
/**
* Gets the value of the equityPremium property.
*
* @return
* possible object is
* {@link EquityPremium }
*
*/
public EquityPremium getEquityPremium() {
return equityPremium;
}
/**
* Sets the value of the equityPremium property.
*
* @param value
* allowed object is
* {@link EquityPremium }
*
*/
public void setEquityPremium(EquityPremium value) {
this.equityPremium = value;
}
/**
* Gets the value of the equityExercise property.
*
* @return
* possible object is
* {@link EquityExerciseValuationSettlement }
*
*/
public EquityExerciseValuationSettlement getEquityExercise() {
return equityExercise;
}
/**
* Sets the value of the equityExercise property.
*
* @param value
* allowed object is
* {@link EquityExerciseValuationSettlement }
*
*/
public void setEquityExercise(EquityExerciseValuationSettlement value) {
this.equityExercise = value;
}
/**
* Gets the value of the exchangeLookAlike property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isExchangeLookAlike() {
return exchangeLookAlike;
}
/**
* Sets the value of the exchangeLookAlike property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setExchangeLookAlike(Boolean value) {
this.exchangeLookAlike = value;
}
/**
* Gets the value of the methodOfAdjustment property.
*
* @return
* possible object is
* {@link MethodOfAdjustmentEnum }
*
*/
public MethodOfAdjustmentEnum getMethodOfAdjustment() {
return methodOfAdjustment;
}
/**
* Sets the value of the methodOfAdjustment property.
*
* @param value
* allowed object is
* {@link MethodOfAdjustmentEnum }
*
*/
public void setMethodOfAdjustment(MethodOfAdjustmentEnum value) {
this.methodOfAdjustment = value;
}
/**
* Gets the value of the optionEntitlement property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getOptionEntitlement() {
return optionEntitlement;
}
/**
* Sets the value of the optionEntitlement property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setOptionEntitlement(BigDecimal value) {
this.optionEntitlement = value;
}
/**
* Gets the value of the multiplier property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getMultiplier() {
return multiplier;
}
/**
* Sets the value of the multiplier property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setMultiplier(BigDecimal value) {
this.multiplier = value;
}
/**
* Gets the value of the clearingInstructions property.
*
* @return
* possible object is
* {@link SwaptionPhysicalSettlement }
*
*/
public SwaptionPhysicalSettlement getClearingInstructions() {
return clearingInstructions;
}
/**
* Sets the value of the clearingInstructions property.
*
* @param value
* allowed object is
* {@link SwaptionPhysicalSettlement }
*
*/
public void setClearingInstructions(SwaptionPhysicalSettlement value) {
this.clearingInstructions = value;
}
/**
* Gets the value of the dividendSwapTransactionSupplement property.
*
* @return
* possible object is
* {@link DividendSwapTransactionSupplement }
*
*/
public DividendSwapTransactionSupplement getDividendSwapTransactionSupplement() {
return dividendSwapTransactionSupplement;
}
/**
* Sets the value of the dividendSwapTransactionSupplement property.
*
* @param value
* allowed object is
* {@link DividendSwapTransactionSupplement }
*
*/
public void setDividendSwapTransactionSupplement(DividendSwapTransactionSupplement value) {
this.dividendSwapTransactionSupplement = value;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy