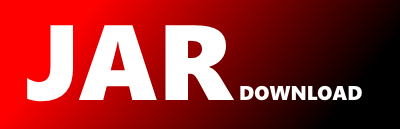
org.fpml.fpml_5.confirmation.ExtraordinaryEvents Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.05.10 at 03:58:40 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlType;
/**
* Where the underlying is shares, defines market events affecting the issuer of those shares that may require the terms of the transaction to be adjusted.
*
* Java class for ExtraordinaryEvents complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="ExtraordinaryEvents">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="mergerEvents" type="{http://www.fpml.org/FpML-5/confirmation}EquityCorporateEvents" minOccurs="0"/>
* <element name="tenderOffer" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="tenderOfferEvents" type="{http://www.fpml.org/FpML-5/confirmation}EquityCorporateEvents" minOccurs="0"/>
* <element name="compositionOfCombinedConsideration" type="{http://www.w3.org/2001/XMLSchema}boolean" minOccurs="0"/>
* <element name="indexAdjustmentEvents" type="{http://www.fpml.org/FpML-5/confirmation}IndexAdjustmentEvents" minOccurs="0"/>
* <choice>
* <element name="additionalDisruptionEvents" type="{http://www.fpml.org/FpML-5/confirmation}AdditionalDisruptionEvents"/>
* <element name="failureToDeliver" type="{http://www.w3.org/2001/XMLSchema}boolean"/>
* </choice>
* <element name="representations" type="{http://www.fpml.org/FpML-5/confirmation}Representations" minOccurs="0"/>
* <element name="nationalisationOrInsolvency" type="{http://www.fpml.org/FpML-5/confirmation}NationalisationOrInsolvencyOrDelistingEventEnum" minOccurs="0"/>
* <element name="delisting" type="{http://www.fpml.org/FpML-5/confirmation}NationalisationOrInsolvencyOrDelistingEventEnum" minOccurs="0"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}ExchangeIdentifier.model" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "ExtraordinaryEvents", propOrder = {
"mergerEvents",
"tenderOffer",
"tenderOfferEvents",
"compositionOfCombinedConsideration",
"indexAdjustmentEvents",
"additionalDisruptionEvents",
"failureToDeliver",
"representations",
"nationalisationOrInsolvency",
"delisting",
"relatedExchangeId",
"optionsExchangeId",
"specifiedExchangeId"
})
public class ExtraordinaryEvents {
protected EquityCorporateEvents mergerEvents;
protected Boolean tenderOffer;
protected EquityCorporateEvents tenderOfferEvents;
protected Boolean compositionOfCombinedConsideration;
protected IndexAdjustmentEvents indexAdjustmentEvents;
protected AdditionalDisruptionEvents additionalDisruptionEvents;
protected Boolean failureToDeliver;
protected Representations representations;
@XmlSchemaType(name = "token")
protected NationalisationOrInsolvencyOrDelistingEventEnum nationalisationOrInsolvency;
@XmlSchemaType(name = "token")
protected NationalisationOrInsolvencyOrDelistingEventEnum delisting;
protected List relatedExchangeId;
protected List optionsExchangeId;
protected List specifiedExchangeId;
/**
* Gets the value of the mergerEvents property.
*
* @return
* possible object is
* {@link EquityCorporateEvents }
*
*/
public EquityCorporateEvents getMergerEvents() {
return mergerEvents;
}
/**
* Sets the value of the mergerEvents property.
*
* @param value
* allowed object is
* {@link EquityCorporateEvents }
*
*/
public void setMergerEvents(EquityCorporateEvents value) {
this.mergerEvents = value;
}
/**
* Gets the value of the tenderOffer property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isTenderOffer() {
return tenderOffer;
}
/**
* Sets the value of the tenderOffer property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setTenderOffer(Boolean value) {
this.tenderOffer = value;
}
/**
* Gets the value of the tenderOfferEvents property.
*
* @return
* possible object is
* {@link EquityCorporateEvents }
*
*/
public EquityCorporateEvents getTenderOfferEvents() {
return tenderOfferEvents;
}
/**
* Sets the value of the tenderOfferEvents property.
*
* @param value
* allowed object is
* {@link EquityCorporateEvents }
*
*/
public void setTenderOfferEvents(EquityCorporateEvents value) {
this.tenderOfferEvents = value;
}
/**
* Gets the value of the compositionOfCombinedConsideration property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isCompositionOfCombinedConsideration() {
return compositionOfCombinedConsideration;
}
/**
* Sets the value of the compositionOfCombinedConsideration property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setCompositionOfCombinedConsideration(Boolean value) {
this.compositionOfCombinedConsideration = value;
}
/**
* Gets the value of the indexAdjustmentEvents property.
*
* @return
* possible object is
* {@link IndexAdjustmentEvents }
*
*/
public IndexAdjustmentEvents getIndexAdjustmentEvents() {
return indexAdjustmentEvents;
}
/**
* Sets the value of the indexAdjustmentEvents property.
*
* @param value
* allowed object is
* {@link IndexAdjustmentEvents }
*
*/
public void setIndexAdjustmentEvents(IndexAdjustmentEvents value) {
this.indexAdjustmentEvents = value;
}
/**
* Gets the value of the additionalDisruptionEvents property.
*
* @return
* possible object is
* {@link AdditionalDisruptionEvents }
*
*/
public AdditionalDisruptionEvents getAdditionalDisruptionEvents() {
return additionalDisruptionEvents;
}
/**
* Sets the value of the additionalDisruptionEvents property.
*
* @param value
* allowed object is
* {@link AdditionalDisruptionEvents }
*
*/
public void setAdditionalDisruptionEvents(AdditionalDisruptionEvents value) {
this.additionalDisruptionEvents = value;
}
/**
* Gets the value of the failureToDeliver property.
*
* @return
* possible object is
* {@link Boolean }
*
*/
public Boolean isFailureToDeliver() {
return failureToDeliver;
}
/**
* Sets the value of the failureToDeliver property.
*
* @param value
* allowed object is
* {@link Boolean }
*
*/
public void setFailureToDeliver(Boolean value) {
this.failureToDeliver = value;
}
/**
* Gets the value of the representations property.
*
* @return
* possible object is
* {@link Representations }
*
*/
public Representations getRepresentations() {
return representations;
}
/**
* Sets the value of the representations property.
*
* @param value
* allowed object is
* {@link Representations }
*
*/
public void setRepresentations(Representations value) {
this.representations = value;
}
/**
* Gets the value of the nationalisationOrInsolvency property.
*
* @return
* possible object is
* {@link NationalisationOrInsolvencyOrDelistingEventEnum }
*
*/
public NationalisationOrInsolvencyOrDelistingEventEnum getNationalisationOrInsolvency() {
return nationalisationOrInsolvency;
}
/**
* Sets the value of the nationalisationOrInsolvency property.
*
* @param value
* allowed object is
* {@link NationalisationOrInsolvencyOrDelistingEventEnum }
*
*/
public void setNationalisationOrInsolvency(NationalisationOrInsolvencyOrDelistingEventEnum value) {
this.nationalisationOrInsolvency = value;
}
/**
* Gets the value of the delisting property.
*
* @return
* possible object is
* {@link NationalisationOrInsolvencyOrDelistingEventEnum }
*
*/
public NationalisationOrInsolvencyOrDelistingEventEnum getDelisting() {
return delisting;
}
/**
* Sets the value of the delisting property.
*
* @param value
* allowed object is
* {@link NationalisationOrInsolvencyOrDelistingEventEnum }
*
*/
public void setDelisting(NationalisationOrInsolvencyOrDelistingEventEnum value) {
this.delisting = value;
}
/**
* Gets the value of the relatedExchangeId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the relatedExchangeId property.
*
*
* For example, to add a new item, do as follows:
*
* getRelatedExchangeId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ExchangeId }
*
*
*/
public List getRelatedExchangeId() {
if (relatedExchangeId == null) {
relatedExchangeId = new ArrayList();
}
return this.relatedExchangeId;
}
/**
* Gets the value of the optionsExchangeId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the optionsExchangeId property.
*
*
* For example, to add a new item, do as follows:
*
* getOptionsExchangeId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ExchangeId }
*
*
*/
public List getOptionsExchangeId() {
if (optionsExchangeId == null) {
optionsExchangeId = new ArrayList();
}
return this.optionsExchangeId;
}
/**
* Gets the value of the specifiedExchangeId property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the specifiedExchangeId property.
*
*
* For example, to add a new item, do as follows:
*
* getSpecifiedExchangeId().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ExchangeId }
*
*
*/
public List getSpecifiedExchangeId() {
if (specifiedExchangeId == null) {
specifiedExchangeId = new ArrayList();
}
return this.specifiedExchangeId;
}
}