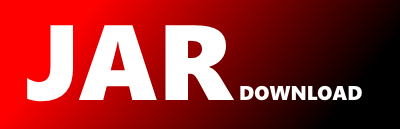
org.fpml.fpml_5.confirmation.FacilitySummary Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.05.10 at 03:58:40 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.datatype.XMLGregorianCalendar;
/**
* A short form of a facility.
*
* Java class for FacilitySummary complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FacilitySummary">
* <complexContent>
* <extension base="{http://www.fpml.org/FpML-5/confirmation}FacilityIdentifier">
* <sequence>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}FacilityRoles.model"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}FacilityDates.model"/>
* <group ref="{http://www.fpml.org/FpML-5/confirmation}FacilityCommitment.model"/>
* </sequence>
* </extension>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FacilitySummary", propOrder = {
"borrowerPartyReference",
"coBorrowerPartyReference",
"agentPartyReference",
"lcIssuingBankPartyReference",
"guarantorPartyReference",
"startDate",
"expiryDate",
"maturityDate",
"currentCommitment",
"originalCommitment",
"commitmentSchedule",
"dealFxRate"
})
@XmlSeeAlso({
AbstractFacility.class
})
public class FacilitySummary
extends FacilityIdentifier
{
@XmlElement(required = true)
protected PartyReference borrowerPartyReference;
protected List coBorrowerPartyReference;
protected PartyReference agentPartyReference;
protected List lcIssuingBankPartyReference;
protected List guarantorPartyReference;
@XmlElement(required = true)
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar startDate;
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar expiryDate;
@XmlSchemaType(name = "date")
protected XMLGregorianCalendar maturityDate;
@XmlElement(required = true)
protected FacilityCommitment currentCommitment;
protected MoneyWithParticipantShare originalCommitment;
protected CommitmentSchedule commitmentSchedule;
protected FxTerms dealFxRate;
/**
* Gets the value of the borrowerPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getBorrowerPartyReference() {
return borrowerPartyReference;
}
/**
* Sets the value of the borrowerPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setBorrowerPartyReference(PartyReference value) {
this.borrowerPartyReference = value;
}
/**
* Gets the value of the coBorrowerPartyReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the coBorrowerPartyReference property.
*
*
* For example, to add a new item, do as follows:
*
* getCoBorrowerPartyReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyReference }
*
*
*/
public List getCoBorrowerPartyReference() {
if (coBorrowerPartyReference == null) {
coBorrowerPartyReference = new ArrayList();
}
return this.coBorrowerPartyReference;
}
/**
* Gets the value of the agentPartyReference property.
*
* @return
* possible object is
* {@link PartyReference }
*
*/
public PartyReference getAgentPartyReference() {
return agentPartyReference;
}
/**
* Sets the value of the agentPartyReference property.
*
* @param value
* allowed object is
* {@link PartyReference }
*
*/
public void setAgentPartyReference(PartyReference value) {
this.agentPartyReference = value;
}
/**
* Gets the value of the lcIssuingBankPartyReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the lcIssuingBankPartyReference property.
*
*
* For example, to add a new item, do as follows:
*
* getLcIssuingBankPartyReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyReference }
*
*
*/
public List getLcIssuingBankPartyReference() {
if (lcIssuingBankPartyReference == null) {
lcIssuingBankPartyReference = new ArrayList();
}
return this.lcIssuingBankPartyReference;
}
/**
* Gets the value of the guarantorPartyReference property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the guarantorPartyReference property.
*
*
* For example, to add a new item, do as follows:
*
* getGuarantorPartyReference().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link PartyReference }
*
*
*/
public List getGuarantorPartyReference() {
if (guarantorPartyReference == null) {
guarantorPartyReference = new ArrayList();
}
return this.guarantorPartyReference;
}
/**
* Gets the value of the startDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getStartDate() {
return startDate;
}
/**
* Sets the value of the startDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setStartDate(XMLGregorianCalendar value) {
this.startDate = value;
}
/**
* Gets the value of the expiryDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getExpiryDate() {
return expiryDate;
}
/**
* Sets the value of the expiryDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setExpiryDate(XMLGregorianCalendar value) {
this.expiryDate = value;
}
/**
* Gets the value of the maturityDate property.
*
* @return
* possible object is
* {@link XMLGregorianCalendar }
*
*/
public XMLGregorianCalendar getMaturityDate() {
return maturityDate;
}
/**
* Sets the value of the maturityDate property.
*
* @param value
* allowed object is
* {@link XMLGregorianCalendar }
*
*/
public void setMaturityDate(XMLGregorianCalendar value) {
this.maturityDate = value;
}
/**
* Gets the value of the currentCommitment property.
*
* @return
* possible object is
* {@link FacilityCommitment }
*
*/
public FacilityCommitment getCurrentCommitment() {
return currentCommitment;
}
/**
* Sets the value of the currentCommitment property.
*
* @param value
* allowed object is
* {@link FacilityCommitment }
*
*/
public void setCurrentCommitment(FacilityCommitment value) {
this.currentCommitment = value;
}
/**
* Gets the value of the originalCommitment property.
*
* @return
* possible object is
* {@link MoneyWithParticipantShare }
*
*/
public MoneyWithParticipantShare getOriginalCommitment() {
return originalCommitment;
}
/**
* Sets the value of the originalCommitment property.
*
* @param value
* allowed object is
* {@link MoneyWithParticipantShare }
*
*/
public void setOriginalCommitment(MoneyWithParticipantShare value) {
this.originalCommitment = value;
}
/**
* Gets the value of the commitmentSchedule property.
*
* @return
* possible object is
* {@link CommitmentSchedule }
*
*/
public CommitmentSchedule getCommitmentSchedule() {
return commitmentSchedule;
}
/**
* Sets the value of the commitmentSchedule property.
*
* @param value
* allowed object is
* {@link CommitmentSchedule }
*
*/
public void setCommitmentSchedule(CommitmentSchedule value) {
this.commitmentSchedule = value;
}
/**
* Gets the value of the dealFxRate property.
*
* @return
* possible object is
* {@link FxTerms }
*
*/
public FxTerms getDealFxRate() {
return dealFxRate;
}
/**
* Sets the value of the dealFxRate property.
*
* @param value
* allowed object is
* {@link FxTerms }
*
*/
public void setDealFxRate(FxTerms value) {
this.dealFxRate = value;
}
}