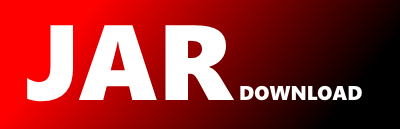
org.fpml.fpml_5.confirmation.FloatingRateDefinition Maven / Gradle / Ivy
//
// This file was generated by the JavaTM Architecture for XML Binding(JAXB) Reference Implementation, v2.3.2
// See https://javaee.github.io/jaxb-v2/
// Any modifications to this file will be lost upon recompilation of the source schema.
// Generated on: 2024.05.10 at 03:58:40 PM UTC
//
package org.fpml.fpml_5.confirmation;
import java.math.BigDecimal;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
/**
* A type defining parameters associated with a floating rate reset. This type forms part of the cashflows representation of a stream.
*
* Java class for FloatingRateDefinition complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="FloatingRateDefinition">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="calculatedRate" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="rateObservation" type="{http://www.fpml.org/FpML-5/confirmation}RateObservation" maxOccurs="unbounded" minOccurs="0"/>
* <element name="floatingRateMultiplier" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="spread" type="{http://www.w3.org/2001/XMLSchema}decimal" minOccurs="0"/>
* <element name="capRate" type="{http://www.fpml.org/FpML-5/confirmation}Strike" maxOccurs="unbounded" minOccurs="0"/>
* <element name="floorRate" type="{http://www.fpml.org/FpML-5/confirmation}Strike" maxOccurs="unbounded" minOccurs="0"/>
* </sequence>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "FloatingRateDefinition", propOrder = {
"calculatedRate",
"rateObservation",
"floatingRateMultiplier",
"spread",
"capRate",
"floorRate"
})
public class FloatingRateDefinition {
protected BigDecimal calculatedRate;
protected List rateObservation;
protected BigDecimal floatingRateMultiplier;
protected BigDecimal spread;
protected List capRate;
protected List floorRate;
/**
* Gets the value of the calculatedRate property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getCalculatedRate() {
return calculatedRate;
}
/**
* Sets the value of the calculatedRate property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setCalculatedRate(BigDecimal value) {
this.calculatedRate = value;
}
/**
* Gets the value of the rateObservation property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the rateObservation property.
*
*
* For example, to add a new item, do as follows:
*
* getRateObservation().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link RateObservation }
*
*
*/
public List getRateObservation() {
if (rateObservation == null) {
rateObservation = new ArrayList();
}
return this.rateObservation;
}
/**
* Gets the value of the floatingRateMultiplier property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getFloatingRateMultiplier() {
return floatingRateMultiplier;
}
/**
* Sets the value of the floatingRateMultiplier property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setFloatingRateMultiplier(BigDecimal value) {
this.floatingRateMultiplier = value;
}
/**
* Gets the value of the spread property.
*
* @return
* possible object is
* {@link BigDecimal }
*
*/
public BigDecimal getSpread() {
return spread;
}
/**
* Sets the value of the spread property.
*
* @param value
* allowed object is
* {@link BigDecimal }
*
*/
public void setSpread(BigDecimal value) {
this.spread = value;
}
/**
* Gets the value of the capRate property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the capRate property.
*
*
* For example, to add a new item, do as follows:
*
* getCapRate().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Strike }
*
*
*/
public List getCapRate() {
if (capRate == null) {
capRate = new ArrayList();
}
return this.capRate;
}
/**
* Gets the value of the floorRate property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the floorRate property.
*
*
* For example, to add a new item, do as follows:
*
* getFloorRate().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Strike }
*
*
*/
public List getFloorRate() {
if (floorRate == null) {
floorRate = new ArrayList();
}
return this.floorRate;
}
}