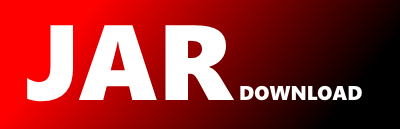
core_pure_changetoken.changetoken.pure Maven / Gradle / Ivy
The newest version!
// Copyright 2022 Goldman Sachs
//
// Licensed under the Apache License, Version 2.0 (the "License");
// you may not use this file except in compliance with the License.
// You may obtain a copy of the License at
//
// http://www.apache.org/licenses/LICENSE-2.0
//
// Unless required by applicable law or agreed to in writing, software
// distributed under the License is distributed on an "AS IS" BASIS,
// WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
// See the License for the specific language governing permissions and
// limitations under the License.
import meta::pure::changetoken::*;
Class <>
{doc.doc='change tokens describe the changes to a data model from one version to the next. They are generated by a tool, then reviewed and updated by developers, and committed alongside the model changes.'}
meta::pure::changetoken::ChangeToken
{
}
Class
{doc.doc='Version describes a single release to a data model and contains an order-dependent list of change tokens that transform the model from prevVersion to the present version.'}
meta::pure::changetoken::Version
{
{doc.doc='string version label, e.g. "ftdm:"'}
version: String[1];
{doc.doc='reference to the previous version label'}
prevVersion: String[0..1];
{doc.doc='list of change tokens that describe the change to the model from prevVersion to Version'}
changeTokens: ChangeToken[*];
toString() { 'Version('
+ 'version=' + $this.version->toString() + ','
+ 'prevVersion=' + $this.prevVersion->map(p|$p->toString())->joinStrings(',') + ','
+ 'changeTokens=[' + $this.changeTokens->map(c|$c->toString())->joinStrings(',') + ']'
+ ')' }: String[1];
}
Class
{doc.doc='A single linear sequence of versions of how the present model evolved over time'}
meta::pure::changetoken::Versions
[
linearVersions: $this.versions->size()->range()->map(i|
if($i==0,
|$this.versions->at($i).prevVersion->size()==0,
|$this.versions->at($i).prevVersion == $this.versions->at($i-1).version))
->fold({b,res|$b && $res}, true)
]
{
{doc.doc='all versions in a sorted order from oldest to newest'}
versions: meta::pure::changetoken::Version[*];
toString() { 'Versions('
+ 'versions=[' + $this.versions->map(v|$v->toString())->joinStrings(',') + ']'
+ ')' }: String[1];
}
Class <>
{doc.doc='the root class for any change tokens affecting a single class'}
meta::pure::changetoken::ClassChangeToken extends ChangeToken
{
{doc.doc='affected class name, e.g. "meta::domain::ftdm::MyClass". It is a string because the class may no longer be present in the current model.'}
class: String[1];
}
Class
{doc.doc='a class was added in this version'}
meta::pure::changetoken::AddedClass extends ClassChangeToken
{
toString() { 'AddedClass('
+ 'class=' + $this.class->toString()
+ ')' }: String[1];
}
Class
{doc.doc='a class was removed in this version'}
meta::pure::changetoken::RemovedClass extends ClassChangeToken
{
toString() { 'RemovedClass('
+ 'class=' + $this.class->toString()
+ ')' }: String[1];
}
Class
{doc.doc='a class was renamed in this version'}
meta::pure::changetoken::RenamedClass extends ClassChangeToken
{
newName: String[1];
toString() { 'RenamedClass('
+ 'class=' + $this.class->toString()
+ 'newName=' + $this.newName->toString()
+ ')' }: String[1];
}
Class <>
{doc.doc='root of a hierarchy of getters for a default value of a newly-added field'}
meta::pure::changetoken::FieldGetter
{
}
Class
{doc.doc='a constant value, e.g. 100 or "SomeValue"'}
meta::pure::changetoken::ConstValue extends FieldGetter
{
value: Any[0..1];
toString() { 'ConstValue('
+ 'value=' + $this.value->map(v|$v->toString())->joinStrings(',')
+ ')' }: String[1];
}
Class <>
{doc.doc='a way to refer to another field for its value'}
meta::pure::changetoken::FieldReference
{
}
Class
{doc.doc='refer to another field using a relative path in the serialized form'}
meta::pure::changetoken::RelativeFieldReference extends FieldReference
{
{doc.doc='relative path, e.g. "../../some/other/field"'}
path: String[1];
toString() { 'RelativeFieldReference('
+ 'path=' + $this.path->toString()
+ ')' }: String[1];
}
Class
{doc.doc='a field getter, which copies a value from another field'}
meta::pure::changetoken::CopyValue extends FieldGetter
{
{doc.doc='source field for the copied value'}
source: FieldReference[1];
toString() { 'CopyValue('
+ 'source=' + $this.source->toString()
+ ')' }: String[1];
}
Class
<>
{doc.doc='a field addition or removal to/from a class'}
meta::pure::changetoken::AddRemoveField extends ClassChangeToken
{
fieldName: String[1];
{doc.doc='a field\'s type'}
fieldType: String[1];
{doc.doc='where the default value for this field comes from'}
defaultValue: meta::pure::changetoken::FieldGetter[1];
{doc.doc='whether to fail if casting removes a non-default value'}
safeCast: Boolean[1];
}
Class
{doc.doc='a field is added to a class. We use the default for upcast to create and for downcast to delete if equal.'}
meta::pure::changetoken::AddField extends AddRemoveField
{
toString() { 'AddField('
+ 'class=' + $this.class->toString() + ','
+ 'fieldName=' + $this.fieldName->toString() + ','
+ 'fieldType=' + $this.fieldType->toString() + ','
+ 'defaultValue=' + $this.defaultValue->toString() + ','
+ 'safeCast=' + $this.safeCast->toString()
+ ')' }: String[1];
}
Class
{doc.doc='a field is removed from a class. We use the default for downcast to create and for upcast to delete if equal.'}
meta::pure::changetoken::RemoveField extends AddRemoveField
{
toString() { 'RemoveField('
+ 'class=' + $this.class->toString() + ','
+ 'fieldName=' + $this.fieldName->toString() + ','
+ 'fieldType=' + $this.fieldType->toString() + ','
+ 'defaultValue=' + $this.defaultValue->toString() + ','
+ 'safeCast=' + $this.safeCast->toString()
+ ')' }: String[1];
}
Class
{doc.doc='rename a field of a class'}
meta::pure::changetoken::RenameField extends ClassChangeToken
{
oldFieldName: String[1..*];
newFieldName: String[1..*];
toString() { 'RenameField('
+ 'oldFieldName=' + $this.oldFieldName->joinStrings('.')->toString() + ','
+ 'newFieldName=' + $this.newFieldName->joinStrings('.')->toString()
+ ')' }: String[1];
}
Class
{doc.doc='change the type of a field'}
meta::pure::changetoken::ChangeFieldType extends ClassChangeToken
{
fieldName: String[1];
oldFieldType: String[1];
newFieldType: String[1];
toString() { 'ChangeFieldType('
+ 'fieldName=' + $this.fieldName->toString() + ','
+ 'oldFieldType=' + $this.oldFieldType->toString() + ','
+ 'newFieldType=' + $this.newFieldType->toString()
+ ')' }: String[1];
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy