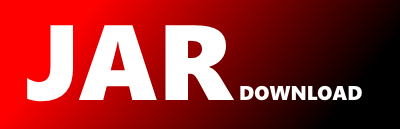
org.finos.legend.pure.generated.Package_Impl Maven / Gradle / Ivy
package org.finos.legend.pure.generated;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.factory.Lists;
import org.eclipse.collections.api.factory.Maps;
import org.eclipse.collections.api.list.ListIterable;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.map.MutableMap;
import org.finos.legend.pure.m3.execution.ExecutionSupport;
import org.finos.legend.pure.m4.ModelRepository;
import org.finos.legend.pure.m4.coreinstance.CoreInstance;
import org.finos.legend.pure.m4.coreinstance.SourceInformation;
import org.finos.legend.pure.m4.coreinstance.factory.CoreInstanceFactory;
import org.finos.legend.pure.runtime.java.compiled.execution.*;
import org.finos.legend.pure.runtime.java.compiled.execution.sourceInformation.E_;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.*;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.coreinstance.ReflectiveCoreInstance;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.coreinstance.ValCoreInstance;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.coreinstance.GetterOverrideExecutor;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.function.*;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.function.defended.*;
public class Package_Impl extends Root_meta_pure_metamodel_PackageableElement_Impl implements org.finos.legend.pure.m3.coreinstance.Package
{
public static final String tempTypeName = "Package";
private static final String tempFullTypeId = "Package";
private static final KeyIndex KEY_INDEX = keyIndexBuilder(8)
.withKey("Root::meta::pure::metamodel::ModelElement", "name")
.withKeys("Root::meta::pure::metamodel::PackageableElement", "package", "referenceUsages")
.withKey(tempFullTypeId, "children")
.withKey("Root::meta::pure::metamodel::extension::ElementWithStereotypes", "stereotypes")
.withKeys("Root::meta::pure::metamodel::type::Any", "classifierGenericType", "elementOverride")
.withKey("Root::meta::pure::metamodel::extension::ElementWithTaggedValues", "taggedValues")
.build();
private CoreInstance classifier;
public Package_Impl(String id)
{
super(id);
}
public Package_Impl(String name, SourceInformation sourceInformation, CoreInstance classifier)
{
this(name == null ? "Anonymous_NoCounter": name);
this.setSourceInformation(sourceInformation);
this.classifier = classifier;
}
public static final CoreInstanceFactory FACTORY = new org.finos.legend.pure.runtime.java.compiled.generation.processors.support.coreinstance.BaseJavaModelCoreInstanceFactory()
{
@Override
public CoreInstance createCoreInstance(String name, int internalSyntheticId, SourceInformation sourceInformation, CoreInstance classifier, ModelRepository repository, boolean persistent)
{
return new Package_Impl(name, sourceInformation, classifier);
}
@Override
public boolean supports(String classifierPath)
{
return tempFullTypeId.equals(classifierPath);
}
};
@Override
public CoreInstance getClassifier()
{
return this.classifier;
}
@Override
public RichIterable getKeys()
{
return KEY_INDEX.getKeys();
}
@Override
public ListIterable getRealKeyByName(String name)
{
return KEY_INDEX.getRealKeyByName(name);
}
@Override
public CoreInstance getValueForMetaPropertyToOne(String keyName)
{
switch (keyName)
{
case "classifierGenericType":
{
return ValCoreInstance.toCoreInstance(_classifierGenericType());
}
case "elementOverride":
{
return ValCoreInstance.toCoreInstance(_elementOverride());
}
case "name":
{
return ValCoreInstance.toCoreInstance(_name());
}
case "package":
{
return ValCoreInstance.toCoreInstance(_package());
}
default:
{
return super.getValueForMetaPropertyToOne(keyName);
}
}
}
@Override
public ListIterable getValueForMetaPropertyToMany(String keyName)
{
switch (keyName)
{
case "children":
{
return ValCoreInstance.toCoreInstances(_children());
}
case "referenceUsages":
{
return ValCoreInstance.toCoreInstances(_referenceUsages());
}
case "stereotypes":
{
return ValCoreInstance.toCoreInstances(_stereotypes());
}
case "taggedValues":
{
return ValCoreInstance.toCoreInstances(_taggedValues());
}
default:
{
return super.getValueForMetaPropertyToMany(keyName);
}
}
}
private org.finos.legend.pure.m3.coreinstance.Package _taggedValues(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val, boolean add)
{
if (val == null)
{
if (!add)
{
this._taggedValues = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).add(val);
}
else
{
this._taggedValues = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _taggedValues(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val, boolean add)
{
if (add)
{
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).addAllIterable(val);
}
else
{
this._taggedValues = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValues(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val)
{
return this._taggedValues(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
return this._taggedValues(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val)
{
return this._taggedValues(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesRemove()
{
this._taggedValues = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).remove(val);
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverride(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride val)
{
this._elementOverride = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverride(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride> val)
{
return _elementOverride(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverrideRemove()
{
this._elementOverride = null;
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _stereotypes(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val, boolean add)
{
if (val == null)
{
if (!add)
{
this._stereotypes = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).add(val);
}
else
{
this._stereotypes = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _stereotypes(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val, boolean add)
{
if (add)
{
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).addAllIterable(val);
}
else
{
this._stereotypes = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypes(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val)
{
return this._stereotypes(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
return this._stereotypes(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val)
{
return this._stereotypes(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemove()
{
this._stereotypes = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).remove(val);
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddCoreInstance(CoreInstance val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddAllCoreInstance(RichIterable extends CoreInstance> val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesCoreInstance(RichIterable extends org.finos.legend.pure.m4.coreinstance.CoreInstance> val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemoveCoreInstance(CoreInstance val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public RichIterable _stereotypesCoreInstance()
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _name(java.lang.String val)
{
this._name = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _name(RichIterable extends java.lang.String> val)
{
return _name(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _nameRemove()
{
this._name = null;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _package(org.finos.legend.pure.m3.coreinstance.Package val)
{
this._package = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _package(RichIterable extends org.finos.legend.pure.m3.coreinstance.Package> val)
{
return _package(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _packageRemove()
{
this._package = null;
return this;
}
public RichIterable _children = Lists.mutable.with();
private org.finos.legend.pure.m3.coreinstance.Package _children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val, boolean add)
{
if (val == null)
{
if (!add)
{
this._children = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).add(val);
}
else
{
this._children = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _children(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val, boolean add)
{
if (add)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).addAllIterable(val);
}
else
{
this._children = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _children(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val)
{
return this._children(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
return this._children(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val)
{
return this._children(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenRemove()
{
this._children = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).remove(val);
return this;
}
public void _reverse_children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).add(val);
}
public void _sever_reverse_children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).remove(val);
}
public RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> _children()
{
return this._elementOverride() == null || !GetterOverrideExecutor.class.isInstance(this._elementOverride()) ? this._children : (RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement>)((GetterOverrideExecutor)this._elementOverride()).executeToMany(this, "Package", "children");
}
private org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val, boolean add)
{
if (val == null)
{
if (!add)
{
this._referenceUsages = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).add(val);
}
else
{
this._referenceUsages = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val, boolean add)
{
if (add)
{
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).addAllIterable(val);
}
else
{
this._referenceUsages = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val)
{
return this._referenceUsages(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
return this._referenceUsages(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val)
{
return this._referenceUsages(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesRemove()
{
this._referenceUsages = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).remove(val);
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericType(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType val)
{
this._classifierGenericType = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericType(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType> val)
{
return _classifierGenericType(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericTypeRemove()
{
this._classifierGenericType = null;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package copy()
{
return new Package_Impl(this);
}
public Package_Impl(org.finos.legend.pure.m3.coreinstance.Package src)
{
this("Anonymous_NoCounter");
this.classifier = ((Package_Impl)src).classifier;
this._taggedValues = Lists.mutable.ofAll(((Package_Impl)src)._taggedValues);
this._elementOverride = (org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride)((Package_Impl)src)._elementOverride;
this._stereotypes = Lists.mutable.ofAll(((Package_Impl)src)._stereotypes);
this._name = (java.lang.String)((Package_Impl)src)._name;
this._package = (org.finos.legend.pure.m3.coreinstance.Package)((Package_Impl)src)._package;
this._children = Lists.mutable.ofAll(((Package_Impl)src)._children);
this._referenceUsages = Lists.mutable.ofAll(((Package_Impl)src)._referenceUsages);
this._classifierGenericType = (org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType)((Package_Impl)src)._classifierGenericType;
}
@Override
public String getFullSystemPath()
{
return tempFullTypeId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy