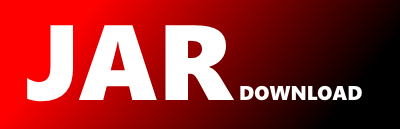
org.finos.legend.pure.generated.Package_LazyImpl Maven / Gradle / Ivy
package org.finos.legend.pure.generated;
import java.util.concurrent.atomic.AtomicBoolean;
import org.eclipse.collections.api.list.ListIterable;
import org.eclipse.collections.api.list.MutableList;
import org.eclipse.collections.api.RichIterable;
import org.eclipse.collections.api.map.MutableMap;
import org.eclipse.collections.impl.factory.Lists;
import org.eclipse.collections.impl.factory.Maps;
import org.eclipse.collections.impl.map.mutable.UnifiedMap;
import org.eclipse.collections.api.map.ImmutableMap;
import org.eclipse.collections.impl.list.mutable.FastList;
import org.finos.legend.pure.m4.coreinstance.CoreInstance;
import org.finos.legend.pure.m4.coreinstance.factory.CoreInstanceFactory;
import org.finos.legend.pure.m4.ModelRepository;
import org.finos.legend.pure.m4.coreinstance.SourceInformation;
import org.finos.legend.pure.runtime.java.compiled.metadata.MetadataLazy;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.*;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.function.defended.*;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.function.*;
import org.finos.legend.pure.runtime.java.compiled.execution.*;
import org.finos.legend.pure.runtime.java.compiled.execution.sourceInformation.*;
import org.finos.legend.pure.runtime.java.compiled.generation.processors.support.coreinstance.*;
import org.finos.legend.pure.runtime.java.compiled.serialization.model.*;
import org.finos.legend.pure.m3.execution.ExecutionSupport;
import org.finos.legend.pure.m4.coreinstance.CoreInstance;
public class Package_LazyImpl extends AbstractLazyReflectiveCoreInstance implements org.finos.legend.pure.m3.coreinstance.Package
{
public static final String tempTypeName = "Package";
private static final String tempFullTypeId = "Package";
private static final KeyIndex KEY_INDEX = keyIndexBuilder(8)
.withKey("Root::meta::pure::metamodel::ModelElement", "name")
.withKeys("Root::meta::pure::metamodel::PackageableElement", "package", "referenceUsages")
.withKey(tempFullTypeId, "children")
.withKey("Root::meta::pure::metamodel::extension::ElementWithStereotypes", "stereotypes")
.withKeys("Root::meta::pure::metamodel::type::Any", "classifierGenericType", "elementOverride")
.withKey("Root::meta::pure::metamodel::extension::ElementWithTaggedValues", "taggedValues")
.build();
public Package_LazyImpl(Obj instance, MetadataLazy metadataLazy)
{
super(instance, metadataLazy);
}
public Package_LazyImpl(String id, org.finos.legend.pure.m4.coreinstance.SourceInformation sourceInformation, ImmutableMap vals, MetadataLazy metadataLazy)
{
super(id, sourceInformation, metadataLazy, vals);
}
public Package_LazyImpl(String id, org.finos.legend.pure.m4.coreinstance.SourceInformation sourceInformation, ImmutableMap vals, MetadataLazy metadataLazy, CoreInstance classifier)
{
super(id, sourceInformation, metadataLazy, vals, classifier);
}
public Package_LazyImpl(String name, SourceInformation sourceInformation, CoreInstance classifier)
{
super(name, sourceInformation, classifier);
}
public static final CoreInstanceFactory FACTORY = new BaseJavaModelCoreInstanceFactory()
{
@Override
public CoreInstance createCoreInstance(String name, int internalSyntheticId, SourceInformation sourceInformation, CoreInstance classifier, ModelRepository repository, boolean persistent)
{
return new Package_LazyImpl(name, sourceInformation, classifier);
}
@Override
public boolean supports(String classifierPath)
{
return tempFullTypeId.equals(classifierPath);
}
};
@Override
public RichIterable getKeys()
{
return KEY_INDEX.getKeys();
}
@Override
public ListIterable getRealKeyByName(String name)
{
return KEY_INDEX.getRealKeyByName(name);
}
@Override
public CoreInstance getValueForMetaPropertyToOne(String keyName)
{
switch (keyName)
{
case "classifierGenericType":
{
return ValCoreInstance.toCoreInstance(_classifierGenericType());
}
case "elementOverride":
{
return ValCoreInstance.toCoreInstance(_elementOverride());
}
case "name":
{
return ValCoreInstance.toCoreInstance(_name());
}
case "package":
{
return ValCoreInstance.toCoreInstance(_package());
}
default:
{
return super.getValueForMetaPropertyToOne(keyName);
}
}
}
@Override
public ListIterable getValueForMetaPropertyToMany(String keyName)
{
switch (keyName)
{
case "children":
{
return ValCoreInstance.toCoreInstances(_children());
}
case "referenceUsages":
{
return ValCoreInstance.toCoreInstances(_referenceUsages());
}
case "stereotypes":
{
return ValCoreInstance.toCoreInstances(_stereotypes());
}
case "taggedValues":
{
return ValCoreInstance.toCoreInstances(_taggedValues());
}
default:
{
return super.getValueForMetaPropertyToMany(keyName);
}
}
}
public final AtomicBoolean _taggedValues_initialized = new AtomicBoolean(false);
public RichIterable _taggedValues = Lists.mutable.with();
public void _reverse_taggedValues(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
this._taggedValues();
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).add(val);
}
public void _sever_reverse_taggedValues(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
this._taggedValues();
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).remove(val);
}
private org.finos.legend.pure.m3.coreinstance.Package _taggedValues(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val, boolean add)
{
this._taggedValues();
if (val == null)
{
if (!add)
{
this._taggedValues = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).add(val);
}
else
{
this._taggedValues = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _taggedValues(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val, boolean add)
{
this._taggedValues();
if (add)
{
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).addAllIterable(val);
}
else
{
this._taggedValues = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValues(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val)
{
return this._taggedValues(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
return this._taggedValues(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> val)
{
return this._taggedValues(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesRemove()
{
this._taggedValues();
this._taggedValues = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _taggedValuesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue val)
{
this._taggedValues();
if (!(this._taggedValues instanceof MutableList))
{
this._taggedValues = this._taggedValues.toList();
}
((MutableList)this._taggedValues).remove(val);
return this;
}
public RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.TaggedValue> _taggedValues()
{
if (!this._taggedValues_initialized.get())
{
synchronized (this._taggedValues_initialized)
{
if (!this._taggedValues_initialized.get())
{
this._taggedValues = loadValuesFromMetadata("taggedValues");
this._taggedValues_initialized.set(true);
}
}
}
return this._taggedValues;
}
public final AtomicBoolean _elementOverride_initialized = new AtomicBoolean(false);
public org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride _elementOverride;
public void _reverse_elementOverride(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride val)
{
this._elementOverride();
this._elementOverride = val;
}
public void _sever_reverse_elementOverride(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride val)
{
this._elementOverride();
this._elementOverride = null;
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverride(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride val)
{
this._elementOverride();
this._elementOverride = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverride(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride> val)
{
return _elementOverride(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _elementOverrideRemove()
{
this._elementOverride();
this._elementOverride = null;
return this;
}
public org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride _elementOverride()
{
if (!this._elementOverride_initialized.get())
{
synchronized (this._elementOverride_initialized)
{
if (!this._elementOverride_initialized.get())
{
this._elementOverride = loadValueFromMetadata("elementOverride");
this._elementOverride_initialized.set(true);
}
}
}
return this._elementOverride;
}
public final AtomicBoolean _stereotypes_initialized = new AtomicBoolean(false);
public RichIterable _stereotypes = Lists.mutable.with();
public void _reverse_stereotypes(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
this._stereotypes();
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).add(val);
}
public void _sever_reverse_stereotypes(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
this._stereotypes();
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).remove(val);
}
private org.finos.legend.pure.m3.coreinstance.Package _stereotypes(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val, boolean add)
{
this._stereotypes();
if (val == null)
{
if (!add)
{
this._stereotypes = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).add(val);
}
else
{
this._stereotypes = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _stereotypes(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val, boolean add)
{
this._stereotypes();
if (add)
{
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).addAllIterable(val);
}
else
{
this._stereotypes = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypes(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val)
{
return this._stereotypes(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
return this._stereotypes(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> val)
{
return this._stereotypes(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemove()
{
this._stereotypes();
this._stereotypes = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype val)
{
this._stereotypes();
if (!(this._stereotypes instanceof MutableList))
{
this._stereotypes = this._stereotypes.toList();
}
((MutableList)this._stereotypes).remove(val);
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddCoreInstance(CoreInstance val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesAddAllCoreInstance(RichIterable extends CoreInstance> val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesCoreInstance(RichIterable extends org.finos.legend.pure.m4.coreinstance.CoreInstance> val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public org.finos.legend.pure.m3.coreinstance.Package _stereotypesRemoveCoreInstance(CoreInstance val)
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public RichIterable _stereotypesCoreInstance()
{
throw new UnsupportedOperationException("Not supported in Compiled Mode at this time");
}
public RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.extension.Stereotype> _stereotypes()
{
if (!this._stereotypes_initialized.get())
{
synchronized (this._stereotypes_initialized)
{
if (!this._stereotypes_initialized.get())
{
this._stereotypes = loadValuesFromMetadata("stereotypes");
this._stereotypes_initialized.set(true);
}
}
}
return this._stereotypes;
}
public final AtomicBoolean _name_initialized = new AtomicBoolean(false);
public java.lang.String _name;
public org.finos.legend.pure.m3.coreinstance.Package _name(java.lang.String val)
{
this._name();
this._name = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _name(RichIterable extends java.lang.String> val)
{
return _name(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _nameRemove()
{
this._name();
this._name = null;
return this;
}
public java.lang.String _name()
{
if (!this._name_initialized.get())
{
synchronized (this._name_initialized)
{
if (!this._name_initialized.get())
{
this._name = loadValueFromMetadata("name");
this._name_initialized.set(true);
}
}
}
return this._name;
}
public final AtomicBoolean _package_initialized = new AtomicBoolean(false);
public org.finos.legend.pure.m3.coreinstance.Package _package;
public void _reverse_package(org.finos.legend.pure.m3.coreinstance.Package val)
{
this._package();
this._package = val;
}
public void _sever_reverse_package(org.finos.legend.pure.m3.coreinstance.Package val)
{
this._package();
this._package = null;
}
public org.finos.legend.pure.m3.coreinstance.Package _package(org.finos.legend.pure.m3.coreinstance.Package val)
{
this._package();
this._package = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _package(RichIterable extends org.finos.legend.pure.m3.coreinstance.Package> val)
{
return _package(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _packageRemove()
{
this._package();
this._package = null;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _package()
{
if (!this._package_initialized.get())
{
synchronized (this._package_initialized)
{
if (!this._package_initialized.get())
{
this._package = loadValueFromMetadata("package");
this._package_initialized.set(true);
}
}
}
return this._package;
}
public final AtomicBoolean _children_initialized = new AtomicBoolean(false);
public RichIterable _children = Lists.mutable.with();
public void _reverse_children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
this._children();
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).add(val);
}
public void _sever_reverse_children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
this._children();
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).remove(val);
}
private org.finos.legend.pure.m3.coreinstance.Package _children(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val, boolean add)
{
this._children();
if (val == null)
{
if (!add)
{
this._children = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).add(val);
}
else
{
this._children = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _children(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val, boolean add)
{
this._children();
if (add)
{
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).addAllIterable(val);
}
else
{
this._children = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _children(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val)
{
return this._children(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
return this._children(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> val)
{
return this._children(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenRemove()
{
this._children();
this._children = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _childrenRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement val)
{
this._children();
if (!(this._children instanceof MutableList))
{
this._children = this._children.toList();
}
((MutableList)this._children).remove(val);
return this;
}
public RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.PackageableElement> _children()
{
if (!this._children_initialized.get())
{
synchronized (this._children_initialized)
{
if (!this._children_initialized.get())
{
this._children = loadValuesFromMetadata("children");
this._children_initialized.set(true);
}
}
}
return this._children;
}
public final AtomicBoolean _referenceUsages_initialized = new AtomicBoolean(false);
public RichIterable _referenceUsages = Lists.mutable.with();
public void _reverse_referenceUsages(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
this._referenceUsages();
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).add(val);
}
public void _sever_reverse_referenceUsages(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
this._referenceUsages();
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).remove(val);
}
private org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val, boolean add)
{
this._referenceUsages();
if (val == null)
{
if (!add)
{
this._referenceUsages = Lists.mutable.with();
}
return this;
}
if (add)
{
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).add(val);
}
else
{
this._referenceUsages = (val == null ? Lists.mutable.empty() : Lists.mutable.with(val));
}
return this;
}
private org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val, boolean add)
{
this._referenceUsages();
if (add)
{
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).addAllIterable(val);
}
else
{
this._referenceUsages = val;
}
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsages(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val)
{
return this._referenceUsages(val, false);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesAdd(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
return this._referenceUsages(Lists.immutable.with(val), true);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesAddAll(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> val)
{
return this._referenceUsages(val, true);
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesRemove()
{
this._referenceUsages();
this._referenceUsages = Lists.mutable.empty();
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _referenceUsagesRemove(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage val)
{
this._referenceUsages();
if (!(this._referenceUsages instanceof MutableList))
{
this._referenceUsages = this._referenceUsages.toList();
}
((MutableList)this._referenceUsages).remove(val);
return this;
}
public RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.ReferenceUsage> _referenceUsages()
{
if (!this._referenceUsages_initialized.get())
{
synchronized (this._referenceUsages_initialized)
{
if (!this._referenceUsages_initialized.get())
{
this._referenceUsages = loadValuesFromMetadata("referenceUsages");
this._referenceUsages_initialized.set(true);
}
}
}
return this._referenceUsages;
}
public final AtomicBoolean _classifierGenericType_initialized = new AtomicBoolean(false);
public org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType _classifierGenericType;
public void _reverse_classifierGenericType(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType val)
{
this._classifierGenericType();
this._classifierGenericType = val;
}
public void _sever_reverse_classifierGenericType(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType val)
{
this._classifierGenericType();
this._classifierGenericType = null;
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericType(org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType val)
{
this._classifierGenericType();
this._classifierGenericType = val;
return this;
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericType(RichIterable extends org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType> val)
{
return _classifierGenericType(val.getFirst());
}
public org.finos.legend.pure.m3.coreinstance.Package _classifierGenericTypeRemove()
{
this._classifierGenericType();
this._classifierGenericType = null;
return this;
}
public org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType _classifierGenericType()
{
if (!this._classifierGenericType_initialized.get())
{
synchronized (this._classifierGenericType_initialized)
{
if (!this._classifierGenericType_initialized.get())
{
this._classifierGenericType = loadValueFromMetadata("classifierGenericType");
this._classifierGenericType_initialized.set(true);
}
}
}
return this._classifierGenericType;
}
public org.finos.legend.pure.m3.coreinstance.Package copy()
{
return new Package_LazyImpl(this);
}
public Package_LazyImpl(org.finos.legend.pure.m3.coreinstance.Package src)
{
super((Package_LazyImpl)src);
synchronized (((Package_LazyImpl)src)._taggedValues_initialized)
{
this._taggedValues = FastList.newList(((Package_LazyImpl)src)._taggedValues);
this._taggedValues_initialized.set(((Package_LazyImpl)src)._taggedValues_initialized.get());
}
synchronized (((Package_LazyImpl)src)._elementOverride_initialized)
{
this._elementOverride = (org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.ElementOverride)((Package_LazyImpl)src)._elementOverride;
this._elementOverride_initialized.set(((Package_LazyImpl)src)._elementOverride_initialized.get());
}
synchronized (((Package_LazyImpl)src)._stereotypes_initialized)
{
this._stereotypes = FastList.newList(((Package_LazyImpl)src)._stereotypes);
this._stereotypes_initialized.set(((Package_LazyImpl)src)._stereotypes_initialized.get());
}
synchronized (((Package_LazyImpl)src)._name_initialized)
{
this._name = (java.lang.String)((Package_LazyImpl)src)._name;
this._name_initialized.set(((Package_LazyImpl)src)._name_initialized.get());
}
synchronized (((Package_LazyImpl)src)._package_initialized)
{
this._package = (org.finos.legend.pure.m3.coreinstance.Package)((Package_LazyImpl)src)._package;
this._package_initialized.set(((Package_LazyImpl)src)._package_initialized.get());
}
synchronized (((Package_LazyImpl)src)._children_initialized)
{
this._children = FastList.newList(((Package_LazyImpl)src)._children);
this._children_initialized.set(((Package_LazyImpl)src)._children_initialized.get());
}
synchronized (((Package_LazyImpl)src)._referenceUsages_initialized)
{
this._referenceUsages = FastList.newList(((Package_LazyImpl)src)._referenceUsages);
this._referenceUsages_initialized.set(((Package_LazyImpl)src)._referenceUsages_initialized.get());
}
synchronized (((Package_LazyImpl)src)._classifierGenericType_initialized)
{
this._classifierGenericType = (org.finos.legend.pure.m3.coreinstance.meta.pure.metamodel.type.generics.GenericType)((Package_LazyImpl)src)._classifierGenericType;
this._classifierGenericType_initialized.set(((Package_LazyImpl)src)._classifierGenericType_initialized.get());
}
}
@Override
public String getFullSystemPath()
{
return tempFullTypeId;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy