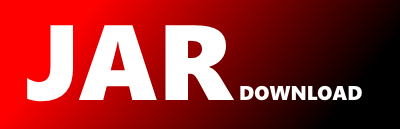
com.symphony.api.agent.SignalsApi Maven / Gradle / Ivy
package com.symphony.api.agent;
import com.symphony.api.model.AgentInfo;
import com.symphony.api.model.BaseSignal;
import com.symphony.api.model.ChannelSubscriberResponse;
import com.symphony.api.model.ChannelSubscriptionResponse;
import com.symphony.api.model.Signal;
import com.symphony.api.model.SignalList;
import com.symphony.api.model.SuccessResponse;
import com.symphony.api.model.V2Error;
import java.io.InputStream;
import java.io.OutputStream;
import java.util.List;
import java.util.Map;
import javax.ws.rs.*;
import javax.ws.rs.core.Response;
import javax.ws.rs.core.MediaType;
import org.apache.cxf.jaxrs.ext.multipart.*;
import io.swagger.v3.oas.annotations.Operation;
import io.swagger.v3.oas.annotations.responses.ApiResponses;
import io.swagger.v3.oas.annotations.responses.ApiResponse;
import io.swagger.v3.oas.annotations.media.ArraySchema;
import io.swagger.v3.oas.annotations.media.Content;
import io.swagger.v3.oas.annotations.media.Schema;
/**
* Agent API
*
* This document refers to Symphony API calls to send and receive messages and content. They need the on-premise Agent installed to perform decryption/encryption of content. - sessionToken and keyManagerToken can be obtained by calling the authenticationAPI on the symphony back end and the key manager respectively. Refer to the methods described in authenticatorAPI.yaml. - Actions are defined to be atomic, ie will succeed in their entirety or fail and have changed nothing. - If it returns a 40X status then it will have sent no message to any stream even if a request to aome subset of the requested streams would have succeeded. - If this contract cannot be met for any reason then this is an error and the response code will be 50X. - MessageML is a markup language for messages. See reference here: https://rest-api.symphony.com/docs/messagemlv2 - **Real Time Events**: The following events are returned when reading from a real time messages and events stream (\"datafeed\"). These events will be returned for datafeeds created with the v5 endpoints. To know more about the endpoints, refer to Create Messages/Events Stream and Read Messages/Events Stream. Unless otherwise specified, all events were added in 1.46.
*
*/
@Path("/")
public interface SignalsApi {
/**
* Get information about the Agent
*
*/
@GET
@Path("/v1/info")
@Produces({ "application/json" })
@Operation(summary = "Get information about the Agent", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Agent info.", content = @Content(schema = @Schema(implementation = AgentInfo.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public AgentInfo v1InfoGet();
/**
* Create a signal.
*
*/
@POST
@Path("/v1/signals/create")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Create a signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal created.", content = @Content(schema = @Schema(implementation = Signal.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "451", description = "Compliance Issues found in signal", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public Signal v1SignalsCreatePost(BaseSignal body, @HeaderParam("sessionToken") String sessionToken, @HeaderParam("keyManagerToken") String keyManagerToken);
/**
* Delete a signal.
*
*/
@POST
@Path("/v1/signals/{id}/delete")
@Produces({ "application/json" })
@Operation(summary = "Delete a signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal deleted.", content = @Content(schema = @Schema(implementation = SuccessResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public SuccessResponse v1SignalsIdDeletePost(@HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, @HeaderParam("keyManagerToken") String keyManagerToken);
/**
* Get details of the requested signal.
*
*/
@GET
@Path("/v1/signals/{id}/get")
@Produces({ "application/json" })
@Operation(summary = "Get details of the requested signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "List of signals for the requesting user.", content = @Content(schema = @Schema(implementation = Signal.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public Signal v1SignalsIdGetGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, @HeaderParam("keyManagerToken") String keyManagerToken);
/**
* Subscribe to a Signal.
*
*/
@POST
@Path("/v1/signals/{id}/subscribe")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Subscribe to a Signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal subscribed.", content = @Content(schema = @Schema(implementation = ChannelSubscriptionResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public ChannelSubscriptionResponse v1SignalsIdSubscribePost(@HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, List body, @HeaderParam("keyManagerToken") String keyManagerToken, @QueryParam("pushed")Boolean pushed);
/**
* Get the subscribers of a signal
*
*/
@GET
@Path("/v1/signals/{id}/subscribers")
@Produces({ "application/json" })
@Operation(summary = "Get the subscribers of a signal", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal Subscribers.", content = @Content(schema = @Schema(implementation = ChannelSubscriberResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public ChannelSubscriberResponse v1SignalsIdSubscribersGet(@HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, @HeaderParam("keyManagerToken") String keyManagerToken, @QueryParam("skip")@DefaultValue("0") Integer skip, @QueryParam("limit")@DefaultValue("100") Integer limit);
/**
* Unsubscribe to a Signal.
*
*/
@POST
@Path("/v1/signals/{id}/unsubscribe")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Unsubscribe to a Signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal unsubscribed.", content = @Content(schema = @Schema(implementation = ChannelSubscriptionResponse.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public ChannelSubscriptionResponse v1SignalsIdUnsubscribePost(@HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, List body, @HeaderParam("keyManagerToken") String keyManagerToken);
/**
* Update a signal.
*
*/
@POST
@Path("/v1/signals/{id}/update")
@Consumes({ "application/json" })
@Produces({ "application/json" })
@Operation(summary = "Update a signal.", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "Signal updated.", content = @Content(schema = @Schema(implementation = Signal.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "451", description = "Compliance Issues found in signal", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public Signal v1SignalsIdUpdatePost(BaseSignal body, @HeaderParam("sessionToken") String sessionToken, @PathParam("id") String id, @HeaderParam("keyManagerToken") String keyManagerToken);
/**
* List signals for the requesting user. This includes signals that the user has created and public signals to which they subscribed.
*
*/
@GET
@Path("/v1/signals/list")
@Produces({ "application/json" })
@Operation(summary = "List signals for the requesting user. This includes signals that the user has created and public signals to which they subscribed. ", tags={ })
@ApiResponses(value = {
@ApiResponse(responseCode = "200", description = "List of signals for the requesting user.", content = @Content(schema = @Schema(implementation = SignalList.class))),
@ApiResponse(responseCode = "400", description = "Client error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "401", description = "Unauthorized: Session tokens invalid.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "403", description = "Forbidden: Caller lacks necessary entitlement.", content = @Content(schema = @Schema(implementation = V2Error.class))),
@ApiResponse(responseCode = "500", description = "Server error, see response body for further details.", content = @Content(schema = @Schema(implementation = V2Error.class))) })
public SignalList v1SignalsListGet(@HeaderParam("sessionToken") String sessionToken, @HeaderParam("keyManagerToken") String keyManagerToken, @QueryParam("skip")Integer skip, @QueryParam("limit")Integer limit);
}