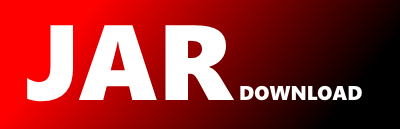
com.symphony.api.model.AckId Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* An object containing the ackId (and parameters) associated with events that the client has received through an individual feed.
**/
@Schema(description="An object containing the ackId (and parameters) associated with events that the client has received through an individual feed.")
public class AckId {
@Schema(description = "A unique id for events that can be deleted from a client's. Empty for the first read. feed.")
/**
* A unique id for events that can be deleted from a client's. Empty for the first read. feed.
**/
private String ackId = null;
@Schema(description = "Set to false to avoid updating the user's presence when reading events. Default is true.")
/**
* Set to false to avoid updating the user's presence when reading events. Default is true.
**/
private Boolean updatePresence = true;
/**
* A unique id for events that can be deleted from a client's. Empty for the first read. feed.
* @return ackId
**/
@JsonProperty("ackId")
public String getAckId() {
return ackId;
}
public void setAckId(String ackId) {
this.ackId = ackId;
}
public AckId ackId(String ackId) {
this.ackId = ackId;
return this;
}
/**
* Set to false to avoid updating the user's presence when reading events. Default is true.
* @return updatePresence
**/
@JsonProperty("updatePresence")
public Boolean isUpdatePresence() {
return updatePresence;
}
public void setUpdatePresence(Boolean updatePresence) {
this.updatePresence = updatePresence;
}
public AckId updatePresence(Boolean updatePresence) {
this.updatePresence = updatePresence;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AckId {\n");
sb.append(" ackId: ").append(toIndentedString(ackId)).append("\n");
sb.append(" updatePresence: ").append(toIndentedString(updatePresence)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy