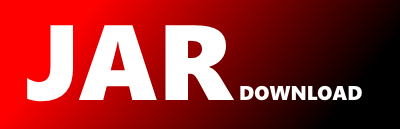
com.symphony.api.model.AdminStreamAttributes Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* additional optional properties for the stream
**/
@Schema(description="additional optional properties for the stream")
public class AdminStreamAttributes {
@Schema(description = "room name (room only)")
/**
* room name (room only)
**/
private String roomName = null;
@Schema(description = "description of the room (room only)")
/**
* description of the room (room only)
**/
private String roomDescription = null;
@Schema(description = "list of userid who is member of the stream - im or mim only")
/**
* list of userid who is member of the stream - im or mim only
**/
private List members = null;
@Schema(description = "creator user id")
/**
* creator user id
**/
private Long createdByUserId = null;
@Schema(description = "created date")
/**
* created date
**/
private Long createdDate = null;
@Schema(description = "last modified date")
/**
* last modified date
**/
private Long lastModifiedDate = null;
@Schema(description = "company name of the creator")
/**
* company name of the creator
**/
private String originCompany = null;
@Schema(description = "company id of the creator")
/**
* company id of the creator
**/
private Integer originCompanyId = null;
@Schema(description = "total number of members in the stream")
/**
* total number of members in the stream
**/
private Integer membersCount = null;
/**
* room name (room only)
* @return roomName
**/
@JsonProperty("roomName")
public String getRoomName() {
return roomName;
}
public void setRoomName(String roomName) {
this.roomName = roomName;
}
public AdminStreamAttributes roomName(String roomName) {
this.roomName = roomName;
return this;
}
/**
* description of the room (room only)
* @return roomDescription
**/
@JsonProperty("roomDescription")
public String getRoomDescription() {
return roomDescription;
}
public void setRoomDescription(String roomDescription) {
this.roomDescription = roomDescription;
}
public AdminStreamAttributes roomDescription(String roomDescription) {
this.roomDescription = roomDescription;
return this;
}
/**
* list of userid who is member of the stream - im or mim only
* @return members
**/
@JsonProperty("members")
public List getMembers() {
return members;
}
public void setMembers(List members) {
this.members = members;
}
public AdminStreamAttributes members(List members) {
this.members = members;
return this;
}
public AdminStreamAttributes addMembersItem(Long membersItem) {
this.members.add(membersItem);
return this;
}
/**
* creator user id
* @return createdByUserId
**/
@JsonProperty("createdByUserId")
public Long getCreatedByUserId() {
return createdByUserId;
}
public void setCreatedByUserId(Long createdByUserId) {
this.createdByUserId = createdByUserId;
}
public AdminStreamAttributes createdByUserId(Long createdByUserId) {
this.createdByUserId = createdByUserId;
return this;
}
/**
* created date
* @return createdDate
**/
@JsonProperty("createdDate")
public Long getCreatedDate() {
return createdDate;
}
public void setCreatedDate(Long createdDate) {
this.createdDate = createdDate;
}
public AdminStreamAttributes createdDate(Long createdDate) {
this.createdDate = createdDate;
return this;
}
/**
* last modified date
* @return lastModifiedDate
**/
@JsonProperty("lastModifiedDate")
public Long getLastModifiedDate() {
return lastModifiedDate;
}
public void setLastModifiedDate(Long lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
}
public AdminStreamAttributes lastModifiedDate(Long lastModifiedDate) {
this.lastModifiedDate = lastModifiedDate;
return this;
}
/**
* company name of the creator
* @return originCompany
**/
@JsonProperty("originCompany")
public String getOriginCompany() {
return originCompany;
}
public void setOriginCompany(String originCompany) {
this.originCompany = originCompany;
}
public AdminStreamAttributes originCompany(String originCompany) {
this.originCompany = originCompany;
return this;
}
/**
* company id of the creator
* @return originCompanyId
**/
@JsonProperty("originCompanyId")
public Integer getOriginCompanyId() {
return originCompanyId;
}
public void setOriginCompanyId(Integer originCompanyId) {
this.originCompanyId = originCompanyId;
}
public AdminStreamAttributes originCompanyId(Integer originCompanyId) {
this.originCompanyId = originCompanyId;
return this;
}
/**
* total number of members in the stream
* @return membersCount
**/
@JsonProperty("membersCount")
public Integer getMembersCount() {
return membersCount;
}
public void setMembersCount(Integer membersCount) {
this.membersCount = membersCount;
}
public AdminStreamAttributes membersCount(Integer membersCount) {
this.membersCount = membersCount;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AdminStreamAttributes {\n");
sb.append(" roomName: ").append(toIndentedString(roomName)).append("\n");
sb.append(" roomDescription: ").append(toIndentedString(roomDescription)).append("\n");
sb.append(" members: ").append(toIndentedString(members)).append("\n");
sb.append(" createdByUserId: ").append(toIndentedString(createdByUserId)).append("\n");
sb.append(" createdDate: ").append(toIndentedString(createdDate)).append("\n");
sb.append(" lastModifiedDate: ").append(toIndentedString(lastModifiedDate)).append("\n");
sb.append(" originCompany: ").append(toIndentedString(originCompany)).append("\n");
sb.append(" originCompanyId: ").append(toIndentedString(originCompanyId)).append("\n");
sb.append(" membersCount: ").append(toIndentedString(membersCount)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy