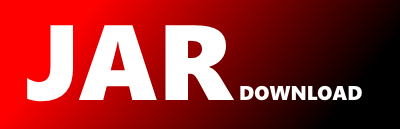
com.symphony.api.model.AgentInfo Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class AgentInfo {
@Schema(description = "The IP address of the Agent server.")
/**
* The IP address of the Agent server.
**/
private String ipAddress = null;
@Schema(description = "The hostname of the Agent server.")
/**
* The hostname of the Agent server.
**/
private String hostname = null;
@Schema(description = "The fully-qualified domain name of the Agent server. Must be set by the user at startup.")
/**
* The fully-qualified domain name of the Agent server. Must be set by the user at startup.
**/
private String serverFqdn = null;
@Schema(description = "The version of the Agent.")
/**
* The version of the Agent.
**/
private String version = null;
@Schema(description = "The URL under which the Agent is available.")
/**
* The URL under which the Agent is available.
**/
private String url = null;
@Schema(description = "Whether this is an on-prem or cloud installation.")
/**
* Whether this is an on-prem or cloud installation.
**/
private Boolean onPrem = null;
@Schema(description = "The Git commit ID of the running revision.")
/**
* The Git commit ID of the running revision.
**/
private String commitId = null;
/**
* The IP address of the Agent server.
* @return ipAddress
**/
@JsonProperty("ipAddress")
public String getIpAddress() {
return ipAddress;
}
public void setIpAddress(String ipAddress) {
this.ipAddress = ipAddress;
}
public AgentInfo ipAddress(String ipAddress) {
this.ipAddress = ipAddress;
return this;
}
/**
* The hostname of the Agent server.
* @return hostname
**/
@JsonProperty("hostname")
public String getHostname() {
return hostname;
}
public void setHostname(String hostname) {
this.hostname = hostname;
}
public AgentInfo hostname(String hostname) {
this.hostname = hostname;
return this;
}
/**
* The fully-qualified domain name of the Agent server. Must be set by the user at startup.
* @return serverFqdn
**/
@JsonProperty("serverFqdn")
public String getServerFqdn() {
return serverFqdn;
}
public void setServerFqdn(String serverFqdn) {
this.serverFqdn = serverFqdn;
}
public AgentInfo serverFqdn(String serverFqdn) {
this.serverFqdn = serverFqdn;
return this;
}
/**
* The version of the Agent.
* @return version
**/
@JsonProperty("version")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public AgentInfo version(String version) {
this.version = version;
return this;
}
/**
* The URL under which the Agent is available.
* @return url
**/
@JsonProperty("url")
public String getUrl() {
return url;
}
public void setUrl(String url) {
this.url = url;
}
public AgentInfo url(String url) {
this.url = url;
return this;
}
/**
* Whether this is an on-prem or cloud installation.
* @return onPrem
**/
@JsonProperty("onPrem")
public Boolean isOnPrem() {
return onPrem;
}
public void setOnPrem(Boolean onPrem) {
this.onPrem = onPrem;
}
public AgentInfo onPrem(Boolean onPrem) {
this.onPrem = onPrem;
return this;
}
/**
* The Git commit ID of the running revision.
* @return commitId
**/
@JsonProperty("commitId")
public String getCommitId() {
return commitId;
}
public void setCommitId(String commitId) {
this.commitId = commitId;
}
public AgentInfo commitId(String commitId) {
this.commitId = commitId;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class AgentInfo {\n");
sb.append(" ipAddress: ").append(toIndentedString(ipAddress)).append("\n");
sb.append(" hostname: ").append(toIndentedString(hostname)).append("\n");
sb.append(" serverFqdn: ").append(toIndentedString(serverFqdn)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" url: ").append(toIndentedString(url)).append("\n");
sb.append(" onPrem: ").append(toIndentedString(onPrem)).append("\n");
sb.append(" commitId: ").append(toIndentedString(commitId)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy