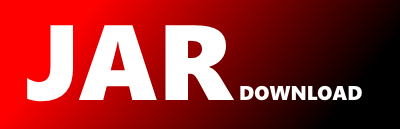
com.symphony.api.model.ApplicationInfo Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Required information for creating an application.
**/
@Schema(description="Required information for creating an application. ")
public class ApplicationInfo {
@Schema(description = "An unique id for the application.")
/**
* An unique id for the application.
**/
private String appId = null;
@Schema(description = "User defined name for the application.")
/**
* User defined name for the application.
**/
private String name = null;
@Schema(description = "The url for the app. Must start with \"https://\".")
/**
* The url for the app. Must start with \"https://\".
**/
private String appUrl = null;
@Schema(description = "Domain for app, that must match app url domain.")
/**
* Domain for app, that must match app url domain.
**/
private String domain = null;
@Schema(description = "The publisher for this application.")
/**
* The publisher for this application.
**/
private String publisher = null;
/**
* An unique id for the application.
* @return appId
**/
@JsonProperty("appId")
public String getAppId() {
return appId;
}
public void setAppId(String appId) {
this.appId = appId;
}
public ApplicationInfo appId(String appId) {
this.appId = appId;
return this;
}
/**
* User defined name for the application.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ApplicationInfo name(String name) {
this.name = name;
return this;
}
/**
* The url for the app. Must start with \"https://\".
* @return appUrl
**/
@JsonProperty("appUrl")
public String getAppUrl() {
return appUrl;
}
public void setAppUrl(String appUrl) {
this.appUrl = appUrl;
}
public ApplicationInfo appUrl(String appUrl) {
this.appUrl = appUrl;
return this;
}
/**
* Domain for app, that must match app url domain.
* @return domain
**/
@JsonProperty("domain")
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public ApplicationInfo domain(String domain) {
this.domain = domain;
return this;
}
/**
* The publisher for this application.
* @return publisher
**/
@JsonProperty("publisher")
public String getPublisher() {
return publisher;
}
public void setPublisher(String publisher) {
this.publisher = publisher;
}
public ApplicationInfo publisher(String publisher) {
this.publisher = publisher;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ApplicationInfo {\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" appUrl: ").append(toIndentedString(appUrl)).append("\n");
sb.append(" domain: ").append(toIndentedString(domain)).append("\n");
sb.append(" publisher: ").append(toIndentedString(publisher)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy