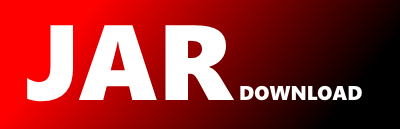
com.symphony.api.model.CompanyCertInfo Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class CompanyCertInfo {
@Schema(description = "Unique identifier")
/**
* Unique identifier
**/
private String fingerPrint = null;
@Schema(description = "Unique identifier of issuer cert if known")
/**
* Unique identifier of issuer cert if known
**/
private String issuerFingerPrint = null;
@Schema(description = "Date when we last saw this certificate presented")
/**
* Date when we last saw this certificate presented
**/
private Long lastSeen = null;
@Schema(description = "Date when this cert was last updated by administrator")
/**
* Date when this cert was last updated by administrator
**/
private Long updatedAt = null;
@Schema(description = "User ID of administrator who last updated this cert")
/**
* User ID of administrator who last updated this cert
**/
private Long updatedBy = null;
@Schema(description = "The Symphony account name which this certificate authenticates")
/**
* The Symphony account name which this certificate authenticates
**/
private String commonName = null;
@Schema(description = "Expiry date of this cert")
/**
* Expiry date of this cert
**/
private Long expiryDate = null;
/**
* Unique identifier
* @return fingerPrint
**/
@JsonProperty("fingerPrint")
public String getFingerPrint() {
return fingerPrint;
}
public void setFingerPrint(String fingerPrint) {
this.fingerPrint = fingerPrint;
}
public CompanyCertInfo fingerPrint(String fingerPrint) {
this.fingerPrint = fingerPrint;
return this;
}
/**
* Unique identifier of issuer cert if known
* @return issuerFingerPrint
**/
@JsonProperty("issuerFingerPrint")
public String getIssuerFingerPrint() {
return issuerFingerPrint;
}
public void setIssuerFingerPrint(String issuerFingerPrint) {
this.issuerFingerPrint = issuerFingerPrint;
}
public CompanyCertInfo issuerFingerPrint(String issuerFingerPrint) {
this.issuerFingerPrint = issuerFingerPrint;
return this;
}
/**
* Date when we last saw this certificate presented
* @return lastSeen
**/
@JsonProperty("lastSeen")
public Long getLastSeen() {
return lastSeen;
}
public void setLastSeen(Long lastSeen) {
this.lastSeen = lastSeen;
}
public CompanyCertInfo lastSeen(Long lastSeen) {
this.lastSeen = lastSeen;
return this;
}
/**
* Date when this cert was last updated by administrator
* @return updatedAt
**/
@JsonProperty("updatedAt")
public Long getUpdatedAt() {
return updatedAt;
}
public void setUpdatedAt(Long updatedAt) {
this.updatedAt = updatedAt;
}
public CompanyCertInfo updatedAt(Long updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* User ID of administrator who last updated this cert
* @return updatedBy
**/
@JsonProperty("updatedBy")
public Long getUpdatedBy() {
return updatedBy;
}
public void setUpdatedBy(Long updatedBy) {
this.updatedBy = updatedBy;
}
public CompanyCertInfo updatedBy(Long updatedBy) {
this.updatedBy = updatedBy;
return this;
}
/**
* The Symphony account name which this certificate authenticates
* @return commonName
**/
@JsonProperty("commonName")
public String getCommonName() {
return commonName;
}
public void setCommonName(String commonName) {
this.commonName = commonName;
}
public CompanyCertInfo commonName(String commonName) {
this.commonName = commonName;
return this;
}
/**
* Expiry date of this cert
* @return expiryDate
**/
@JsonProperty("expiryDate")
public Long getExpiryDate() {
return expiryDate;
}
public void setExpiryDate(Long expiryDate) {
this.expiryDate = expiryDate;
}
public CompanyCertInfo expiryDate(Long expiryDate) {
this.expiryDate = expiryDate;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class CompanyCertInfo {\n");
sb.append(" fingerPrint: ").append(toIndentedString(fingerPrint)).append("\n");
sb.append(" issuerFingerPrint: ").append(toIndentedString(issuerFingerPrint)).append("\n");
sb.append(" lastSeen: ").append(toIndentedString(lastSeen)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" updatedBy: ").append(toIndentedString(updatedBy)).append("\n");
sb.append(" commonName: ").append(toIndentedString(commonName)).append("\n");
sb.append(" expiryDate: ").append(toIndentedString(expiryDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy