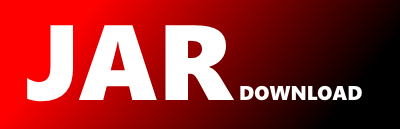
com.symphony.api.model.DelegateAction Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class DelegateAction {
@Schema(description = "")
private Long userId = null;
public enum ActionEnum {
ADD("ADD"),
REMOVE("REMOVE");
private String value;
ActionEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ActionEnum fromValue(String text) {
for (ActionEnum b : ActionEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "")
private ActionEnum action = null;
/**
* Get userId
* @return userId
**/
@JsonProperty("userId")
public Long getUserId() {
return userId;
}
public void setUserId(Long userId) {
this.userId = userId;
}
public DelegateAction userId(Long userId) {
this.userId = userId;
return this;
}
/**
* Get action
* @return action
**/
@JsonProperty("action")
public String getAction() {
if (action == null) {
return null;
}
return action.getValue();
}
public void setAction(ActionEnum action) {
this.action = action;
}
public DelegateAction action(ActionEnum action) {
this.action = action;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DelegateAction {\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy