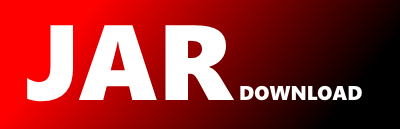
com.symphony.api.model.FileExtension Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
public class FileExtension {
@Schema(example = ".txt", required = true, description = "The file extension that this specific record is for. This is a unique ID that allows this record to be referenced in the URI. ")
/**
* The file extension that this specific record is for. This is a unique ID that allows this record to be referenced in the URI.
**/
private String extension = null;
@Schema(description = "File extension allowed for internal scope ")
/**
* File extension allowed for internal scope
**/
private Boolean scopeInternal = null;
@Schema(description = "File extension allowed for external scope ")
/**
* File extension allowed for external scope
**/
private Boolean scopeExternal = null;
public enum SourceEnum {
SYSTEM("SYSTEM"),
CUSTOMER("CUSTOMER");
private String value;
SourceEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SourceEnum fromValue(String text) {
for (SourceEnum b : SourceEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "File extension with metadata understood by the system or file extension created by a customer ")
/**
* File extension with metadata understood by the system or file extension created by a customer
**/
private SourceEnum source = null;
/**
* The file extension that this specific record is for. This is a unique ID that allows this record to be referenced in the URI.
* @return extension
**/
@JsonProperty("extension")
public String getExtension() {
return extension;
}
public void setExtension(String extension) {
this.extension = extension;
}
public FileExtension extension(String extension) {
this.extension = extension;
return this;
}
/**
* File extension allowed for internal scope
* @return scopeInternal
**/
@JsonProperty("scope_internal")
public Boolean isScopeInternal() {
return scopeInternal;
}
public void setScopeInternal(Boolean scopeInternal) {
this.scopeInternal = scopeInternal;
}
public FileExtension scopeInternal(Boolean scopeInternal) {
this.scopeInternal = scopeInternal;
return this;
}
/**
* File extension allowed for external scope
* @return scopeExternal
**/
@JsonProperty("scope_external")
public Boolean isScopeExternal() {
return scopeExternal;
}
public void setScopeExternal(Boolean scopeExternal) {
this.scopeExternal = scopeExternal;
}
public FileExtension scopeExternal(Boolean scopeExternal) {
this.scopeExternal = scopeExternal;
return this;
}
/**
* File extension with metadata understood by the system or file extension created by a customer
* @return source
**/
@JsonProperty("source")
public String getSource() {
if (source == null) {
return null;
}
return source.getValue();
}
public void setSource(SourceEnum source) {
this.source = source;
}
public FileExtension source(SourceEnum source) {
this.source = source;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class FileExtension {\n");
sb.append(" extension: ").append(toIndentedString(extension)).append("\n");
sb.append(" scopeInternal: ").append(toIndentedString(scopeInternal)).append("\n");
sb.append(" scopeExternal: ").append(toIndentedString(scopeExternal)).append("\n");
sb.append(" source: ").append(toIndentedString(source)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy