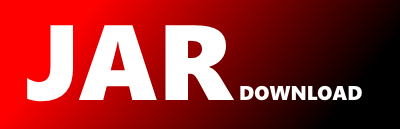
com.symphony.api.model.ImmutableRoomAttributes Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* These attributes cannot be changed once the room has been created
**/
@Schema(description="These attributes cannot be changed once the room has been created")
public class ImmutableRoomAttributes {
@Schema(description = "If true, this is a public chatroom. IF false, a private chatroom.")
/**
* If true, this is a public chatroom. IF false, a private chatroom.
**/
private Boolean _public = null;
@Schema(description = "If true, only stream owners can send messages.")
/**
* If true, only stream owners can send messages.
**/
private Boolean readOnly = null;
@Schema(description = "If true, clients disable the clipboard copy for content in this stream.")
/**
* If true, clients disable the clipboard copy for content in this stream.
**/
private Boolean copyProtected = null;
/**
* If true, this is a public chatroom. IF false, a private chatroom.
* @return _public
**/
@JsonProperty("public")
public Boolean isPublic() {
return _public;
}
public void setPublic(Boolean _public) {
this._public = _public;
}
public ImmutableRoomAttributes _public(Boolean _public) {
this._public = _public;
return this;
}
/**
* If true, only stream owners can send messages.
* @return readOnly
**/
@JsonProperty("readOnly")
public Boolean isReadOnly() {
return readOnly;
}
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
public ImmutableRoomAttributes readOnly(Boolean readOnly) {
this.readOnly = readOnly;
return this;
}
/**
* If true, clients disable the clipboard copy for content in this stream.
* @return copyProtected
**/
@JsonProperty("copyProtected")
public Boolean isCopyProtected() {
return copyProtected;
}
public void setCopyProtected(Boolean copyProtected) {
this.copyProtected = copyProtected;
}
public ImmutableRoomAttributes copyProtected(Boolean copyProtected) {
this.copyProtected = copyProtected;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class ImmutableRoomAttributes {\n");
sb.append(" _public: ").append(toIndentedString(_public)).append("\n");
sb.append(" readOnly: ").append(toIndentedString(readOnly)).append("\n");
sb.append(" copyProtected: ").append(toIndentedString(copyProtected)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy