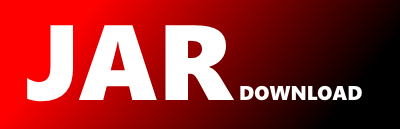
com.symphony.api.model.MessageReceiptDetail Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.DownloadReceiptCount;
import com.symphony.api.model.UserCompp;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Message receipt details response object
**/
@Schema(description="Message receipt details response object")
public class MessageReceiptDetail {
@Schema(description = "")
private UserCompp user = null;
@Schema(description = "Timestamp of message delivery receipts")
/**
* Timestamp of message delivery receipts
**/
private Long deliveryReceiptTimestamp = null;
@Schema(description = "Timestamp of message read receipts")
/**
* Timestamp of message read receipts
**/
private Long readReceiptTimestamp = null;
@Schema(description = "Timestamp of message email notifications")
/**
* Timestamp of message email notifications
**/
private Long emailNotificationTimestamp = null;
@Schema(description = "")
private List downloadReceiptCounts = null;
/**
* Get user
* @return user
**/
@JsonProperty("user")
public UserCompp getUser() {
return user;
}
public void setUser(UserCompp user) {
this.user = user;
}
public MessageReceiptDetail user(UserCompp user) {
this.user = user;
return this;
}
/**
* Timestamp of message delivery receipts
* @return deliveryReceiptTimestamp
**/
@JsonProperty("deliveryReceiptTimestamp")
public Long getDeliveryReceiptTimestamp() {
return deliveryReceiptTimestamp;
}
public void setDeliveryReceiptTimestamp(Long deliveryReceiptTimestamp) {
this.deliveryReceiptTimestamp = deliveryReceiptTimestamp;
}
public MessageReceiptDetail deliveryReceiptTimestamp(Long deliveryReceiptTimestamp) {
this.deliveryReceiptTimestamp = deliveryReceiptTimestamp;
return this;
}
/**
* Timestamp of message read receipts
* @return readReceiptTimestamp
**/
@JsonProperty("readReceiptTimestamp")
public Long getReadReceiptTimestamp() {
return readReceiptTimestamp;
}
public void setReadReceiptTimestamp(Long readReceiptTimestamp) {
this.readReceiptTimestamp = readReceiptTimestamp;
}
public MessageReceiptDetail readReceiptTimestamp(Long readReceiptTimestamp) {
this.readReceiptTimestamp = readReceiptTimestamp;
return this;
}
/**
* Timestamp of message email notifications
* @return emailNotificationTimestamp
**/
@JsonProperty("emailNotificationTimestamp")
public Long getEmailNotificationTimestamp() {
return emailNotificationTimestamp;
}
public void setEmailNotificationTimestamp(Long emailNotificationTimestamp) {
this.emailNotificationTimestamp = emailNotificationTimestamp;
}
public MessageReceiptDetail emailNotificationTimestamp(Long emailNotificationTimestamp) {
this.emailNotificationTimestamp = emailNotificationTimestamp;
return this;
}
/**
* Get downloadReceiptCounts
* @return downloadReceiptCounts
**/
@JsonProperty("downloadReceiptCounts")
public List getDownloadReceiptCounts() {
return downloadReceiptCounts;
}
public void setDownloadReceiptCounts(List downloadReceiptCounts) {
this.downloadReceiptCounts = downloadReceiptCounts;
}
public MessageReceiptDetail downloadReceiptCounts(List downloadReceiptCounts) {
this.downloadReceiptCounts = downloadReceiptCounts;
return this;
}
public MessageReceiptDetail addDownloadReceiptCountsItem(DownloadReceiptCount downloadReceiptCountsItem) {
this.downloadReceiptCounts.add(downloadReceiptCountsItem);
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MessageReceiptDetail {\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" deliveryReceiptTimestamp: ").append(toIndentedString(deliveryReceiptTimestamp)).append("\n");
sb.append(" readReceiptTimestamp: ").append(toIndentedString(readReceiptTimestamp)).append("\n");
sb.append(" emailNotificationTimestamp: ").append(toIndentedString(emailNotificationTimestamp)).append("\n");
sb.append(" downloadReceiptCounts: ").append(toIndentedString(downloadReceiptCounts)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy