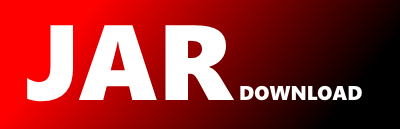
com.symphony.api.model.RoomSearchCriteria Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.UserId;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Room Query Object. Used to specify the parameters for room search.
**/
@Schema(description="Room Query Object. Used to specify the parameters for room search.")
public class RoomSearchCriteria {
@Schema(required = true, description = "The search query. Matches the room name and description.")
/**
* The search query. Matches the room name and description.
**/
private String query = null;
@Schema(description = "A list of room tag labels whose values will be queried.")
/**
* A list of room tag labels whose values will be queried.
**/
private List labels = null;
@Schema(description = "Restrict the search to active/inactive rooms. If unspecified, search all rooms.")
/**
* Restrict the search to active/inactive rooms. If unspecified, search all rooms.
**/
private Boolean active = null;
@Schema(description = "Restrict the search to private rooms. If unspecified, search all rooms.")
/**
* Restrict the search to private rooms. If unspecified, search all rooms.
**/
private Boolean _private = null;
@Schema(description = "")
private UserId owner = null;
@Schema(description = "")
private UserId creator = null;
@Schema(description = "")
private UserId member = null;
public enum SortOrderEnum {
BASIC("BASIC"),
RELEVANCE("RELEVANCE");
private String value;
SortOrderEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static SortOrderEnum fromValue(String text) {
for (SortOrderEnum b : SortOrderEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "Sort algorithm to be used. Supports two values: \"BASIC\" (legacy algorithm) and \"RELEVANCE\" (enhanced algorithm). ")
/**
* Sort algorithm to be used. Supports two values: \"BASIC\" (legacy algorithm) and \"RELEVANCE\" (enhanced algorithm).
**/
private SortOrderEnum sortOrder = null;
/**
* The search query. Matches the room name and description.
* @return query
**/
@JsonProperty("query")
public String getQuery() {
return query;
}
public void setQuery(String query) {
this.query = query;
}
public RoomSearchCriteria query(String query) {
this.query = query;
return this;
}
/**
* A list of room tag labels whose values will be queried.
* @return labels
**/
@JsonProperty("labels")
public List getLabels() {
return labels;
}
public void setLabels(List labels) {
this.labels = labels;
}
public RoomSearchCriteria labels(List labels) {
this.labels = labels;
return this;
}
public RoomSearchCriteria addLabelsItem(String labelsItem) {
this.labels.add(labelsItem);
return this;
}
/**
* Restrict the search to active/inactive rooms. If unspecified, search all rooms.
* @return active
**/
@JsonProperty("active")
public Boolean isActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public RoomSearchCriteria active(Boolean active) {
this.active = active;
return this;
}
/**
* Restrict the search to private rooms. If unspecified, search all rooms.
* @return _private
**/
@JsonProperty("private")
public Boolean isPrivate() {
return _private;
}
public void setPrivate(Boolean _private) {
this._private = _private;
}
public RoomSearchCriteria _private(Boolean _private) {
this._private = _private;
return this;
}
/**
* Get owner
* @return owner
**/
@JsonProperty("owner")
public UserId getOwner() {
return owner;
}
public void setOwner(UserId owner) {
this.owner = owner;
}
public RoomSearchCriteria owner(UserId owner) {
this.owner = owner;
return this;
}
/**
* Get creator
* @return creator
**/
@JsonProperty("creator")
public UserId getCreator() {
return creator;
}
public void setCreator(UserId creator) {
this.creator = creator;
}
public RoomSearchCriteria creator(UserId creator) {
this.creator = creator;
return this;
}
/**
* Get member
* @return member
**/
@JsonProperty("member")
public UserId getMember() {
return member;
}
public void setMember(UserId member) {
this.member = member;
}
public RoomSearchCriteria member(UserId member) {
this.member = member;
return this;
}
/**
* Sort algorithm to be used. Supports two values: \"BASIC\" (legacy algorithm) and \"RELEVANCE\" (enhanced algorithm).
* @return sortOrder
**/
@JsonProperty("sortOrder")
public String getSortOrder() {
if (sortOrder == null) {
return null;
}
return sortOrder.getValue();
}
public void setSortOrder(SortOrderEnum sortOrder) {
this.sortOrder = sortOrder;
}
public RoomSearchCriteria sortOrder(SortOrderEnum sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class RoomSearchCriteria {\n");
sb.append(" query: ").append(toIndentedString(query)).append("\n");
sb.append(" labels: ").append(toIndentedString(labels)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" _private: ").append(toIndentedString(_private)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" creator: ").append(toIndentedString(creator)).append("\n");
sb.append(" member: ").append(toIndentedString(member)).append("\n");
sb.append(" sortOrder: ").append(toIndentedString(sortOrder)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy