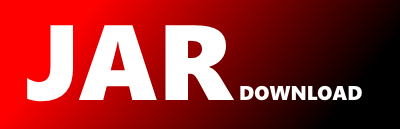
com.symphony.api.model.UserAppEntitlement Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.ProductList;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Application Entitlements for the user
**/
@Schema(description="Application Entitlements for the user")
public class UserAppEntitlement {
@Schema(required = true, description = "Unique ID for the Application")
/**
* Unique ID for the Application
**/
private String appId = null;
@Schema(description = "Name for this Application")
/**
* Name for this Application
**/
private String appName = null;
@Schema(required = true, description = "if true, this application will be listed in the appstore for this user. Otherwise, this application will be hidden in the appstore.")
/**
* if true, this application will be listed in the appstore for this user. Otherwise, this application will be hidden in the appstore.
**/
private Boolean listed = null;
@Schema(required = true, description = "if true, it indicate this application is installed for this user. Otherwise, this user does not have this application installed.")
/**
* if true, it indicate this application is installed for this user. Otherwise, this user does not have this application installed.
**/
private Boolean install = null;
@Schema(description = "")
private ProductList products = null;
/**
* Unique ID for the Application
* @return appId
**/
@JsonProperty("appId")
public String getAppId() {
return appId;
}
public void setAppId(String appId) {
this.appId = appId;
}
public UserAppEntitlement appId(String appId) {
this.appId = appId;
return this;
}
/**
* Name for this Application
* @return appName
**/
@JsonProperty("appName")
public String getAppName() {
return appName;
}
public void setAppName(String appName) {
this.appName = appName;
}
public UserAppEntitlement appName(String appName) {
this.appName = appName;
return this;
}
/**
* if true, this application will be listed in the appstore for this user. Otherwise, this application will be hidden in the appstore.
* @return listed
**/
@JsonProperty("listed")
public Boolean isListed() {
return listed;
}
public void setListed(Boolean listed) {
this.listed = listed;
}
public UserAppEntitlement listed(Boolean listed) {
this.listed = listed;
return this;
}
/**
* if true, it indicate this application is installed for this user. Otherwise, this user does not have this application installed.
* @return install
**/
@JsonProperty("install")
public Boolean isInstall() {
return install;
}
public void setInstall(Boolean install) {
this.install = install;
}
public UserAppEntitlement install(Boolean install) {
this.install = install;
return this;
}
/**
* Get products
* @return products
**/
@JsonProperty("products")
public ProductList getProducts() {
return products;
}
public void setProducts(ProductList products) {
this.products = products;
}
public UserAppEntitlement products(ProductList products) {
this.products = products;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserAppEntitlement {\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" appName: ").append(toIndentedString(appName)).append("\n");
sb.append(" listed: ").append(toIndentedString(listed)).append("\n");
sb.append(" install: ").append(toIndentedString(install)).append("\n");
sb.append(" products: ").append(toIndentedString(products)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy