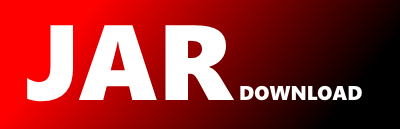
com.symphony.api.model.UserAppEntitlementPatch Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.Product;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Application Entitlements to patch for the user
**/
@Schema(description="Application Entitlements to patch for the user")
public class UserAppEntitlementPatch {
@Schema(required = true, description = "Unique ID for the Application")
/**
* Unique ID for the Application
**/
private String appId = null;
public enum ListedEnum {
TRUE("TRUE"),
FALSE("FALSE"),
KEEP("KEEP"),
REMOVE("REMOVE");
private String value;
ListedEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static ListedEnum fromValue(String text) {
for (ListedEnum b : ListedEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "If \"TRUE\", this application will be listed in the appstore for this user. If \"FALSE\", this application will be hidden in the appstore. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.")
/**
* If \"TRUE\", this application will be listed in the appstore for this user. If \"FALSE\", this application will be hidden in the appstore. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.
**/
private ListedEnum listed = ListedEnum.KEEP;
public enum InstallEnum {
TRUE("TRUE"),
FALSE("FALSE"),
KEEP("KEEP"),
REMOVE("REMOVE");
private String value;
InstallEnum(String value) {
this.value = value;
}
@JsonValue
public String getValue() {
return value;
}
@Override
public String toString() {
return String.valueOf(value);
}
@JsonCreator
public static InstallEnum fromValue(String text) {
for (InstallEnum b : InstallEnum.values()) {
if (String.valueOf(b.value).equals(text)) {
return b;
}
}
return null;
}
}
@Schema(description = "If \"TRUE\", this application will be installed for this user. If \"FALSE\", this application will not be installed for this user. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.")
/**
* If \"TRUE\", this application will be installed for this user. If \"FALSE\", this application will not be installed for this user. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.
**/
private InstallEnum install = InstallEnum.KEEP;
@Schema(description = "")
private Product product = null;
/**
* Unique ID for the Application
* @return appId
**/
@JsonProperty("appId")
public String getAppId() {
return appId;
}
public void setAppId(String appId) {
this.appId = appId;
}
public UserAppEntitlementPatch appId(String appId) {
this.appId = appId;
return this;
}
/**
* If \"TRUE\", this application will be listed in the appstore for this user. If \"FALSE\", this application will be hidden in the appstore. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.
* @return listed
**/
@JsonProperty("listed")
public String getListed() {
if (listed == null) {
return null;
}
return listed.getValue();
}
public void setListed(ListedEnum listed) {
this.listed = listed;
}
public UserAppEntitlementPatch listed(ListedEnum listed) {
this.listed = listed;
return this;
}
/**
* If \"TRUE\", this application will be installed for this user. If \"FALSE\", this application will not be installed for this user. If \"KEEP\" or not set, the current value is kept. If \"REMOVE\", it will be removed from user settings and the pod level setting's value will be used.
* @return install
**/
@JsonProperty("install")
public String getInstall() {
if (install == null) {
return null;
}
return install.getValue();
}
public void setInstall(InstallEnum install) {
this.install = install;
}
public UserAppEntitlementPatch install(InstallEnum install) {
this.install = install;
return this;
}
/**
* Get product
* @return product
**/
@JsonProperty("product")
public Product getProduct() {
return product;
}
public void setProduct(Product product) {
this.product = product;
}
public UserAppEntitlementPatch product(Product product) {
this.product = product;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserAppEntitlementPatch {\n");
sb.append(" appId: ").append(toIndentedString(appId)).append("\n");
sb.append(" listed: ").append(toIndentedString(listed)).append("\n");
sb.append(" install: ").append(toIndentedString(install)).append("\n");
sb.append(" product: ").append(toIndentedString(product)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy