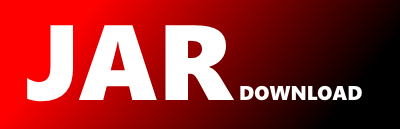
com.symphony.api.model.UserGroupAssignee Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.GroupRoleScope;
import com.symphony.api.model.UserCompp;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* User group assignee object
**/
@Schema(description="User group assignee object")
public class UserGroupAssignee {
@Schema(description = "Assignee id")
/**
* Assignee id
**/
private String id = null;
@Schema(description = "")
private String groupId = null;
@Schema(description = "")
private GroupRoleScope group = null;
@Schema(description = "")
private Long userId = null;
@Schema(description = "")
private UserCompp user = null;
@Schema(description = "")
private List userRoles = null;
@Schema(description = "")
private Boolean active = null;
@Schema(description = "Last added")
/**
* Last added
**/
private Long lastAddedDate = null;
@Schema(description = "Last removed")
/**
* Last removed
**/
private Long lastRemovedDate = null;
/**
* Assignee id
* @return id
**/
@JsonProperty("id")
public String getId() {
return id;
}
public void setId(String id) {
this.id = id;
}
public UserGroupAssignee id(String id) {
this.id = id;
return this;
}
/**
* Get groupId
* @return groupId
**/
@JsonProperty("groupId")
public String getGroupId() {
return groupId;
}
public void setGroupId(String groupId) {
this.groupId = groupId;
}
public UserGroupAssignee groupId(String groupId) {
this.groupId = groupId;
return this;
}
/**
* Get group
* @return group
**/
@JsonProperty("group")
public GroupRoleScope getGroup() {
return group;
}
public void setGroup(GroupRoleScope group) {
this.group = group;
}
public UserGroupAssignee group(GroupRoleScope group) {
this.group = group;
return this;
}
/**
* Get userId
* @return userId
**/
@JsonProperty("userId")
public Long getUserId() {
return userId;
}
public void setUserId(Long userId) {
this.userId = userId;
}
public UserGroupAssignee userId(Long userId) {
this.userId = userId;
return this;
}
/**
* Get user
* @return user
**/
@JsonProperty("user")
public UserCompp getUser() {
return user;
}
public void setUser(UserCompp user) {
this.user = user;
}
public UserGroupAssignee user(UserCompp user) {
this.user = user;
return this;
}
/**
* Get userRoles
* @return userRoles
**/
@JsonProperty("userRoles")
public List getUserRoles() {
return userRoles;
}
public void setUserRoles(List userRoles) {
this.userRoles = userRoles;
}
public UserGroupAssignee userRoles(List userRoles) {
this.userRoles = userRoles;
return this;
}
public UserGroupAssignee addUserRolesItem(String userRolesItem) {
this.userRoles.add(userRolesItem);
return this;
}
/**
* Get active
* @return active
**/
@JsonProperty("active")
public Boolean isActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public UserGroupAssignee active(Boolean active) {
this.active = active;
return this;
}
/**
* Last added
* @return lastAddedDate
**/
@JsonProperty("lastAddedDate")
public Long getLastAddedDate() {
return lastAddedDate;
}
public void setLastAddedDate(Long lastAddedDate) {
this.lastAddedDate = lastAddedDate;
}
public UserGroupAssignee lastAddedDate(Long lastAddedDate) {
this.lastAddedDate = lastAddedDate;
return this;
}
/**
* Last removed
* @return lastRemovedDate
**/
@JsonProperty("lastRemovedDate")
public Long getLastRemovedDate() {
return lastRemovedDate;
}
public void setLastRemovedDate(Long lastRemovedDate) {
this.lastRemovedDate = lastRemovedDate;
}
public UserGroupAssignee lastRemovedDate(Long lastRemovedDate) {
this.lastRemovedDate = lastRemovedDate;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class UserGroupAssignee {\n");
sb.append(" id: ").append(toIndentedString(id)).append("\n");
sb.append(" groupId: ").append(toIndentedString(groupId)).append("\n");
sb.append(" group: ").append(toIndentedString(group)).append("\n");
sb.append(" userId: ").append(toIndentedString(userId)).append("\n");
sb.append(" user: ").append(toIndentedString(user)).append("\n");
sb.append(" userRoles: ").append(toIndentedString(userRoles)).append("\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" lastAddedDate: ").append(toIndentedString(lastAddedDate)).append("\n");
sb.append(" lastRemovedDate: ").append(toIndentedString(lastRemovedDate)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy