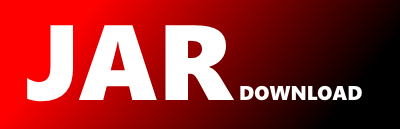
com.symphony.api.model.V1AuditTrailInitiatorResponse Maven / Gradle / Ivy
package com.symphony.api.model;
import io.swagger.v3.oas.annotations.media.Schema;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* Audit Trail Initiator object response. The attributes may vary according to the action. There are different types of action and each action could have specific attributes.
**/
@Schema(description="Audit Trail Initiator object response. The attributes may vary according to the action. There are different types of action and each action could have specific attributes. ")
public class V1AuditTrailInitiatorResponse {
@Schema(description = "The audit trail action that has peformed")
/**
* The audit trail action that has peformed
**/
private String action = null;
@Schema(description = "The audit trail action name that has peformed")
/**
* The audit trail action name that has peformed
**/
private String actionName = null;
@Schema(description = "The timestamp when the action has occurred")
/**
* The timestamp when the action has occurred
**/
private String timestamp = null;
@Schema(description = "The user's id that has performed the action")
/**
* The user's id that has performed the action
**/
private String initiatorId = null;
@Schema(description = "The username that has performed the action")
/**
* The username that has performed the action
**/
private String initiatorUsername = null;
@Schema(description = "The user's e-mail address that has performed the action")
/**
* The user's e-mail address that has performed the action
**/
private String initiatorEmailAddress = null;
/**
* The audit trail action that has peformed
* @return action
**/
@JsonProperty("action")
public String getAction() {
return action;
}
public void setAction(String action) {
this.action = action;
}
public V1AuditTrailInitiatorResponse action(String action) {
this.action = action;
return this;
}
/**
* The audit trail action name that has peformed
* @return actionName
**/
@JsonProperty("actionName")
public String getActionName() {
return actionName;
}
public void setActionName(String actionName) {
this.actionName = actionName;
}
public V1AuditTrailInitiatorResponse actionName(String actionName) {
this.actionName = actionName;
return this;
}
/**
* The timestamp when the action has occurred
* @return timestamp
**/
@JsonProperty("timestamp")
public String getTimestamp() {
return timestamp;
}
public void setTimestamp(String timestamp) {
this.timestamp = timestamp;
}
public V1AuditTrailInitiatorResponse timestamp(String timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* The user's id that has performed the action
* @return initiatorId
**/
@JsonProperty("initiatorId")
public String getInitiatorId() {
return initiatorId;
}
public void setInitiatorId(String initiatorId) {
this.initiatorId = initiatorId;
}
public V1AuditTrailInitiatorResponse initiatorId(String initiatorId) {
this.initiatorId = initiatorId;
return this;
}
/**
* The username that has performed the action
* @return initiatorUsername
**/
@JsonProperty("initiatorUsername")
public String getInitiatorUsername() {
return initiatorUsername;
}
public void setInitiatorUsername(String initiatorUsername) {
this.initiatorUsername = initiatorUsername;
}
public V1AuditTrailInitiatorResponse initiatorUsername(String initiatorUsername) {
this.initiatorUsername = initiatorUsername;
return this;
}
/**
* The user's e-mail address that has performed the action
* @return initiatorEmailAddress
**/
@JsonProperty("initiatorEmailAddress")
public String getInitiatorEmailAddress() {
return initiatorEmailAddress;
}
public void setInitiatorEmailAddress(String initiatorEmailAddress) {
this.initiatorEmailAddress = initiatorEmailAddress;
}
public V1AuditTrailInitiatorResponse initiatorEmailAddress(String initiatorEmailAddress) {
this.initiatorEmailAddress = initiatorEmailAddress;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1AuditTrailInitiatorResponse {\n");
sb.append(" action: ").append(toIndentedString(action)).append("\n");
sb.append(" actionName: ").append(toIndentedString(actionName)).append("\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" initiatorId: ").append(toIndentedString(initiatorId)).append("\n");
sb.append(" initiatorUsername: ").append(toIndentedString(initiatorUsername)).append("\n");
sb.append(" initiatorEmailAddress: ").append(toIndentedString(initiatorEmailAddress)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy