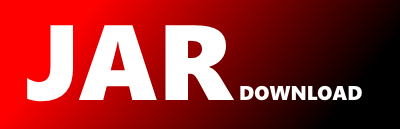
com.symphony.api.model.V1DLPPolicy Maven / Gradle / Ivy
package com.symphony.api.model;
import com.symphony.api.model.V1DLPDictionaryRef;
import io.swagger.v3.oas.annotations.media.Schema;
import java.util.ArrayList;
import java.util.List;
import io.swagger.v3.oas.annotations.media.Schema;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.XmlEnum;
import javax.xml.bind.annotation.XmlEnumValue;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonValue;
import com.fasterxml.jackson.annotation.JsonCreator;
/**
* The policy object for expression filter, one policy can have multiple dictionaries
**/
@Schema(description="The policy object for expression filter, one policy can have multiple dictionaries")
public class V1DLPPolicy {
@Schema(description = "Indicate whether the policy is active or not")
/**
* Indicate whether the policy is active or not
**/
private Boolean active = null;
@Schema(required = true, description = "The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified. ")
/**
* The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified.
**/
private List contentTypes = new ArrayList();
@Schema(description = "Creation time of the policy in milliseconds elapsed as of epoch time. ")
/**
* Creation time of the policy in milliseconds elapsed as of epoch time.
**/
private Long creationDate = null;
@Schema(description = "Numeric userId of the creator")
/**
* Numeric userId of the creator
**/
private String creatorId = null;
@Schema(description = "List of dictionaries.")
/**
* List of dictionaries.
**/
private List dictionaryRefs = null;
@Schema(description = "Recent disable time of the policy in milliseconds elapsed as of epoch time. ")
/**
* Recent disable time of the policy in milliseconds elapsed as of epoch time.
**/
private Long lastDisabledDate = null;
@Schema(description = "Recent update time of the policy in milliseconds elapsed as of epoch time.")
/**
* Recent update time of the policy in milliseconds elapsed as of epoch time.
**/
private Long lastUpdatedDate = null;
@Schema(required = true, description = "Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.")
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
**/
private String name = null;
@Schema(description = "Policy Id")
/**
* Policy Id
**/
private String policyId = null;
@Schema(required = true, description = "List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"]. ")
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
**/
private List scopes = new ArrayList();
@Schema(required = true, description = "Type of policy. Possible values \"Block\" or \"Warn\".")
/**
* Type of policy. Possible values \"Block\" or \"Warn\".
**/
private String type = null;
@Schema(description = "The version of a dictionary, in format \"major.minor\". Initial value will set by backend as \"1.0\" when created. Whenever the dictionary version needs to be changed, the minor version by 1 unless minor == 999, then the major version is increased by 1 until it reaches 999. ")
/**
* The version of a dictionary, in format \"major.minor\". Initial value will set by backend as \"1.0\" when created. Whenever the dictionary version needs to be changed, the minor version by 1 unless minor == 999, then the major version is increased by 1 until it reaches 999.
**/
private String version = null;
/**
* Indicate whether the policy is active or not
* @return active
**/
@JsonProperty("active")
public Boolean isActive() {
return active;
}
public void setActive(Boolean active) {
this.active = active;
}
public V1DLPPolicy active(Boolean active) {
this.active = active;
return this;
}
/**
* The list of content types that policy should apply to. Cannot be empty. Policy content types could be either of \"Messages\", \"RoomMeta\", \"SignalMeta\". Default is set to [\"Messages\"] if not specified.
* @return contentTypes
**/
@JsonProperty("contentTypes")
public List getContentTypes() {
return contentTypes;
}
public void setContentTypes(List contentTypes) {
this.contentTypes = contentTypes;
}
public V1DLPPolicy contentTypes(List contentTypes) {
this.contentTypes = contentTypes;
return this;
}
public V1DLPPolicy addContentTypesItem(String contentTypesItem) {
this.contentTypes.add(contentTypesItem);
return this;
}
/**
* Creation time of the policy in milliseconds elapsed as of epoch time.
* @return creationDate
**/
@JsonProperty("creationDate")
public Long getCreationDate() {
return creationDate;
}
public void setCreationDate(Long creationDate) {
this.creationDate = creationDate;
}
public V1DLPPolicy creationDate(Long creationDate) {
this.creationDate = creationDate;
return this;
}
/**
* Numeric userId of the creator
* @return creatorId
**/
@JsonProperty("creatorId")
public String getCreatorId() {
return creatorId;
}
public void setCreatorId(String creatorId) {
this.creatorId = creatorId;
}
public V1DLPPolicy creatorId(String creatorId) {
this.creatorId = creatorId;
return this;
}
/**
* List of dictionaries.
* @return dictionaryRefs
**/
@JsonProperty("dictionaryRefs")
public List getDictionaryRefs() {
return dictionaryRefs;
}
public void setDictionaryRefs(List dictionaryRefs) {
this.dictionaryRefs = dictionaryRefs;
}
public V1DLPPolicy dictionaryRefs(List dictionaryRefs) {
this.dictionaryRefs = dictionaryRefs;
return this;
}
public V1DLPPolicy addDictionaryRefsItem(V1DLPDictionaryRef dictionaryRefsItem) {
this.dictionaryRefs.add(dictionaryRefsItem);
return this;
}
/**
* Recent disable time of the policy in milliseconds elapsed as of epoch time.
* @return lastDisabledDate
**/
@JsonProperty("lastDisabledDate")
public Long getLastDisabledDate() {
return lastDisabledDate;
}
public void setLastDisabledDate(Long lastDisabledDate) {
this.lastDisabledDate = lastDisabledDate;
}
public V1DLPPolicy lastDisabledDate(Long lastDisabledDate) {
this.lastDisabledDate = lastDisabledDate;
return this;
}
/**
* Recent update time of the policy in milliseconds elapsed as of epoch time.
* @return lastUpdatedDate
**/
@JsonProperty("lastUpdatedDate")
public Long getLastUpdatedDate() {
return lastUpdatedDate;
}
public void setLastUpdatedDate(Long lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
}
public V1DLPPolicy lastUpdatedDate(Long lastUpdatedDate) {
this.lastUpdatedDate = lastUpdatedDate;
return this;
}
/**
* Unique name of a policy, max 30 characters. Cannot be empty. All the leading and trailing blank spaces are trimmed.
* @return name
**/
@JsonProperty("name")
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public V1DLPPolicy name(String name) {
this.name = name;
return this;
}
/**
* Policy Id
* @return policyId
**/
@JsonProperty("policyId")
public String getPolicyId() {
return policyId;
}
public void setPolicyId(String policyId) {
this.policyId = policyId;
}
public V1DLPPolicy policyId(String policyId) {
this.policyId = policyId;
return this;
}
/**
* List of communication scopes. Possible values are \"Internal\" (for Internal conversations) or \"External\" (for External conversations). You can apply both scopes if you set it to [\"Internal\", \"External\"].
* @return scopes
**/
@JsonProperty("scopes")
public List getScopes() {
return scopes;
}
public void setScopes(List scopes) {
this.scopes = scopes;
}
public V1DLPPolicy scopes(List scopes) {
this.scopes = scopes;
return this;
}
public V1DLPPolicy addScopesItem(String scopesItem) {
this.scopes.add(scopesItem);
return this;
}
/**
* Type of policy. Possible values \"Block\" or \"Warn\".
* @return type
**/
@JsonProperty("type")
public String getType() {
return type;
}
public void setType(String type) {
this.type = type;
}
public V1DLPPolicy type(String type) {
this.type = type;
return this;
}
/**
* The version of a dictionary, in format \"major.minor\". Initial value will set by backend as \"1.0\" when created. Whenever the dictionary version needs to be changed, the minor version by 1 unless minor == 999, then the major version is increased by 1 until it reaches 999.
* @return version
**/
@JsonProperty("version")
public String getVersion() {
return version;
}
public void setVersion(String version) {
this.version = version;
}
public V1DLPPolicy version(String version) {
this.version = version;
return this;
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class V1DLPPolicy {\n");
sb.append(" active: ").append(toIndentedString(active)).append("\n");
sb.append(" contentTypes: ").append(toIndentedString(contentTypes)).append("\n");
sb.append(" creationDate: ").append(toIndentedString(creationDate)).append("\n");
sb.append(" creatorId: ").append(toIndentedString(creatorId)).append("\n");
sb.append(" dictionaryRefs: ").append(toIndentedString(dictionaryRefs)).append("\n");
sb.append(" lastDisabledDate: ").append(toIndentedString(lastDisabledDate)).append("\n");
sb.append(" lastUpdatedDate: ").append(toIndentedString(lastUpdatedDate)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" policyId: ").append(toIndentedString(policyId)).append("\n");
sb.append(" scopes: ").append(toIndentedString(scopes)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private static String toIndentedString(java.lang.Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy